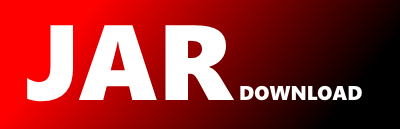
com.pulumi.azurenative.streamanalytics.outputs.AzureFunctionOutputDataSourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureFunctionOutputDataSourceResponse {
/**
* @return If you want to use an Azure Function from another subscription, you can do so by providing the key to access your function.
*
*/
private @Nullable String apiKey;
/**
* @return The name of your Azure Functions app.
*
*/
private @Nullable String functionAppName;
/**
* @return The name of the function in your Azure Functions app.
*
*/
private @Nullable String functionName;
/**
* @return A property that lets you specify the maximum number of events in each batch that's sent to Azure Functions. The default value is 100.
*
*/
private @Nullable Double maxBatchCount;
/**
* @return A property that lets you set the maximum size for each output batch that's sent to your Azure function. The input unit is in bytes. By default, this value is 262,144 bytes (256 KB).
*
*/
private @Nullable Double maxBatchSize;
/**
* @return Indicates the type of data source output will be written to. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.AzureFunction'.
*
*/
private String type;
private AzureFunctionOutputDataSourceResponse() {}
/**
* @return If you want to use an Azure Function from another subscription, you can do so by providing the key to access your function.
*
*/
public Optional apiKey() {
return Optional.ofNullable(this.apiKey);
}
/**
* @return The name of your Azure Functions app.
*
*/
public Optional functionAppName() {
return Optional.ofNullable(this.functionAppName);
}
/**
* @return The name of the function in your Azure Functions app.
*
*/
public Optional functionName() {
return Optional.ofNullable(this.functionName);
}
/**
* @return A property that lets you specify the maximum number of events in each batch that's sent to Azure Functions. The default value is 100.
*
*/
public Optional maxBatchCount() {
return Optional.ofNullable(this.maxBatchCount);
}
/**
* @return A property that lets you set the maximum size for each output batch that's sent to your Azure function. The input unit is in bytes. By default, this value is 262,144 bytes (256 KB).
*
*/
public Optional maxBatchSize() {
return Optional.ofNullable(this.maxBatchSize);
}
/**
* @return Indicates the type of data source output will be written to. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.AzureFunction'.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureFunctionOutputDataSourceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String apiKey;
private @Nullable String functionAppName;
private @Nullable String functionName;
private @Nullable Double maxBatchCount;
private @Nullable Double maxBatchSize;
private String type;
public Builder() {}
public Builder(AzureFunctionOutputDataSourceResponse defaults) {
Objects.requireNonNull(defaults);
this.apiKey = defaults.apiKey;
this.functionAppName = defaults.functionAppName;
this.functionName = defaults.functionName;
this.maxBatchCount = defaults.maxBatchCount;
this.maxBatchSize = defaults.maxBatchSize;
this.type = defaults.type;
}
@CustomType.Setter
public Builder apiKey(@Nullable String apiKey) {
this.apiKey = apiKey;
return this;
}
@CustomType.Setter
public Builder functionAppName(@Nullable String functionAppName) {
this.functionAppName = functionAppName;
return this;
}
@CustomType.Setter
public Builder functionName(@Nullable String functionName) {
this.functionName = functionName;
return this;
}
@CustomType.Setter
public Builder maxBatchCount(@Nullable Double maxBatchCount) {
this.maxBatchCount = maxBatchCount;
return this;
}
@CustomType.Setter
public Builder maxBatchSize(@Nullable Double maxBatchSize) {
this.maxBatchSize = maxBatchSize;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("AzureFunctionOutputDataSourceResponse", "type");
}
this.type = type;
return this;
}
public AzureFunctionOutputDataSourceResponse build() {
final var _resultValue = new AzureFunctionOutputDataSourceResponse();
_resultValue.apiKey = apiKey;
_resultValue.functionAppName = functionAppName;
_resultValue.functionName = functionName;
_resultValue.maxBatchCount = maxBatchCount;
_resultValue.maxBatchSize = maxBatchSize;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy