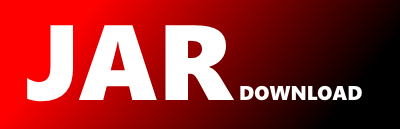
com.pulumi.azurenative.storage.ObjectReplicationPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.azurenative.storage.inputs.ObjectReplicationPolicyRuleArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ObjectReplicationPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final ObjectReplicationPolicyArgs Empty = new ObjectReplicationPolicyArgs();
/**
* The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
*
*/
public Output accountName() {
return this.accountName;
}
/**
* Required. Destination account name. It should be full resource id if allowCrossTenantReplication set to false.
*
*/
@Import(name="destinationAccount", required=true)
private Output destinationAccount;
/**
* @return Required. Destination account name. It should be full resource id if allowCrossTenantReplication set to false.
*
*/
public Output destinationAccount() {
return this.destinationAccount;
}
/**
* For the destination account, provide the value 'default'. Configure the policy on the destination account first. For the source account, provide the value of the policy ID that is returned when you download the policy that was defined on the destination account. The policy is downloaded as a JSON file.
*
*/
@Import(name="objectReplicationPolicyId")
private @Nullable Output objectReplicationPolicyId;
/**
* @return For the destination account, provide the value 'default'. Configure the policy on the destination account first. For the source account, provide the value of the policy ID that is returned when you download the policy that was defined on the destination account. The policy is downloaded as a JSON file.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy