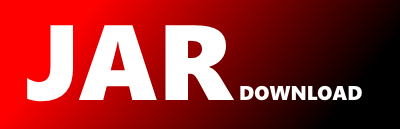
com.pulumi.azurenative.synapse.WorkspaceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.synapse;
import com.pulumi.azurenative.synapse.enums.WorkspacePublicNetworkAccess;
import com.pulumi.azurenative.synapse.inputs.CspWorkspaceAdminPropertiesArgs;
import com.pulumi.azurenative.synapse.inputs.DataLakeStorageAccountDetailsArgs;
import com.pulumi.azurenative.synapse.inputs.EncryptionDetailsArgs;
import com.pulumi.azurenative.synapse.inputs.ManagedIdentityArgs;
import com.pulumi.azurenative.synapse.inputs.ManagedVirtualNetworkSettingsArgs;
import com.pulumi.azurenative.synapse.inputs.PrivateEndpointConnectionArgs;
import com.pulumi.azurenative.synapse.inputs.PurviewConfigurationArgs;
import com.pulumi.azurenative.synapse.inputs.VirtualNetworkProfileArgs;
import com.pulumi.azurenative.synapse.inputs.WorkspaceRepositoryConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkspaceArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkspaceArgs Empty = new WorkspaceArgs();
/**
* Enable or Disable AzureADOnlyAuthentication on All Workspace subresource
*
*/
@Import(name="azureADOnlyAuthentication")
private @Nullable Output azureADOnlyAuthentication;
/**
* @return Enable or Disable AzureADOnlyAuthentication on All Workspace subresource
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy