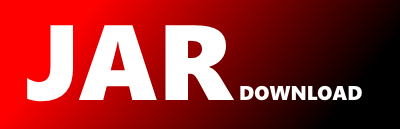
com.pulumi.azurenative.storage.StorageFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.storage.inputs.GetBlobContainerArgs;
import com.pulumi.azurenative.storage.inputs.GetBlobContainerImmutabilityPolicyArgs;
import com.pulumi.azurenative.storage.inputs.GetBlobContainerImmutabilityPolicyPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetBlobContainerPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetBlobInventoryPolicyArgs;
import com.pulumi.azurenative.storage.inputs.GetBlobInventoryPolicyPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetBlobServicePropertiesArgs;
import com.pulumi.azurenative.storage.inputs.GetBlobServicePropertiesPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetEncryptionScopeArgs;
import com.pulumi.azurenative.storage.inputs.GetEncryptionScopePlainArgs;
import com.pulumi.azurenative.storage.inputs.GetFileServicePropertiesArgs;
import com.pulumi.azurenative.storage.inputs.GetFileServicePropertiesPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetFileShareArgs;
import com.pulumi.azurenative.storage.inputs.GetFileSharePlainArgs;
import com.pulumi.azurenative.storage.inputs.GetLocalUserArgs;
import com.pulumi.azurenative.storage.inputs.GetLocalUserPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetManagementPolicyArgs;
import com.pulumi.azurenative.storage.inputs.GetManagementPolicyPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetObjectReplicationPolicyArgs;
import com.pulumi.azurenative.storage.inputs.GetObjectReplicationPolicyPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.storage.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetQueueArgs;
import com.pulumi.azurenative.storage.inputs.GetQueuePlainArgs;
import com.pulumi.azurenative.storage.inputs.GetQueueServicePropertiesArgs;
import com.pulumi.azurenative.storage.inputs.GetQueueServicePropertiesPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetStorageAccountArgs;
import com.pulumi.azurenative.storage.inputs.GetStorageAccountPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetStorageTaskAssignmentArgs;
import com.pulumi.azurenative.storage.inputs.GetStorageTaskAssignmentPlainArgs;
import com.pulumi.azurenative.storage.inputs.GetTableArgs;
import com.pulumi.azurenative.storage.inputs.GetTablePlainArgs;
import com.pulumi.azurenative.storage.inputs.GetTableServicePropertiesArgs;
import com.pulumi.azurenative.storage.inputs.GetTableServicePropertiesPlainArgs;
import com.pulumi.azurenative.storage.inputs.ListLocalUserKeysArgs;
import com.pulumi.azurenative.storage.inputs.ListLocalUserKeysPlainArgs;
import com.pulumi.azurenative.storage.inputs.ListStorageAccountKeysArgs;
import com.pulumi.azurenative.storage.inputs.ListStorageAccountKeysPlainArgs;
import com.pulumi.azurenative.storage.inputs.ListStorageAccountSASArgs;
import com.pulumi.azurenative.storage.inputs.ListStorageAccountSASPlainArgs;
import com.pulumi.azurenative.storage.inputs.ListStorageAccountServiceSASArgs;
import com.pulumi.azurenative.storage.inputs.ListStorageAccountServiceSASPlainArgs;
import com.pulumi.azurenative.storage.outputs.GetBlobContainerImmutabilityPolicyResult;
import com.pulumi.azurenative.storage.outputs.GetBlobContainerResult;
import com.pulumi.azurenative.storage.outputs.GetBlobInventoryPolicyResult;
import com.pulumi.azurenative.storage.outputs.GetBlobServicePropertiesResult;
import com.pulumi.azurenative.storage.outputs.GetEncryptionScopeResult;
import com.pulumi.azurenative.storage.outputs.GetFileServicePropertiesResult;
import com.pulumi.azurenative.storage.outputs.GetFileShareResult;
import com.pulumi.azurenative.storage.outputs.GetLocalUserResult;
import com.pulumi.azurenative.storage.outputs.GetManagementPolicyResult;
import com.pulumi.azurenative.storage.outputs.GetObjectReplicationPolicyResult;
import com.pulumi.azurenative.storage.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.storage.outputs.GetQueueResult;
import com.pulumi.azurenative.storage.outputs.GetQueueServicePropertiesResult;
import com.pulumi.azurenative.storage.outputs.GetStorageAccountResult;
import com.pulumi.azurenative.storage.outputs.GetStorageTaskAssignmentResult;
import com.pulumi.azurenative.storage.outputs.GetTableResult;
import com.pulumi.azurenative.storage.outputs.GetTableServicePropertiesResult;
import com.pulumi.azurenative.storage.outputs.ListLocalUserKeysResult;
import com.pulumi.azurenative.storage.outputs.ListStorageAccountKeysResult;
import com.pulumi.azurenative.storage.outputs.ListStorageAccountSASResult;
import com.pulumi.azurenative.storage.outputs.ListStorageAccountServiceSASResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class StorageFunctions {
/**
* Gets properties of a specified container.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobContainer(GetBlobContainerArgs args) {
return getBlobContainer(args, InvokeOptions.Empty);
}
/**
* Gets properties of a specified container.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobContainerPlain(GetBlobContainerPlainArgs args) {
return getBlobContainerPlain(args, InvokeOptions.Empty);
}
/**
* Gets properties of a specified container.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobContainer(GetBlobContainerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getBlobContainer", TypeShape.of(GetBlobContainerResult.class), args, Utilities.withVersion(options));
}
/**
* Gets properties of a specified container.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobContainerPlain(GetBlobContainerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getBlobContainer", TypeShape.of(GetBlobContainerResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2019-04-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobContainerImmutabilityPolicy(GetBlobContainerImmutabilityPolicyArgs args) {
return getBlobContainerImmutabilityPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2019-04-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobContainerImmutabilityPolicyPlain(GetBlobContainerImmutabilityPolicyPlainArgs args) {
return getBlobContainerImmutabilityPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2019-04-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobContainerImmutabilityPolicy(GetBlobContainerImmutabilityPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getBlobContainerImmutabilityPolicy", TypeShape.of(GetBlobContainerImmutabilityPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2019-04-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobContainerImmutabilityPolicyPlain(GetBlobContainerImmutabilityPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getBlobContainerImmutabilityPolicy", TypeShape.of(GetBlobContainerImmutabilityPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the blob inventory policy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobInventoryPolicy(GetBlobInventoryPolicyArgs args) {
return getBlobInventoryPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the blob inventory policy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobInventoryPolicyPlain(GetBlobInventoryPolicyPlainArgs args) {
return getBlobInventoryPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the blob inventory policy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobInventoryPolicy(GetBlobInventoryPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getBlobInventoryPolicy", TypeShape.of(GetBlobInventoryPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the blob inventory policy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobInventoryPolicyPlain(GetBlobInventoryPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getBlobInventoryPolicy", TypeShape.of(GetBlobInventoryPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of a storage account’s Blob service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobServiceProperties(GetBlobServicePropertiesArgs args) {
return getBlobServiceProperties(args, InvokeOptions.Empty);
}
/**
* Gets the properties of a storage account’s Blob service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobServicePropertiesPlain(GetBlobServicePropertiesPlainArgs args) {
return getBlobServicePropertiesPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of a storage account’s Blob service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getBlobServiceProperties(GetBlobServicePropertiesArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getBlobServiceProperties", TypeShape.of(GetBlobServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of a storage account’s Blob service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getBlobServicePropertiesPlain(GetBlobServicePropertiesPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getBlobServiceProperties", TypeShape.of(GetBlobServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties for the specified encryption scope.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getEncryptionScope(GetEncryptionScopeArgs args) {
return getEncryptionScope(args, InvokeOptions.Empty);
}
/**
* Returns the properties for the specified encryption scope.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getEncryptionScopePlain(GetEncryptionScopePlainArgs args) {
return getEncryptionScopePlain(args, InvokeOptions.Empty);
}
/**
* Returns the properties for the specified encryption scope.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getEncryptionScope(GetEncryptionScopeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getEncryptionScope", TypeShape.of(GetEncryptionScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties for the specified encryption scope.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getEncryptionScopePlain(GetEncryptionScopePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getEncryptionScope", TypeShape.of(GetEncryptionScopeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of file services in storage accounts, including CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getFileServiceProperties(GetFileServicePropertiesArgs args) {
return getFileServiceProperties(args, InvokeOptions.Empty);
}
/**
* Gets the properties of file services in storage accounts, including CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getFileServicePropertiesPlain(GetFileServicePropertiesPlainArgs args) {
return getFileServicePropertiesPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of file services in storage accounts, including CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getFileServiceProperties(GetFileServicePropertiesArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getFileServiceProperties", TypeShape.of(GetFileServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of file services in storage accounts, including CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getFileServicePropertiesPlain(GetFileServicePropertiesPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getFileServiceProperties", TypeShape.of(GetFileServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* Gets properties of a specified share.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getFileShare(GetFileShareArgs args) {
return getFileShare(args, InvokeOptions.Empty);
}
/**
* Gets properties of a specified share.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getFileSharePlain(GetFileSharePlainArgs args) {
return getFileSharePlain(args, InvokeOptions.Empty);
}
/**
* Gets properties of a specified share.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getFileShare(GetFileShareArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getFileShare", TypeShape.of(GetFileShareResult.class), args, Utilities.withVersion(options));
}
/**
* Gets properties of a specified share.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getFileSharePlain(GetFileSharePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getFileShare", TypeShape.of(GetFileShareResult.class), args, Utilities.withVersion(options));
}
/**
* Get the local user of the storage account by username.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getLocalUser(GetLocalUserArgs args) {
return getLocalUser(args, InvokeOptions.Empty);
}
/**
* Get the local user of the storage account by username.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getLocalUserPlain(GetLocalUserPlainArgs args) {
return getLocalUserPlain(args, InvokeOptions.Empty);
}
/**
* Get the local user of the storage account by username.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getLocalUser(GetLocalUserArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getLocalUser", TypeShape.of(GetLocalUserResult.class), args, Utilities.withVersion(options));
}
/**
* Get the local user of the storage account by username.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getLocalUserPlain(GetLocalUserPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getLocalUser", TypeShape.of(GetLocalUserResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the managementpolicy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-03-01-preview, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getManagementPolicy(GetManagementPolicyArgs args) {
return getManagementPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the managementpolicy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-03-01-preview, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getManagementPolicyPlain(GetManagementPolicyPlainArgs args) {
return getManagementPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the managementpolicy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-03-01-preview, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getManagementPolicy(GetManagementPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getManagementPolicy", TypeShape.of(GetManagementPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the managementpolicy associated with the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-03-01-preview, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getManagementPolicyPlain(GetManagementPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getManagementPolicy", TypeShape.of(GetManagementPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Get the object replication policy of the storage account by policy ID.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getObjectReplicationPolicy(GetObjectReplicationPolicyArgs args) {
return getObjectReplicationPolicy(args, InvokeOptions.Empty);
}
/**
* Get the object replication policy of the storage account by policy ID.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getObjectReplicationPolicyPlain(GetObjectReplicationPolicyPlainArgs args) {
return getObjectReplicationPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Get the object replication policy of the storage account by policy ID.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getObjectReplicationPolicy(GetObjectReplicationPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getObjectReplicationPolicy", TypeShape.of(GetObjectReplicationPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Get the object replication policy of the storage account by policy ID.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getObjectReplicationPolicyPlain(GetObjectReplicationPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getObjectReplicationPolicy", TypeShape.of(GetObjectReplicationPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the queue with the specified queue name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getQueue(GetQueueArgs args) {
return getQueue(args, InvokeOptions.Empty);
}
/**
* Gets the queue with the specified queue name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getQueuePlain(GetQueuePlainArgs args) {
return getQueuePlain(args, InvokeOptions.Empty);
}
/**
* Gets the queue with the specified queue name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getQueue(GetQueueArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getQueue", TypeShape.of(GetQueueResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the queue with the specified queue name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getQueuePlain(GetQueuePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getQueue", TypeShape.of(GetQueueResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of a storage account’s Queue service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getQueueServiceProperties(GetQueueServicePropertiesArgs args) {
return getQueueServiceProperties(args, InvokeOptions.Empty);
}
/**
* Gets the properties of a storage account’s Queue service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getQueueServicePropertiesPlain(GetQueueServicePropertiesPlainArgs args) {
return getQueueServicePropertiesPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of a storage account’s Queue service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getQueueServiceProperties(GetQueueServicePropertiesArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getQueueServiceProperties", TypeShape.of(GetQueueServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of a storage account’s Queue service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getQueueServicePropertiesPlain(GetQueueServicePropertiesPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getQueueServiceProperties", TypeShape.of(GetQueueServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location, and account status. The ListKeys operation should be used to retrieve storage keys.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getStorageAccount(GetStorageAccountArgs args) {
return getStorageAccount(args, InvokeOptions.Empty);
}
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location, and account status. The ListKeys operation should be used to retrieve storage keys.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getStorageAccountPlain(GetStorageAccountPlainArgs args) {
return getStorageAccountPlain(args, InvokeOptions.Empty);
}
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location, and account status. The ListKeys operation should be used to retrieve storage keys.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getStorageAccount(GetStorageAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getStorageAccount", TypeShape.of(GetStorageAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location, and account status. The ListKeys operation should be used to retrieve storage keys.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getStorageAccountPlain(GetStorageAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getStorageAccount", TypeShape.of(GetStorageAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Get the storage task assignment properties
* Azure REST API version: 2023-05-01.
*
*/
public static Output getStorageTaskAssignment(GetStorageTaskAssignmentArgs args) {
return getStorageTaskAssignment(args, InvokeOptions.Empty);
}
/**
* Get the storage task assignment properties
* Azure REST API version: 2023-05-01.
*
*/
public static CompletableFuture getStorageTaskAssignmentPlain(GetStorageTaskAssignmentPlainArgs args) {
return getStorageTaskAssignmentPlain(args, InvokeOptions.Empty);
}
/**
* Get the storage task assignment properties
* Azure REST API version: 2023-05-01.
*
*/
public static Output getStorageTaskAssignment(GetStorageTaskAssignmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getStorageTaskAssignment", TypeShape.of(GetStorageTaskAssignmentResult.class), args, Utilities.withVersion(options));
}
/**
* Get the storage task assignment properties
* Azure REST API version: 2023-05-01.
*
*/
public static CompletableFuture getStorageTaskAssignmentPlain(GetStorageTaskAssignmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getStorageTaskAssignment", TypeShape.of(GetStorageTaskAssignmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the table with the specified table name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getTable(GetTableArgs args) {
return getTable(args, InvokeOptions.Empty);
}
/**
* Gets the table with the specified table name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getTablePlain(GetTablePlainArgs args) {
return getTablePlain(args, InvokeOptions.Empty);
}
/**
* Gets the table with the specified table name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getTable(GetTableArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getTable", TypeShape.of(GetTableResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the table with the specified table name, under the specified account if it exists.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getTablePlain(GetTablePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getTable", TypeShape.of(GetTableResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of a storage account’s Table service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getTableServiceProperties(GetTableServicePropertiesArgs args) {
return getTableServiceProperties(args, InvokeOptions.Empty);
}
/**
* Gets the properties of a storage account’s Table service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getTableServicePropertiesPlain(GetTableServicePropertiesPlainArgs args) {
return getTableServicePropertiesPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of a storage account’s Table service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output getTableServiceProperties(GetTableServicePropertiesArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:getTableServiceProperties", TypeShape.of(GetTableServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of a storage account’s Table service, including properties for Storage Analytics and CORS (Cross-Origin Resource Sharing) rules.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture getTableServicePropertiesPlain(GetTableServicePropertiesPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:getTableServiceProperties", TypeShape.of(GetTableServicePropertiesResult.class), args, Utilities.withVersion(options));
}
/**
* List SSH authorized keys and shared key of the local user.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listLocalUserKeys(ListLocalUserKeysArgs args) {
return listLocalUserKeys(args, InvokeOptions.Empty);
}
/**
* List SSH authorized keys and shared key of the local user.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listLocalUserKeysPlain(ListLocalUserKeysPlainArgs args) {
return listLocalUserKeysPlain(args, InvokeOptions.Empty);
}
/**
* List SSH authorized keys and shared key of the local user.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listLocalUserKeys(ListLocalUserKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:listLocalUserKeys", TypeShape.of(ListLocalUserKeysResult.class), args, Utilities.withVersion(options));
}
/**
* List SSH authorized keys and shared key of the local user.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listLocalUserKeysPlain(ListLocalUserKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:listLocalUserKeys", TypeShape.of(ListLocalUserKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listStorageAccountKeys(ListStorageAccountKeysArgs args) {
return listStorageAccountKeys(args, InvokeOptions.Empty);
}
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listStorageAccountKeysPlain(ListStorageAccountKeysPlainArgs args) {
return listStorageAccountKeysPlain(args, InvokeOptions.Empty);
}
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listStorageAccountKeys(ListStorageAccountKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:listStorageAccountKeys", TypeShape.of(ListStorageAccountKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2015-05-01-preview, 2015-06-15, 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listStorageAccountKeysPlain(ListStorageAccountKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:listStorageAccountKeys", TypeShape.of(ListStorageAccountKeysResult.class), args, Utilities.withVersion(options));
}
/**
* List SAS credentials of a storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listStorageAccountSAS(ListStorageAccountSASArgs args) {
return listStorageAccountSAS(args, InvokeOptions.Empty);
}
/**
* List SAS credentials of a storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listStorageAccountSASPlain(ListStorageAccountSASPlainArgs args) {
return listStorageAccountSASPlain(args, InvokeOptions.Empty);
}
/**
* List SAS credentials of a storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listStorageAccountSAS(ListStorageAccountSASArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:listStorageAccountSAS", TypeShape.of(ListStorageAccountSASResult.class), args, Utilities.withVersion(options));
}
/**
* List SAS credentials of a storage account.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listStorageAccountSASPlain(ListStorageAccountSASPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:listStorageAccountSAS", TypeShape.of(ListStorageAccountSASResult.class), args, Utilities.withVersion(options));
}
/**
* List service SAS credentials of a specific resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listStorageAccountServiceSAS(ListStorageAccountServiceSASArgs args) {
return listStorageAccountServiceSAS(args, InvokeOptions.Empty);
}
/**
* List service SAS credentials of a specific resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listStorageAccountServiceSASPlain(ListStorageAccountServiceSASPlainArgs args) {
return listStorageAccountServiceSASPlain(args, InvokeOptions.Empty);
}
/**
* List service SAS credentials of a specific resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static Output listStorageAccountServiceSAS(ListStorageAccountServiceSASArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:storage:listStorageAccountServiceSAS", TypeShape.of(ListStorageAccountServiceSASResult.class), args, Utilities.withVersion(options));
}
/**
* List service SAS credentials of a specific resource.
* Azure REST API version: 2022-09-01.
*
* Other available API versions: 2018-11-01, 2023-01-01, 2023-04-01, 2023-05-01.
*
*/
public static CompletableFuture listStorageAccountServiceSASPlain(ListStorageAccountServiceSASPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:storage:listStorageAccountServiceSAS", TypeShape.of(ListStorageAccountServiceSASResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy