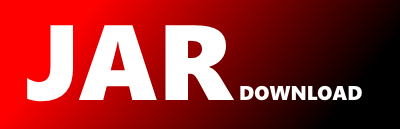
com.pulumi.azurenative.synapse.outputs.GetWorkspaceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.synapse.outputs;
import com.pulumi.azurenative.synapse.outputs.CspWorkspaceAdminPropertiesResponse;
import com.pulumi.azurenative.synapse.outputs.DataLakeStorageAccountDetailsResponse;
import com.pulumi.azurenative.synapse.outputs.EncryptionDetailsResponse;
import com.pulumi.azurenative.synapse.outputs.ManagedIdentityResponse;
import com.pulumi.azurenative.synapse.outputs.ManagedVirtualNetworkSettingsResponse;
import com.pulumi.azurenative.synapse.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.synapse.outputs.PurviewConfigurationResponse;
import com.pulumi.azurenative.synapse.outputs.VirtualNetworkProfileResponse;
import com.pulumi.azurenative.synapse.outputs.WorkspaceRepositoryConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWorkspaceResult {
/**
* @return The ADLA resource ID.
*
*/
private String adlaResourceId;
/**
* @return Connectivity endpoints
*
*/
private Map connectivityEndpoints;
/**
* @return Initial workspace AAD admin properties for a CSP subscription
*
*/
private @Nullable CspWorkspaceAdminPropertiesResponse cspWorkspaceAdminProperties;
/**
* @return Workspace default data lake storage account details
*
*/
private @Nullable DataLakeStorageAccountDetailsResponse defaultDataLakeStorage;
/**
* @return The encryption details of the workspace
*
*/
private @Nullable EncryptionDetailsResponse encryption;
/**
* @return Workspace level configs and feature flags
*
*/
private Object extraProperties;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Identity of the workspace
*
*/
private @Nullable ManagedIdentityResponse identity;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Workspace managed resource group. The resource group name uniquely identifies the resource group within the user subscriptionId. The resource group name must be no longer than 90 characters long, and must be alphanumeric characters (Char.IsLetterOrDigit()) and '-', '_', '(', ')' and'.'. Note that the name cannot end with '.'
*
*/
private @Nullable String managedResourceGroupName;
/**
* @return Setting this to 'default' will ensure that all compute for this workspace is in a virtual network managed on behalf of the user.
*
*/
private @Nullable String managedVirtualNetwork;
/**
* @return Managed Virtual Network Settings
*
*/
private @Nullable ManagedVirtualNetworkSettingsResponse managedVirtualNetworkSettings;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Private endpoint connections to the workspace
*
*/
private @Nullable List privateEndpointConnections;
/**
* @return Resource provisioning state
*
*/
private String provisioningState;
/**
* @return Enable or Disable public network access to workspace
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Purview Configuration
*
*/
private @Nullable PurviewConfigurationResponse purviewConfiguration;
/**
* @return Workspace settings
*
*/
private Map settings;
/**
* @return Login for workspace SQL active directory administrator
*
*/
private @Nullable String sqlAdministratorLogin;
/**
* @return SQL administrator login password
*
*/
private @Nullable String sqlAdministratorLoginPassword;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Is trustedServiceBypassEnabled for the workspace
*
*/
private @Nullable Boolean trustedServiceBypassEnabled;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Virtual Network profile
*
*/
private @Nullable VirtualNetworkProfileResponse virtualNetworkProfile;
/**
* @return Git integration settings
*
*/
private @Nullable WorkspaceRepositoryConfigurationResponse workspaceRepositoryConfiguration;
/**
* @return The workspace unique identifier
*
*/
private String workspaceUID;
private GetWorkspaceResult() {}
/**
* @return The ADLA resource ID.
*
*/
public String adlaResourceId() {
return this.adlaResourceId;
}
/**
* @return Connectivity endpoints
*
*/
public Map connectivityEndpoints() {
return this.connectivityEndpoints;
}
/**
* @return Initial workspace AAD admin properties for a CSP subscription
*
*/
public Optional cspWorkspaceAdminProperties() {
return Optional.ofNullable(this.cspWorkspaceAdminProperties);
}
/**
* @return Workspace default data lake storage account details
*
*/
public Optional defaultDataLakeStorage() {
return Optional.ofNullable(this.defaultDataLakeStorage);
}
/**
* @return The encryption details of the workspace
*
*/
public Optional encryption() {
return Optional.ofNullable(this.encryption);
}
/**
* @return Workspace level configs and feature flags
*
*/
public Object extraProperties() {
return this.extraProperties;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Identity of the workspace
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return Workspace managed resource group. The resource group name uniquely identifies the resource group within the user subscriptionId. The resource group name must be no longer than 90 characters long, and must be alphanumeric characters (Char.IsLetterOrDigit()) and '-', '_', '(', ')' and'.'. Note that the name cannot end with '.'
*
*/
public Optional managedResourceGroupName() {
return Optional.ofNullable(this.managedResourceGroupName);
}
/**
* @return Setting this to 'default' will ensure that all compute for this workspace is in a virtual network managed on behalf of the user.
*
*/
public Optional managedVirtualNetwork() {
return Optional.ofNullable(this.managedVirtualNetwork);
}
/**
* @return Managed Virtual Network Settings
*
*/
public Optional managedVirtualNetworkSettings() {
return Optional.ofNullable(this.managedVirtualNetworkSettings);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Private endpoint connections to the workspace
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections == null ? List.of() : this.privateEndpointConnections;
}
/**
* @return Resource provisioning state
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Enable or Disable public network access to workspace
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Purview Configuration
*
*/
public Optional purviewConfiguration() {
return Optional.ofNullable(this.purviewConfiguration);
}
/**
* @return Workspace settings
*
*/
public Map settings() {
return this.settings;
}
/**
* @return Login for workspace SQL active directory administrator
*
*/
public Optional sqlAdministratorLogin() {
return Optional.ofNullable(this.sqlAdministratorLogin);
}
/**
* @return SQL administrator login password
*
*/
public Optional sqlAdministratorLoginPassword() {
return Optional.ofNullable(this.sqlAdministratorLoginPassword);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Is trustedServiceBypassEnabled for the workspace
*
*/
public Optional trustedServiceBypassEnabled() {
return Optional.ofNullable(this.trustedServiceBypassEnabled);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Virtual Network profile
*
*/
public Optional virtualNetworkProfile() {
return Optional.ofNullable(this.virtualNetworkProfile);
}
/**
* @return Git integration settings
*
*/
public Optional workspaceRepositoryConfiguration() {
return Optional.ofNullable(this.workspaceRepositoryConfiguration);
}
/**
* @return The workspace unique identifier
*
*/
public String workspaceUID() {
return this.workspaceUID;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWorkspaceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String adlaResourceId;
private Map connectivityEndpoints;
private @Nullable CspWorkspaceAdminPropertiesResponse cspWorkspaceAdminProperties;
private @Nullable DataLakeStorageAccountDetailsResponse defaultDataLakeStorage;
private @Nullable EncryptionDetailsResponse encryption;
private Object extraProperties;
private String id;
private @Nullable ManagedIdentityResponse identity;
private String location;
private @Nullable String managedResourceGroupName;
private @Nullable String managedVirtualNetwork;
private @Nullable ManagedVirtualNetworkSettingsResponse managedVirtualNetworkSettings;
private String name;
private @Nullable List privateEndpointConnections;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private @Nullable PurviewConfigurationResponse purviewConfiguration;
private Map settings;
private @Nullable String sqlAdministratorLogin;
private @Nullable String sqlAdministratorLoginPassword;
private @Nullable Map tags;
private @Nullable Boolean trustedServiceBypassEnabled;
private String type;
private @Nullable VirtualNetworkProfileResponse virtualNetworkProfile;
private @Nullable WorkspaceRepositoryConfigurationResponse workspaceRepositoryConfiguration;
private String workspaceUID;
public Builder() {}
public Builder(GetWorkspaceResult defaults) {
Objects.requireNonNull(defaults);
this.adlaResourceId = defaults.adlaResourceId;
this.connectivityEndpoints = defaults.connectivityEndpoints;
this.cspWorkspaceAdminProperties = defaults.cspWorkspaceAdminProperties;
this.defaultDataLakeStorage = defaults.defaultDataLakeStorage;
this.encryption = defaults.encryption;
this.extraProperties = defaults.extraProperties;
this.id = defaults.id;
this.identity = defaults.identity;
this.location = defaults.location;
this.managedResourceGroupName = defaults.managedResourceGroupName;
this.managedVirtualNetwork = defaults.managedVirtualNetwork;
this.managedVirtualNetworkSettings = defaults.managedVirtualNetworkSettings;
this.name = defaults.name;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.purviewConfiguration = defaults.purviewConfiguration;
this.settings = defaults.settings;
this.sqlAdministratorLogin = defaults.sqlAdministratorLogin;
this.sqlAdministratorLoginPassword = defaults.sqlAdministratorLoginPassword;
this.tags = defaults.tags;
this.trustedServiceBypassEnabled = defaults.trustedServiceBypassEnabled;
this.type = defaults.type;
this.virtualNetworkProfile = defaults.virtualNetworkProfile;
this.workspaceRepositoryConfiguration = defaults.workspaceRepositoryConfiguration;
this.workspaceUID = defaults.workspaceUID;
}
@CustomType.Setter
public Builder adlaResourceId(String adlaResourceId) {
if (adlaResourceId == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "adlaResourceId");
}
this.adlaResourceId = adlaResourceId;
return this;
}
@CustomType.Setter
public Builder connectivityEndpoints(Map connectivityEndpoints) {
if (connectivityEndpoints == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "connectivityEndpoints");
}
this.connectivityEndpoints = connectivityEndpoints;
return this;
}
@CustomType.Setter
public Builder cspWorkspaceAdminProperties(@Nullable CspWorkspaceAdminPropertiesResponse cspWorkspaceAdminProperties) {
this.cspWorkspaceAdminProperties = cspWorkspaceAdminProperties;
return this;
}
@CustomType.Setter
public Builder defaultDataLakeStorage(@Nullable DataLakeStorageAccountDetailsResponse defaultDataLakeStorage) {
this.defaultDataLakeStorage = defaultDataLakeStorage;
return this;
}
@CustomType.Setter
public Builder encryption(@Nullable EncryptionDetailsResponse encryption) {
this.encryption = encryption;
return this;
}
@CustomType.Setter
public Builder extraProperties(Object extraProperties) {
if (extraProperties == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "extraProperties");
}
this.extraProperties = extraProperties;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable ManagedIdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedResourceGroupName(@Nullable String managedResourceGroupName) {
this.managedResourceGroupName = managedResourceGroupName;
return this;
}
@CustomType.Setter
public Builder managedVirtualNetwork(@Nullable String managedVirtualNetwork) {
this.managedVirtualNetwork = managedVirtualNetwork;
return this;
}
@CustomType.Setter
public Builder managedVirtualNetworkSettings(@Nullable ManagedVirtualNetworkSettingsResponse managedVirtualNetworkSettings) {
this.managedVirtualNetworkSettings = managedVirtualNetworkSettings;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(@Nullable List privateEndpointConnections) {
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder purviewConfiguration(@Nullable PurviewConfigurationResponse purviewConfiguration) {
this.purviewConfiguration = purviewConfiguration;
return this;
}
@CustomType.Setter
public Builder settings(Map settings) {
if (settings == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "settings");
}
this.settings = settings;
return this;
}
@CustomType.Setter
public Builder sqlAdministratorLogin(@Nullable String sqlAdministratorLogin) {
this.sqlAdministratorLogin = sqlAdministratorLogin;
return this;
}
@CustomType.Setter
public Builder sqlAdministratorLoginPassword(@Nullable String sqlAdministratorLoginPassword) {
this.sqlAdministratorLoginPassword = sqlAdministratorLoginPassword;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder trustedServiceBypassEnabled(@Nullable Boolean trustedServiceBypassEnabled) {
this.trustedServiceBypassEnabled = trustedServiceBypassEnabled;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualNetworkProfile(@Nullable VirtualNetworkProfileResponse virtualNetworkProfile) {
this.virtualNetworkProfile = virtualNetworkProfile;
return this;
}
@CustomType.Setter
public Builder workspaceRepositoryConfiguration(@Nullable WorkspaceRepositoryConfigurationResponse workspaceRepositoryConfiguration) {
this.workspaceRepositoryConfiguration = workspaceRepositoryConfiguration;
return this;
}
@CustomType.Setter
public Builder workspaceUID(String workspaceUID) {
if (workspaceUID == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "workspaceUID");
}
this.workspaceUID = workspaceUID;
return this;
}
public GetWorkspaceResult build() {
final var _resultValue = new GetWorkspaceResult();
_resultValue.adlaResourceId = adlaResourceId;
_resultValue.connectivityEndpoints = connectivityEndpoints;
_resultValue.cspWorkspaceAdminProperties = cspWorkspaceAdminProperties;
_resultValue.defaultDataLakeStorage = defaultDataLakeStorage;
_resultValue.encryption = encryption;
_resultValue.extraProperties = extraProperties;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.location = location;
_resultValue.managedResourceGroupName = managedResourceGroupName;
_resultValue.managedVirtualNetwork = managedVirtualNetwork;
_resultValue.managedVirtualNetworkSettings = managedVirtualNetworkSettings;
_resultValue.name = name;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.purviewConfiguration = purviewConfiguration;
_resultValue.settings = settings;
_resultValue.sqlAdministratorLogin = sqlAdministratorLogin;
_resultValue.sqlAdministratorLoginPassword = sqlAdministratorLoginPassword;
_resultValue.tags = tags;
_resultValue.trustedServiceBypassEnabled = trustedServiceBypassEnabled;
_resultValue.type = type;
_resultValue.virtualNetworkProfile = virtualNetworkProfile;
_resultValue.workspaceRepositoryConfiguration = workspaceRepositoryConfiguration;
_resultValue.workspaceUID = workspaceUID;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy