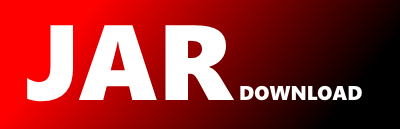
com.pulumi.azurenative.timeseriesinsights.TimeseriesinsightsFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetAccessPolicyArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetAccessPolicyPlainArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetEventHubEventSourceArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetEventHubEventSourcePlainArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetGen1EnvironmentArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetGen1EnvironmentPlainArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetGen2EnvironmentArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetGen2EnvironmentPlainArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetIoTHubEventSourceArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetIoTHubEventSourcePlainArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetReferenceDataSetArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.GetReferenceDataSetPlainArgs;
import com.pulumi.azurenative.timeseriesinsights.outputs.GetAccessPolicyResult;
import com.pulumi.azurenative.timeseriesinsights.outputs.GetEventHubEventSourceResult;
import com.pulumi.azurenative.timeseriesinsights.outputs.GetGen1EnvironmentResult;
import com.pulumi.azurenative.timeseriesinsights.outputs.GetGen2EnvironmentResult;
import com.pulumi.azurenative.timeseriesinsights.outputs.GetIoTHubEventSourceResult;
import com.pulumi.azurenative.timeseriesinsights.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.timeseriesinsights.outputs.GetReferenceDataSetResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class TimeseriesinsightsFunctions {
/**
* Gets the access policy with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static Output getAccessPolicy(GetAccessPolicyArgs args) {
return getAccessPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access policy with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static CompletableFuture getAccessPolicyPlain(GetAccessPolicyPlainArgs args) {
return getAccessPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access policy with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static Output getAccessPolicy(GetAccessPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:timeseriesinsights:getAccessPolicy", TypeShape.of(GetAccessPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access policy with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static CompletableFuture getAccessPolicyPlain(GetAccessPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:timeseriesinsights:getAccessPolicy", TypeShape.of(GetAccessPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getEventHubEventSource(GetEventHubEventSourceArgs args) {
return getEventHubEventSource(args, InvokeOptions.Empty);
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getEventHubEventSourcePlain(GetEventHubEventSourcePlainArgs args) {
return getEventHubEventSourcePlain(args, InvokeOptions.Empty);
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getEventHubEventSource(GetEventHubEventSourceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:timeseriesinsights:getEventHubEventSource", TypeShape.of(GetEventHubEventSourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getEventHubEventSourcePlain(GetEventHubEventSourcePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:timeseriesinsights:getEventHubEventSource", TypeShape.of(GetEventHubEventSourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getGen1Environment(GetGen1EnvironmentArgs args) {
return getGen1Environment(args, InvokeOptions.Empty);
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getGen1EnvironmentPlain(GetGen1EnvironmentPlainArgs args) {
return getGen1EnvironmentPlain(args, InvokeOptions.Empty);
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getGen1Environment(GetGen1EnvironmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:timeseriesinsights:getGen1Environment", TypeShape.of(GetGen1EnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getGen1EnvironmentPlain(GetGen1EnvironmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:timeseriesinsights:getGen1Environment", TypeShape.of(GetGen1EnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getGen2Environment(GetGen2EnvironmentArgs args) {
return getGen2Environment(args, InvokeOptions.Empty);
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getGen2EnvironmentPlain(GetGen2EnvironmentPlainArgs args) {
return getGen2EnvironmentPlain(args, InvokeOptions.Empty);
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getGen2Environment(GetGen2EnvironmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:timeseriesinsights:getGen2Environment", TypeShape.of(GetGen2EnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the environment with the specified name in the specified subscription and resource group.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getGen2EnvironmentPlain(GetGen2EnvironmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:timeseriesinsights:getGen2Environment", TypeShape.of(GetGen2EnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getIoTHubEventSource(GetIoTHubEventSourceArgs args) {
return getIoTHubEventSource(args, InvokeOptions.Empty);
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getIoTHubEventSourcePlain(GetIoTHubEventSourcePlainArgs args) {
return getIoTHubEventSourcePlain(args, InvokeOptions.Empty);
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static Output getIoTHubEventSource(GetIoTHubEventSourceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:timeseriesinsights:getIoTHubEventSource", TypeShape.of(GetIoTHubEventSourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the event source with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
*/
public static CompletableFuture getIoTHubEventSourcePlain(GetIoTHubEventSourcePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:timeseriesinsights:getIoTHubEventSource", TypeShape.of(GetIoTHubEventSourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the private endpoint connection of the environment in the given resource group.
* Azure REST API version: 2021-03-31-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the details of the private endpoint connection of the environment in the given resource group.
* Azure REST API version: 2021-03-31-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of the private endpoint connection of the environment in the given resource group.
* Azure REST API version: 2021-03-31-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:timeseriesinsights:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the private endpoint connection of the environment in the given resource group.
* Azure REST API version: 2021-03-31-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:timeseriesinsights:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the reference data set with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static Output getReferenceDataSet(GetReferenceDataSetArgs args) {
return getReferenceDataSet(args, InvokeOptions.Empty);
}
/**
* Gets the reference data set with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static CompletableFuture getReferenceDataSetPlain(GetReferenceDataSetPlainArgs args) {
return getReferenceDataSetPlain(args, InvokeOptions.Empty);
}
/**
* Gets the reference data set with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static Output getReferenceDataSet(GetReferenceDataSetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:timeseriesinsights:getReferenceDataSet", TypeShape.of(GetReferenceDataSetResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the reference data set with the specified name in the specified environment.
* Azure REST API version: 2020-05-15.
*
* Other available API versions: 2017-11-15, 2021-06-30-preview.
*
*/
public static CompletableFuture getReferenceDataSetPlain(GetReferenceDataSetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:timeseriesinsights:getReferenceDataSet", TypeShape.of(GetReferenceDataSetResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy