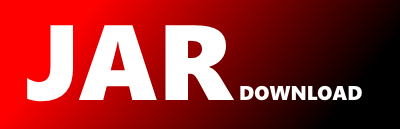
com.pulumi.azurenative.sqlvirtualmachine.inputs.WsfcDomainProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sqlvirtualmachine.inputs;
import com.pulumi.azurenative.sqlvirtualmachine.enums.ClusterSubnetType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Active Directory account details to operate Windows Server Failover Cluster.
*
*/
public final class WsfcDomainProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final WsfcDomainProfileArgs Empty = new WsfcDomainProfileArgs();
/**
* Account name used for creating cluster (at minimum needs permissions to 'Create Computer Objects' in domain).
*
*/
@Import(name="clusterBootstrapAccount")
private @Nullable Output clusterBootstrapAccount;
/**
* @return Account name used for creating cluster (at minimum needs permissions to 'Create Computer Objects' in domain).
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy