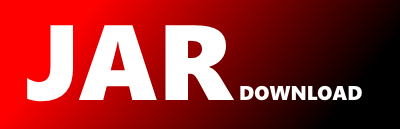
com.pulumi.azurenative.storage.inputs.DateAfterModificationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Object to define the base blob action conditions. Properties daysAfterModificationGreaterThan, daysAfterLastAccessTimeGreaterThan and daysAfterCreationGreaterThan are mutually exclusive. The daysAfterLastTierChangeGreaterThan property is only applicable for tierToArchive actions which requires daysAfterModificationGreaterThan to be set, also it cannot be used in conjunction with daysAfterLastAccessTimeGreaterThan or daysAfterCreationGreaterThan.
*
*/
public final class DateAfterModificationArgs extends com.pulumi.resources.ResourceArgs {
public static final DateAfterModificationArgs Empty = new DateAfterModificationArgs();
/**
* Value indicating the age in days after blob creation.
*
*/
@Import(name="daysAfterCreationGreaterThan")
private @Nullable Output daysAfterCreationGreaterThan;
/**
* @return Value indicating the age in days after blob creation.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy