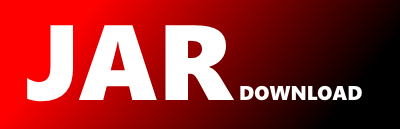
com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.videoanalyzer.inputs.GetAccessPolicyArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetAccessPolicyPlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetEdgeModuleArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetEdgeModulePlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetLivePipelineArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetLivePipelinePlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetPipelineJobArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetPipelineJobPlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetPipelineTopologyArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetPipelineTopologyPlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetVideoAnalyzerArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetVideoAnalyzerPlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetVideoArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.GetVideoPlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.ListEdgeModuleProvisioningTokenArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.ListEdgeModuleProvisioningTokenPlainArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.ListVideoContentTokenArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.ListVideoContentTokenPlainArgs;
import com.pulumi.azurenative.videoanalyzer.outputs.GetAccessPolicyResult;
import com.pulumi.azurenative.videoanalyzer.outputs.GetEdgeModuleResult;
import com.pulumi.azurenative.videoanalyzer.outputs.GetLivePipelineResult;
import com.pulumi.azurenative.videoanalyzer.outputs.GetPipelineJobResult;
import com.pulumi.azurenative.videoanalyzer.outputs.GetPipelineTopologyResult;
import com.pulumi.azurenative.videoanalyzer.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.videoanalyzer.outputs.GetVideoAnalyzerResult;
import com.pulumi.azurenative.videoanalyzer.outputs.GetVideoResult;
import com.pulumi.azurenative.videoanalyzer.outputs.ListEdgeModuleProvisioningTokenResult;
import com.pulumi.azurenative.videoanalyzer.outputs.ListVideoContentTokenResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class VideoanalyzerFunctions {
/**
* Retrieves an existing access policy resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getAccessPolicy(GetAccessPolicyArgs args) {
return getAccessPolicy(args, InvokeOptions.Empty);
}
/**
* Retrieves an existing access policy resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getAccessPolicyPlain(GetAccessPolicyPlainArgs args) {
return getAccessPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves an existing access policy resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getAccessPolicy(GetAccessPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getAccessPolicy", TypeShape.of(GetAccessPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves an existing access policy resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getAccessPolicyPlain(GetAccessPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getAccessPolicy", TypeShape.of(GetAccessPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves an existing edge module resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getEdgeModule(GetEdgeModuleArgs args) {
return getEdgeModule(args, InvokeOptions.Empty);
}
/**
* Retrieves an existing edge module resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getEdgeModulePlain(GetEdgeModulePlainArgs args) {
return getEdgeModulePlain(args, InvokeOptions.Empty);
}
/**
* Retrieves an existing edge module resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getEdgeModule(GetEdgeModuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getEdgeModule", TypeShape.of(GetEdgeModuleResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves an existing edge module resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getEdgeModulePlain(GetEdgeModulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getEdgeModule", TypeShape.of(GetEdgeModuleResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a specific live pipeline by name. If a live pipeline with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getLivePipeline(GetLivePipelineArgs args) {
return getLivePipeline(args, InvokeOptions.Empty);
}
/**
* Retrieves a specific live pipeline by name. If a live pipeline with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getLivePipelinePlain(GetLivePipelinePlainArgs args) {
return getLivePipelinePlain(args, InvokeOptions.Empty);
}
/**
* Retrieves a specific live pipeline by name. If a live pipeline with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getLivePipeline(GetLivePipelineArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getLivePipeline", TypeShape.of(GetLivePipelineResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a specific live pipeline by name. If a live pipeline with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getLivePipelinePlain(GetLivePipelinePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getLivePipeline", TypeShape.of(GetLivePipelineResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a specific pipeline job by name. If a pipeline job with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getPipelineJob(GetPipelineJobArgs args) {
return getPipelineJob(args, InvokeOptions.Empty);
}
/**
* Retrieves a specific pipeline job by name. If a pipeline job with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getPipelineJobPlain(GetPipelineJobPlainArgs args) {
return getPipelineJobPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves a specific pipeline job by name. If a pipeline job with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getPipelineJob(GetPipelineJobArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getPipelineJob", TypeShape.of(GetPipelineJobResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a specific pipeline job by name. If a pipeline job with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getPipelineJobPlain(GetPipelineJobPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getPipelineJob", TypeShape.of(GetPipelineJobResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a specific pipeline topology by name. If a topology with that name has been previously created, the call will return the JSON representation of that topology.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getPipelineTopology(GetPipelineTopologyArgs args) {
return getPipelineTopology(args, InvokeOptions.Empty);
}
/**
* Retrieves a specific pipeline topology by name. If a topology with that name has been previously created, the call will return the JSON representation of that topology.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getPipelineTopologyPlain(GetPipelineTopologyPlainArgs args) {
return getPipelineTopologyPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves a specific pipeline topology by name. If a topology with that name has been previously created, the call will return the JSON representation of that topology.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getPipelineTopology(GetPipelineTopologyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getPipelineTopology", TypeShape.of(GetPipelineTopologyResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a specific pipeline topology by name. If a topology with that name has been previously created, the call will return the JSON representation of that topology.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getPipelineTopologyPlain(GetPipelineTopologyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getPipelineTopology", TypeShape.of(GetPipelineTopologyResult.class), args, Utilities.withVersion(options));
}
/**
* Get private endpoint connection under video analyzer account.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Get private endpoint connection under video analyzer account.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Get private endpoint connection under video analyzer account.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get private endpoint connection under video analyzer account.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves an existing video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static Output getVideo(GetVideoArgs args) {
return getVideo(args, InvokeOptions.Empty);
}
/**
* Retrieves an existing video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static CompletableFuture getVideoPlain(GetVideoPlainArgs args) {
return getVideoPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves an existing video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static Output getVideo(GetVideoArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getVideo", TypeShape.of(GetVideoResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves an existing video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static CompletableFuture getVideoPlain(GetVideoPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getVideo", TypeShape.of(GetVideoResult.class), args, Utilities.withVersion(options));
}
/**
* Get the details of the specified Video Analyzer account
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static Output getVideoAnalyzer(GetVideoAnalyzerArgs args) {
return getVideoAnalyzer(args, InvokeOptions.Empty);
}
/**
* Get the details of the specified Video Analyzer account
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static CompletableFuture getVideoAnalyzerPlain(GetVideoAnalyzerPlainArgs args) {
return getVideoAnalyzerPlain(args, InvokeOptions.Empty);
}
/**
* Get the details of the specified Video Analyzer account
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static Output getVideoAnalyzer(GetVideoAnalyzerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:getVideoAnalyzer", TypeShape.of(GetVideoAnalyzerResult.class), args, Utilities.withVersion(options));
}
/**
* Get the details of the specified Video Analyzer account
* Azure REST API version: 2021-11-01-preview.
*
* Other available API versions: 2021-05-01-preview.
*
*/
public static CompletableFuture getVideoAnalyzerPlain(GetVideoAnalyzerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:getVideoAnalyzer", TypeShape.of(GetVideoAnalyzerResult.class), args, Utilities.withVersion(options));
}
/**
* Creates a new provisioning token. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output listEdgeModuleProvisioningToken(ListEdgeModuleProvisioningTokenArgs args) {
return listEdgeModuleProvisioningToken(args, InvokeOptions.Empty);
}
/**
* Creates a new provisioning token. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture listEdgeModuleProvisioningTokenPlain(ListEdgeModuleProvisioningTokenPlainArgs args) {
return listEdgeModuleProvisioningTokenPlain(args, InvokeOptions.Empty);
}
/**
* Creates a new provisioning token. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output listEdgeModuleProvisioningToken(ListEdgeModuleProvisioningTokenArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:listEdgeModuleProvisioningToken", TypeShape.of(ListEdgeModuleProvisioningTokenResult.class), args, Utilities.withVersion(options));
}
/**
* Creates a new provisioning token. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture listEdgeModuleProvisioningTokenPlain(ListEdgeModuleProvisioningTokenPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:listEdgeModuleProvisioningToken", TypeShape.of(ListEdgeModuleProvisioningTokenResult.class), args, Utilities.withVersion(options));
}
/**
* Generates a streaming token which can be used for accessing content from video content URLs, for a video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output listVideoContentToken(ListVideoContentTokenArgs args) {
return listVideoContentToken(args, InvokeOptions.Empty);
}
/**
* Generates a streaming token which can be used for accessing content from video content URLs, for a video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture listVideoContentTokenPlain(ListVideoContentTokenPlainArgs args) {
return listVideoContentTokenPlain(args, InvokeOptions.Empty);
}
/**
* Generates a streaming token which can be used for accessing content from video content URLs, for a video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static Output listVideoContentToken(ListVideoContentTokenArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:videoanalyzer:listVideoContentToken", TypeShape.of(ListVideoContentTokenResult.class), args, Utilities.withVersion(options));
}
/**
* Generates a streaming token which can be used for accessing content from video content URLs, for a video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
*
*/
public static CompletableFuture listVideoContentTokenPlain(ListVideoContentTokenPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:videoanalyzer:listVideoContentToken", TypeShape.of(ListVideoContentTokenResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy