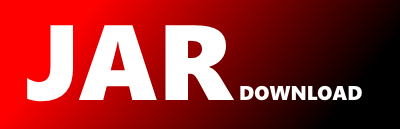
com.pulumi.azurenative.storagecache.outputs.GetCacheResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagecache.outputs;
import com.pulumi.azurenative.storagecache.outputs.CacheDirectorySettingsResponse;
import com.pulumi.azurenative.storagecache.outputs.CacheEncryptionSettingsResponse;
import com.pulumi.azurenative.storagecache.outputs.CacheHealthResponse;
import com.pulumi.azurenative.storagecache.outputs.CacheIdentityResponse;
import com.pulumi.azurenative.storagecache.outputs.CacheNetworkSettingsResponse;
import com.pulumi.azurenative.storagecache.outputs.CacheResponseSku;
import com.pulumi.azurenative.storagecache.outputs.CacheSecuritySettingsResponse;
import com.pulumi.azurenative.storagecache.outputs.CacheUpgradeSettingsResponse;
import com.pulumi.azurenative.storagecache.outputs.CacheUpgradeStatusResponse;
import com.pulumi.azurenative.storagecache.outputs.PrimingJobResponse;
import com.pulumi.azurenative.storagecache.outputs.StorageTargetSpaceAllocationResponse;
import com.pulumi.azurenative.storagecache.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetCacheResult {
/**
* @return The size of this Cache, in GB.
*
*/
private @Nullable Integer cacheSizeGB;
/**
* @return Specifies Directory Services settings of the cache.
*
*/
private @Nullable CacheDirectorySettingsResponse directoryServicesSettings;
/**
* @return Specifies encryption settings of the cache.
*
*/
private @Nullable CacheEncryptionSettingsResponse encryptionSettings;
/**
* @return Health of the cache.
*
*/
private CacheHealthResponse health;
/**
* @return Resource ID of the cache.
*
*/
private String id;
/**
* @return The identity of the cache, if configured.
*
*/
private @Nullable CacheIdentityResponse identity;
/**
* @return Region name string.
*
*/
private @Nullable String location;
/**
* @return Array of IPv4 addresses that can be used by clients mounting this cache.
*
*/
private List mountAddresses;
/**
* @return Name of cache.
*
*/
private String name;
/**
* @return Specifies network settings of the cache.
*
*/
private @Nullable CacheNetworkSettingsResponse networkSettings;
/**
* @return Specifies the priming jobs defined in the cache.
*
*/
private List primingJobs;
/**
* @return ARM provisioning state, see https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/Addendum.md#provisioningstate-property
*
*/
private String provisioningState;
/**
* @return Specifies security settings of the cache.
*
*/
private @Nullable CacheSecuritySettingsResponse securitySettings;
/**
* @return SKU for the cache.
*
*/
private @Nullable CacheResponseSku sku;
/**
* @return Specifies the space allocation percentage for each storage target in the cache.
*
*/
private List spaceAllocation;
/**
* @return Subnet used for the cache.
*
*/
private @Nullable String subnet;
/**
* @return The system meta data relating to this resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Type of the cache; Microsoft.StorageCache/Cache
*
*/
private String type;
/**
* @return Upgrade settings of the cache.
*
*/
private @Nullable CacheUpgradeSettingsResponse upgradeSettings;
/**
* @return Upgrade status of the cache.
*
*/
private CacheUpgradeStatusResponse upgradeStatus;
/**
* @return Availability zones for resources. This field should only contain a single element in the array.
*
*/
private @Nullable List zones;
private GetCacheResult() {}
/**
* @return The size of this Cache, in GB.
*
*/
public Optional cacheSizeGB() {
return Optional.ofNullable(this.cacheSizeGB);
}
/**
* @return Specifies Directory Services settings of the cache.
*
*/
public Optional directoryServicesSettings() {
return Optional.ofNullable(this.directoryServicesSettings);
}
/**
* @return Specifies encryption settings of the cache.
*
*/
public Optional encryptionSettings() {
return Optional.ofNullable(this.encryptionSettings);
}
/**
* @return Health of the cache.
*
*/
public CacheHealthResponse health() {
return this.health;
}
/**
* @return Resource ID of the cache.
*
*/
public String id() {
return this.id;
}
/**
* @return The identity of the cache, if configured.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return Region name string.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Array of IPv4 addresses that can be used by clients mounting this cache.
*
*/
public List mountAddresses() {
return this.mountAddresses;
}
/**
* @return Name of cache.
*
*/
public String name() {
return this.name;
}
/**
* @return Specifies network settings of the cache.
*
*/
public Optional networkSettings() {
return Optional.ofNullable(this.networkSettings);
}
/**
* @return Specifies the priming jobs defined in the cache.
*
*/
public List primingJobs() {
return this.primingJobs;
}
/**
* @return ARM provisioning state, see https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/Addendum.md#provisioningstate-property
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Specifies security settings of the cache.
*
*/
public Optional securitySettings() {
return Optional.ofNullable(this.securitySettings);
}
/**
* @return SKU for the cache.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Specifies the space allocation percentage for each storage target in the cache.
*
*/
public List spaceAllocation() {
return this.spaceAllocation;
}
/**
* @return Subnet used for the cache.
*
*/
public Optional subnet() {
return Optional.ofNullable(this.subnet);
}
/**
* @return The system meta data relating to this resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Type of the cache; Microsoft.StorageCache/Cache
*
*/
public String type() {
return this.type;
}
/**
* @return Upgrade settings of the cache.
*
*/
public Optional upgradeSettings() {
return Optional.ofNullable(this.upgradeSettings);
}
/**
* @return Upgrade status of the cache.
*
*/
public CacheUpgradeStatusResponse upgradeStatus() {
return this.upgradeStatus;
}
/**
* @return Availability zones for resources. This field should only contain a single element in the array.
*
*/
public List zones() {
return this.zones == null ? List.of() : this.zones;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCacheResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer cacheSizeGB;
private @Nullable CacheDirectorySettingsResponse directoryServicesSettings;
private @Nullable CacheEncryptionSettingsResponse encryptionSettings;
private CacheHealthResponse health;
private String id;
private @Nullable CacheIdentityResponse identity;
private @Nullable String location;
private List mountAddresses;
private String name;
private @Nullable CacheNetworkSettingsResponse networkSettings;
private List primingJobs;
private String provisioningState;
private @Nullable CacheSecuritySettingsResponse securitySettings;
private @Nullable CacheResponseSku sku;
private List spaceAllocation;
private @Nullable String subnet;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
private @Nullable CacheUpgradeSettingsResponse upgradeSettings;
private CacheUpgradeStatusResponse upgradeStatus;
private @Nullable List zones;
public Builder() {}
public Builder(GetCacheResult defaults) {
Objects.requireNonNull(defaults);
this.cacheSizeGB = defaults.cacheSizeGB;
this.directoryServicesSettings = defaults.directoryServicesSettings;
this.encryptionSettings = defaults.encryptionSettings;
this.health = defaults.health;
this.id = defaults.id;
this.identity = defaults.identity;
this.location = defaults.location;
this.mountAddresses = defaults.mountAddresses;
this.name = defaults.name;
this.networkSettings = defaults.networkSettings;
this.primingJobs = defaults.primingJobs;
this.provisioningState = defaults.provisioningState;
this.securitySettings = defaults.securitySettings;
this.sku = defaults.sku;
this.spaceAllocation = defaults.spaceAllocation;
this.subnet = defaults.subnet;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
this.upgradeSettings = defaults.upgradeSettings;
this.upgradeStatus = defaults.upgradeStatus;
this.zones = defaults.zones;
}
@CustomType.Setter
public Builder cacheSizeGB(@Nullable Integer cacheSizeGB) {
this.cacheSizeGB = cacheSizeGB;
return this;
}
@CustomType.Setter
public Builder directoryServicesSettings(@Nullable CacheDirectorySettingsResponse directoryServicesSettings) {
this.directoryServicesSettings = directoryServicesSettings;
return this;
}
@CustomType.Setter
public Builder encryptionSettings(@Nullable CacheEncryptionSettingsResponse encryptionSettings) {
this.encryptionSettings = encryptionSettings;
return this;
}
@CustomType.Setter
public Builder health(CacheHealthResponse health) {
if (health == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "health");
}
this.health = health;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable CacheIdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder mountAddresses(List mountAddresses) {
if (mountAddresses == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "mountAddresses");
}
this.mountAddresses = mountAddresses;
return this;
}
public Builder mountAddresses(String... mountAddresses) {
return mountAddresses(List.of(mountAddresses));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkSettings(@Nullable CacheNetworkSettingsResponse networkSettings) {
this.networkSettings = networkSettings;
return this;
}
@CustomType.Setter
public Builder primingJobs(List primingJobs) {
if (primingJobs == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "primingJobs");
}
this.primingJobs = primingJobs;
return this;
}
public Builder primingJobs(PrimingJobResponse... primingJobs) {
return primingJobs(List.of(primingJobs));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder securitySettings(@Nullable CacheSecuritySettingsResponse securitySettings) {
this.securitySettings = securitySettings;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable CacheResponseSku sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder spaceAllocation(List spaceAllocation) {
if (spaceAllocation == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "spaceAllocation");
}
this.spaceAllocation = spaceAllocation;
return this;
}
public Builder spaceAllocation(StorageTargetSpaceAllocationResponse... spaceAllocation) {
return spaceAllocation(List.of(spaceAllocation));
}
@CustomType.Setter
public Builder subnet(@Nullable String subnet) {
this.subnet = subnet;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder upgradeSettings(@Nullable CacheUpgradeSettingsResponse upgradeSettings) {
this.upgradeSettings = upgradeSettings;
return this;
}
@CustomType.Setter
public Builder upgradeStatus(CacheUpgradeStatusResponse upgradeStatus) {
if (upgradeStatus == null) {
throw new MissingRequiredPropertyException("GetCacheResult", "upgradeStatus");
}
this.upgradeStatus = upgradeStatus;
return this;
}
@CustomType.Setter
public Builder zones(@Nullable List zones) {
this.zones = zones;
return this;
}
public Builder zones(String... zones) {
return zones(List.of(zones));
}
public GetCacheResult build() {
final var _resultValue = new GetCacheResult();
_resultValue.cacheSizeGB = cacheSizeGB;
_resultValue.directoryServicesSettings = directoryServicesSettings;
_resultValue.encryptionSettings = encryptionSettings;
_resultValue.health = health;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.location = location;
_resultValue.mountAddresses = mountAddresses;
_resultValue.name = name;
_resultValue.networkSettings = networkSettings;
_resultValue.primingJobs = primingJobs;
_resultValue.provisioningState = provisioningState;
_resultValue.securitySettings = securitySettings;
_resultValue.sku = sku;
_resultValue.spaceAllocation = spaceAllocation;
_resultValue.subnet = subnet;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.upgradeSettings = upgradeSettings;
_resultValue.upgradeStatus = upgradeStatus;
_resultValue.zones = zones;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy