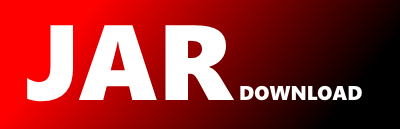
com.pulumi.azurenative.storage.FileServicePropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.azurenative.storage.inputs.CorsRulesArgs;
import com.pulumi.azurenative.storage.inputs.DeleteRetentionPolicyArgs;
import com.pulumi.azurenative.storage.inputs.ProtocolSettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FileServicePropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final FileServicePropertiesArgs Empty = new FileServicePropertiesArgs();
/**
* The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
*
*/
public Output accountName() {
return this.accountName;
}
/**
* Specifies CORS rules for the File service. You can include up to five CorsRule elements in the request. If no CorsRule elements are included in the request body, all CORS rules will be deleted, and CORS will be disabled for the File service.
*
*/
@Import(name="cors")
private @Nullable Output cors;
/**
* @return Specifies CORS rules for the File service. You can include up to five CorsRule elements in the request. If no CorsRule elements are included in the request body, all CORS rules will be deleted, and CORS will be disabled for the File service.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy