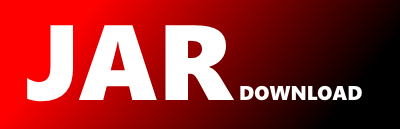
com.pulumi.azurenative.streamanalytics.StreamingJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.streamanalytics.StreamingJobArgs;
import com.pulumi.azurenative.streamanalytics.outputs.ClusterInfoResponse;
import com.pulumi.azurenative.streamanalytics.outputs.FunctionResponse;
import com.pulumi.azurenative.streamanalytics.outputs.IdentityResponse;
import com.pulumi.azurenative.streamanalytics.outputs.InputResponse;
import com.pulumi.azurenative.streamanalytics.outputs.JobStorageAccountResponse;
import com.pulumi.azurenative.streamanalytics.outputs.OutputResponse;
import com.pulumi.azurenative.streamanalytics.outputs.SkuResponse;
import com.pulumi.azurenative.streamanalytics.outputs.TransformationResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A streaming job object, containing all information associated with the named streaming job.
* Azure REST API version: 2020-03-01. Prior API version in Azure Native 1.x: 2016-03-01.
*
* Other available API versions: 2017-04-01-preview, 2021-10-01-preview.
*
* ## Example Usage
* ### Create a complete streaming job (a streaming job with a transformation, at least 1 input and at least 1 output)
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.streamanalytics.StreamingJob;
* import com.pulumi.azurenative.streamanalytics.StreamingJobArgs;
* import com.pulumi.azurenative.streamanalytics.inputs.InputArgs;
* import com.pulumi.azurenative.streamanalytics.inputs.OutputArgs;
* import com.pulumi.azurenative.streamanalytics.inputs.SkuArgs;
* import com.pulumi.azurenative.streamanalytics.inputs.TransformationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var streamingJob = new StreamingJob("streamingJob", StreamingJobArgs.builder()
* .compatibilityLevel("1.0")
* .dataLocale("en-US")
* .eventsLateArrivalMaxDelayInSeconds(5)
* .eventsOutOfOrderMaxDelayInSeconds(0)
* .eventsOutOfOrderPolicy("Drop")
* .functions()
* .inputs(InputArgs.builder()
* .name("inputtest")
* .properties(StreamInputPropertiesArgs.builder()
* .datasource(BlobStreamInputDataSourceArgs.builder()
* .container("containerName")
* .pathPattern("")
* .storageAccounts(StorageAccountArgs.builder()
* .accountKey("yourAccountKey==")
* .accountName("yourAccountName")
* .build())
* .type("Microsoft.Storage/Blob")
* .build())
* .serialization(JsonSerializationArgs.builder()
* .encoding("UTF8")
* .type("Json")
* .build())
* .type("Stream")
* .build())
* .build())
* .jobName("sj7804")
* .location("West US")
* .outputErrorPolicy("Drop")
* .outputs(OutputArgs.builder()
* .datasource(AzureDataLakeStoreOutputDataSourceArgs.builder()
* .database("databaseName")
* .password("userPassword")
* .server("serverName")
* .table("tableName")
* .type("Microsoft.Sql/Server/Database")
* .user("")
* .build())
* .name("outputtest")
* .build())
* .resourceGroupName("sjrg3276")
* .sku(SkuArgs.builder()
* .name("Standard")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key3", "value3"),
* Map.entry("randomKey", "randomValue")
* ))
* .transformation(TransformationArgs.builder()
* .name("transformationtest")
* .query("Select Id, Name from inputtest")
* .streamingUnits(1)
* .build())
* .build());
*
* }
* }
*
* }
*
* ### Create a streaming job shell (a streaming job with no inputs, outputs, transformation, or functions)
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.streamanalytics.StreamingJob;
* import com.pulumi.azurenative.streamanalytics.StreamingJobArgs;
* import com.pulumi.azurenative.streamanalytics.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var streamingJob = new StreamingJob("streamingJob", StreamingJobArgs.builder()
* .compatibilityLevel("1.0")
* .dataLocale("en-US")
* .eventsLateArrivalMaxDelayInSeconds(16)
* .eventsOutOfOrderMaxDelayInSeconds(5)
* .eventsOutOfOrderPolicy("Drop")
* .functions()
* .inputs()
* .jobName("sj59")
* .location("West US")
* .outputErrorPolicy("Drop")
* .outputs()
* .resourceGroupName("sjrg6936")
* .sku(SkuArgs.builder()
* .name("Standard")
* .build())
* .tags(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key3", "value3"),
* Map.entry("randomKey", "randomValue")
* ))
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:streamanalytics:StreamingJob sj59 /subscriptions/{subscriptionId}/resourcegroups/{resourceGroupName}/providers/Microsoft.StreamAnalytics/streamingjobs/{jobName}
* ```
*
*/
@ResourceType(type="azure-native:streamanalytics:StreamingJob")
public class StreamingJob extends com.pulumi.resources.CustomResource {
/**
* The cluster which streaming jobs will run on.
*
*/
@Export(name="cluster", refs={ClusterInfoResponse.class}, tree="[0]")
private Output* @Nullable */ ClusterInfoResponse> cluster;
/**
* @return The cluster which streaming jobs will run on.
*
*/
public Output> cluster() {
return Codegen.optional(this.cluster);
}
/**
* Controls certain runtime behaviors of the streaming job.
*
*/
@Export(name="compatibilityLevel", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> compatibilityLevel;
/**
* @return Controls certain runtime behaviors of the streaming job.
*
*/
public Output> compatibilityLevel() {
return Codegen.optional(this.compatibilityLevel);
}
/**
* Valid values are JobStorageAccount and SystemAccount. If set to JobStorageAccount, this requires the user to also specify jobStorageAccount property. .
*
*/
@Export(name="contentStoragePolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> contentStoragePolicy;
/**
* @return Valid values are JobStorageAccount and SystemAccount. If set to JobStorageAccount, this requires the user to also specify jobStorageAccount property. .
*
*/
public Output> contentStoragePolicy() {
return Codegen.optional(this.contentStoragePolicy);
}
/**
* Value is an ISO-8601 formatted UTC timestamp indicating when the streaming job was created.
*
*/
@Export(name="createdDate", refs={String.class}, tree="[0]")
private Output createdDate;
/**
* @return Value is an ISO-8601 formatted UTC timestamp indicating when the streaming job was created.
*
*/
public Output createdDate() {
return this.createdDate;
}
/**
* The data locale of the stream analytics job. Value should be the name of a supported .NET Culture from the set https://msdn.microsoft.com/en-us/library/system.globalization.culturetypes(v=vs.110).aspx. Defaults to 'en-US' if none specified.
*
*/
@Export(name="dataLocale", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dataLocale;
/**
* @return The data locale of the stream analytics job. Value should be the name of a supported .NET Culture from the set https://msdn.microsoft.com/en-us/library/system.globalization.culturetypes(v=vs.110).aspx. Defaults to 'en-US' if none specified.
*
*/
public Output> dataLocale() {
return Codegen.optional(this.dataLocale);
}
/**
* The current entity tag for the streaming job. This is an opaque string. You can use it to detect whether the resource has changed between requests. You can also use it in the If-Match or If-None-Match headers for write operations for optimistic concurrency.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return The current entity tag for the streaming job. This is an opaque string. You can use it to detect whether the resource has changed between requests. You can also use it in the If-Match or If-None-Match headers for write operations for optimistic concurrency.
*
*/
public Output etag() {
return this.etag;
}
/**
* The maximum tolerable delay in seconds where events arriving late could be included. Supported range is -1 to 1814399 (20.23:59:59 days) and -1 is used to specify wait indefinitely. If the property is absent, it is interpreted to have a value of -1.
*
*/
@Export(name="eventsLateArrivalMaxDelayInSeconds", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> eventsLateArrivalMaxDelayInSeconds;
/**
* @return The maximum tolerable delay in seconds where events arriving late could be included. Supported range is -1 to 1814399 (20.23:59:59 days) and -1 is used to specify wait indefinitely. If the property is absent, it is interpreted to have a value of -1.
*
*/
public Output> eventsLateArrivalMaxDelayInSeconds() {
return Codegen.optional(this.eventsLateArrivalMaxDelayInSeconds);
}
/**
* The maximum tolerable delay in seconds where out-of-order events can be adjusted to be back in order.
*
*/
@Export(name="eventsOutOfOrderMaxDelayInSeconds", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> eventsOutOfOrderMaxDelayInSeconds;
/**
* @return The maximum tolerable delay in seconds where out-of-order events can be adjusted to be back in order.
*
*/
public Output> eventsOutOfOrderMaxDelayInSeconds() {
return Codegen.optional(this.eventsOutOfOrderMaxDelayInSeconds);
}
/**
* Indicates the policy to apply to events that arrive out of order in the input event stream.
*
*/
@Export(name="eventsOutOfOrderPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> eventsOutOfOrderPolicy;
/**
* @return Indicates the policy to apply to events that arrive out of order in the input event stream.
*
*/
public Output> eventsOutOfOrderPolicy() {
return Codegen.optional(this.eventsOutOfOrderPolicy);
}
/**
* A list of one or more functions for the streaming job. The name property for each function is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual transformation.
*
*/
@Export(name="functions", refs={List.class,FunctionResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> functions;
/**
* @return A list of one or more functions for the streaming job. The name property for each function is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual transformation.
*
*/
public Output>> functions() {
return Codegen.optional(this.functions);
}
/**
* Describes the system-assigned managed identity assigned to this job that can be used to authenticate with inputs and outputs.
*
*/
@Export(name="identity", refs={IdentityResponse.class}, tree="[0]")
private Output* @Nullable */ IdentityResponse> identity;
/**
* @return Describes the system-assigned managed identity assigned to this job that can be used to authenticate with inputs and outputs.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* A list of one or more inputs to the streaming job. The name property for each input is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual input.
*
*/
@Export(name="inputs", refs={List.class,InputResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> inputs;
/**
* @return A list of one or more inputs to the streaming job. The name property for each input is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual input.
*
*/
public Output>> inputs() {
return Codegen.optional(this.inputs);
}
/**
* A GUID uniquely identifying the streaming job. This GUID is generated upon creation of the streaming job.
*
*/
@Export(name="jobId", refs={String.class}, tree="[0]")
private Output jobId;
/**
* @return A GUID uniquely identifying the streaming job. This GUID is generated upon creation of the streaming job.
*
*/
public Output jobId() {
return this.jobId;
}
/**
* Describes the state of the streaming job.
*
*/
@Export(name="jobState", refs={String.class}, tree="[0]")
private Output jobState;
/**
* @return Describes the state of the streaming job.
*
*/
public Output jobState() {
return this.jobState;
}
/**
* The properties that are associated with an Azure Storage account with MSI
*
*/
@Export(name="jobStorageAccount", refs={JobStorageAccountResponse.class}, tree="[0]")
private Output* @Nullable */ JobStorageAccountResponse> jobStorageAccount;
/**
* @return The properties that are associated with an Azure Storage account with MSI
*
*/
public Output> jobStorageAccount() {
return Codegen.optional(this.jobStorageAccount);
}
/**
* Describes the type of the job. Valid modes are `Cloud` and 'Edge'.
*
*/
@Export(name="jobType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> jobType;
/**
* @return Describes the type of the job. Valid modes are `Cloud` and 'Edge'.
*
*/
public Output> jobType() {
return Codegen.optional(this.jobType);
}
/**
* Value is either an ISO-8601 formatted timestamp indicating the last output event time of the streaming job or null indicating that output has not yet been produced. In case of multiple outputs or multiple streams, this shows the latest value in that set.
*
*/
@Export(name="lastOutputEventTime", refs={String.class}, tree="[0]")
private Output lastOutputEventTime;
/**
* @return Value is either an ISO-8601 formatted timestamp indicating the last output event time of the streaming job or null indicating that output has not yet been produced. In case of multiple outputs or multiple streams, this shows the latest value in that set.
*
*/
public Output lastOutputEventTime() {
return this.lastOutputEventTime;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return The geo-location where the resource lives
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Indicates the policy to apply to events that arrive at the output and cannot be written to the external storage due to being malformed (missing column values, column values of wrong type or size).
*
*/
@Export(name="outputErrorPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> outputErrorPolicy;
/**
* @return Indicates the policy to apply to events that arrive at the output and cannot be written to the external storage due to being malformed (missing column values, column values of wrong type or size).
*
*/
public Output> outputErrorPolicy() {
return Codegen.optional(this.outputErrorPolicy);
}
/**
* This property should only be utilized when it is desired that the job be started immediately upon creation. Value may be JobStartTime, CustomTime, or LastOutputEventTime to indicate whether the starting point of the output event stream should start whenever the job is started, start at a custom user time stamp specified via the outputStartTime property, or start from the last event output time.
*
*/
@Export(name="outputStartMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> outputStartMode;
/**
* @return This property should only be utilized when it is desired that the job be started immediately upon creation. Value may be JobStartTime, CustomTime, or LastOutputEventTime to indicate whether the starting point of the output event stream should start whenever the job is started, start at a custom user time stamp specified via the outputStartTime property, or start from the last event output time.
*
*/
public Output> outputStartMode() {
return Codegen.optional(this.outputStartMode);
}
/**
* Value is either an ISO-8601 formatted time stamp that indicates the starting point of the output event stream, or null to indicate that the output event stream will start whenever the streaming job is started. This property must have a value if outputStartMode is set to CustomTime.
*
*/
@Export(name="outputStartTime", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> outputStartTime;
/**
* @return Value is either an ISO-8601 formatted time stamp that indicates the starting point of the output event stream, or null to indicate that the output event stream will start whenever the streaming job is started. This property must have a value if outputStartMode is set to CustomTime.
*
*/
public Output> outputStartTime() {
return Codegen.optional(this.outputStartTime);
}
/**
* A list of one or more outputs for the streaming job. The name property for each output is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual output.
*
*/
@Export(name="outputs", refs={List.class,OutputResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> outputs;
/**
* @return A list of one or more outputs for the streaming job. The name property for each output is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual output.
*
*/
public Output>> outputs() {
return Codegen.optional(this.outputs);
}
/**
* Describes the provisioning status of the streaming job.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Describes the provisioning status of the streaming job.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Describes the SKU of the streaming job. Required on PUT (CreateOrReplace) requests.
*
*/
@Export(name="sku", refs={SkuResponse.class}, tree="[0]")
private Output* @Nullable */ SkuResponse> sku;
/**
* @return Describes the SKU of the streaming job. Required on PUT (CreateOrReplace) requests.
*
*/
public Output> sku() {
return Codegen.optional(this.sku);
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Indicates the query and the number of streaming units to use for the streaming job. The name property of the transformation is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual transformation.
*
*/
@Export(name="transformation", refs={TransformationResponse.class}, tree="[0]")
private Output* @Nullable */ TransformationResponse> transformation;
/**
* @return Indicates the query and the number of streaming units to use for the streaming job. The name property of the transformation is required when specifying this property in a PUT request. This property cannot be modify via a PATCH operation. You must use the PATCH API available for the individual transformation.
*
*/
public Output> transformation() {
return Codegen.optional(this.transformation);
}
/**
* The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public StreamingJob(java.lang.String name) {
this(name, StreamingJobArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public StreamingJob(java.lang.String name, StreamingJobArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public StreamingJob(java.lang.String name, StreamingJobArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:streamanalytics:StreamingJob", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private StreamingJob(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:streamanalytics:StreamingJob", name, null, makeResourceOptions(options, id), false);
}
private static StreamingJobArgs makeArgs(StreamingJobArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? StreamingJobArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:streamanalytics/v20160301:StreamingJob").build()),
Output.of(Alias.builder().type("azure-native:streamanalytics/v20170401preview:StreamingJob").build()),
Output.of(Alias.builder().type("azure-native:streamanalytics/v20200301:StreamingJob").build()),
Output.of(Alias.builder().type("azure-native:streamanalytics/v20211001preview:StreamingJob").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static StreamingJob get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new StreamingJob(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy