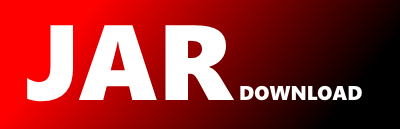
com.pulumi.azurenative.timeseriesinsights.Gen2EnvironmentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights;
import com.pulumi.azurenative.timeseriesinsights.inputs.Gen2StorageConfigurationInputArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.SkuArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.TimeSeriesIdPropertyArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.WarmStoreConfigurationPropertiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class Gen2EnvironmentArgs extends com.pulumi.resources.ResourceArgs {
public static final Gen2EnvironmentArgs Empty = new Gen2EnvironmentArgs();
/**
* Name of the environment
*
*/
@Import(name="environmentName")
private @Nullable Output environmentName;
/**
* @return Name of the environment
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy