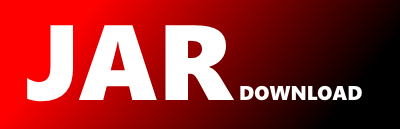
com.pulumi.azurenative.testbase.DraftPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.testbase;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.testbase.DraftPackageArgs;
import com.pulumi.azurenative.testbase.outputs.DraftPackageIntuneAppMetadataResponse;
import com.pulumi.azurenative.testbase.outputs.FirstPartyAppDefinitionResponse;
import com.pulumi.azurenative.testbase.outputs.GalleryAppDefinitionResponse;
import com.pulumi.azurenative.testbase.outputs.HighlightedFileResponse;
import com.pulumi.azurenative.testbase.outputs.InplaceUpgradeOSInfoResponse;
import com.pulumi.azurenative.testbase.outputs.IntuneEnrollmentMetadataResponse;
import com.pulumi.azurenative.testbase.outputs.SystemDataResponse;
import com.pulumi.azurenative.testbase.outputs.TabStateResponse;
import com.pulumi.azurenative.testbase.outputs.TargetOSInfoResponse;
import com.pulumi.azurenative.testbase.outputs.TestResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The Test Base Draft Package resource.
* Azure REST API version: 2023-11-01-preview.
*
* ## Example Usage
* ### DraftPackageCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.testbase.DraftPackage;
* import com.pulumi.azurenative.testbase.DraftPackageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var draftPackage = new DraftPackage("draftPackage", DraftPackageArgs.builder()
* .appFileName("TestBaseM365DigitalClock.msi")
* .applicationName("contoso-package")
* .draftPackageName("61d99543-14ff-47ae-bf03-8a8b8445502e")
* .resourceGroupName("contoso-rg1")
* .sourceType("Native")
* .testBaseAccountName("contoso-testBaseAccount1")
* .useSample(false)
* .version("1.0")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:testbase:DraftPackage 61d99543-14ff-47ae-bf03-8a8b8445502e /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.TestBase/testBaseAccounts/{testBaseAccountName}/draftPackages/{draftPackageName}
* ```
*
*/
@ResourceType(type="azure-native:testbase:DraftPackage")
public class DraftPackage extends com.pulumi.resources.CustomResource {
/**
* The name of the app file.
*
*/
@Export(name="appFileName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> appFileName;
/**
* @return The name of the app file.
*
*/
public Output> appFileName() {
return Codegen.optional(this.appFileName);
}
/**
* Application name
*
*/
@Export(name="applicationName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> applicationName;
/**
* @return Application name
*
*/
public Output> applicationName() {
return Codegen.optional(this.applicationName);
}
/**
* Comments added by user.
*
*/
@Export(name="comments", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> comments;
/**
* @return Comments added by user.
*
*/
public Output> comments() {
return Codegen.optional(this.comments);
}
/**
* The relative path of the folder hosting package files.
*
*/
@Export(name="draftPackagePath", refs={String.class}, tree="[0]")
private Output draftPackagePath;
/**
* @return The relative path of the folder hosting package files.
*
*/
public Output draftPackagePath() {
return this.draftPackagePath;
}
/**
* Specifies whether this draft package is used to edit a package.
*
*/
@Export(name="editPackage", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> editPackage;
/**
* @return Specifies whether this draft package is used to edit a package.
*
*/
public Output> editPackage() {
return Codegen.optional(this.editPackage);
}
/**
* The executable launch command for script auto-fill. Will be used to run the application.
*
*/
@Export(name="executableLaunchCommand", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> executableLaunchCommand;
/**
* @return The executable launch command for script auto-fill. Will be used to run the application.
*
*/
public Output> executableLaunchCommand() {
return Codegen.optional(this.executableLaunchCommand);
}
/**
* The list of first party applications to test along with user application.
*
*/
@Export(name="firstPartyApps", refs={List.class,FirstPartyAppDefinitionResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> firstPartyApps;
/**
* @return The list of first party applications to test along with user application.
*
*/
public Output>> firstPartyApps() {
return Codegen.optional(this.firstPartyApps);
}
/**
* The flighting ring for feature update.
*
*/
@Export(name="flightingRing", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> flightingRing;
/**
* @return The flighting ring for feature update.
*
*/
public Output> flightingRing() {
return Codegen.optional(this.flightingRing);
}
/**
* The list of gallery apps to test along with user application.
*
*/
@Export(name="galleryApps", refs={List.class,GalleryAppDefinitionResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> galleryApps;
/**
* @return The list of gallery apps to test along with user application.
*
*/
public Output>> galleryApps() {
return Codegen.optional(this.galleryApps);
}
/**
* The highlight files in the package.
*
*/
@Export(name="highlightedFiles", refs={List.class,HighlightedFileResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> highlightedFiles;
/**
* @return The highlight files in the package.
*
*/
public Output>> highlightedFiles() {
return Codegen.optional(this.highlightedFiles);
}
/**
* Specifies the baseline os and target os for inplace upgrade.
*
*/
@Export(name="inplaceUpgradeOSPair", refs={InplaceUpgradeOSInfoResponse.class}, tree="[0]")
private Output* @Nullable */ InplaceUpgradeOSInfoResponse> inplaceUpgradeOSPair;
/**
* @return Specifies the baseline os and target os for inplace upgrade.
*
*/
public Output> inplaceUpgradeOSPair() {
return Codegen.optional(this.inplaceUpgradeOSPair);
}
/**
* The metadata of Intune enrollment.
*
*/
@Export(name="intuneEnrollmentMetadata", refs={IntuneEnrollmentMetadataResponse.class}, tree="[0]")
private Output* @Nullable */ IntuneEnrollmentMetadataResponse> intuneEnrollmentMetadata;
/**
* @return The metadata of Intune enrollment.
*
*/
public Output> intuneEnrollmentMetadata() {
return Codegen.optional(this.intuneEnrollmentMetadata);
}
/**
* Metadata used to generate draft package folder and scripts.
*
*/
@Export(name="intuneMetadata", refs={DraftPackageIntuneAppMetadataResponse.class}, tree="[0]")
private Output* @Nullable */ DraftPackageIntuneAppMetadataResponse> intuneMetadata;
/**
* @return Metadata used to generate draft package folder and scripts.
*
*/
public Output> intuneMetadata() {
return Codegen.optional(this.intuneMetadata);
}
/**
* The UTC timestamp when the package was last modified.
*
*/
@Export(name="lastModifiedTime", refs={String.class}, tree="[0]")
private Output lastModifiedTime;
/**
* @return The UTC timestamp when the package was last modified.
*
*/
public Output lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Specifies the package id from which the draft package copied.
*
*/
@Export(name="packageId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> packageId;
/**
* @return Specifies the package id from which the draft package copied.
*
*/
public Output> packageId() {
return Codegen.optional(this.packageId);
}
/**
* Tags of the package to be created.
*
*/
@Export(name="packageTags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> packageTags;
/**
* @return Tags of the package to be created.
*
*/
public Output>> packageTags() {
return Codegen.optional(this.packageTags);
}
/**
* The process name for script auto-fill. Will be used to identify the application process.
*
*/
@Export(name="processName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> processName;
/**
* @return The process name for script auto-fill. Will be used to identify the application process.
*
*/
public Output> processName() {
return Codegen.optional(this.processName);
}
/**
* The provisioning state of the resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The source type.
*
*/
@Export(name="sourceType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sourceType;
/**
* @return The source type.
*
*/
public Output> sourceType() {
return Codegen.optional(this.sourceType);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Tab state.
*
*/
@Export(name="tabState", refs={TabStateResponse.class}, tree="[0]")
private Output* @Nullable */ TabStateResponse> tabState;
/**
* @return Tab state.
*
*/
public Output> tabState() {
return Codegen.optional(this.tabState);
}
/**
* Specifies the target OSs of specific OS Update types.
*
*/
@Export(name="targetOSList", refs={List.class,TargetOSInfoResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> targetOSList;
/**
* @return Specifies the target OSs of specific OS Update types.
*
*/
public Output>> targetOSList() {
return Codegen.optional(this.targetOSList);
}
/**
* OOB, functional or flow driven. Mapped to the data in 'tests' property.
*
*/
@Export(name="testTypes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> testTypes;
/**
* @return OOB, functional or flow driven. Mapped to the data in 'tests' property.
*
*/
public Output>> testTypes() {
return Codegen.optional(this.testTypes);
}
/**
* The detailed test information.
*
*/
@Export(name="tests", refs={List.class,TestResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> tests;
/**
* @return The detailed test information.
*
*/
public Output>> tests() {
return Codegen.optional(this.tests);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* Indicates whether user choose to enable script auto-fill.
*
*/
@Export(name="useAutofill", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> useAutofill;
/**
* @return Indicates whether user choose to enable script auto-fill.
*
*/
public Output> useAutofill() {
return Codegen.optional(this.useAutofill);
}
/**
* Specifies whether a sample package should be used instead of the one uploaded by the user.
*
*/
@Export(name="useSample", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> useSample;
/**
* @return Specifies whether a sample package should be used instead of the one uploaded by the user.
*
*/
public Output> useSample() {
return Codegen.optional(this.useSample);
}
/**
* Application version
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> version;
/**
* @return Application version
*
*/
public Output> version() {
return Codegen.optional(this.version);
}
/**
* The relative path for a temporarily folder for package creation work.
*
*/
@Export(name="workingPath", refs={String.class}, tree="[0]")
private Output workingPath;
/**
* @return The relative path for a temporarily folder for package creation work.
*
*/
public Output workingPath() {
return this.workingPath;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public DraftPackage(java.lang.String name) {
this(name, DraftPackageArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public DraftPackage(java.lang.String name, DraftPackageArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public DraftPackage(java.lang.String name, DraftPackageArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:testbase:DraftPackage", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private DraftPackage(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:testbase:DraftPackage", name, null, makeResourceOptions(options, id), false);
}
private static DraftPackageArgs makeArgs(DraftPackageArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? DraftPackageArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:testbase/v20231101preview:DraftPackage").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static DraftPackage get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new DraftPackage(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy