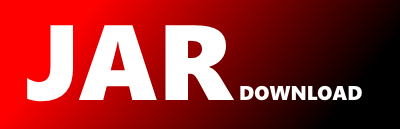
com.pulumi.azurenative.videoanalyzer.inputs.JwtAuthenticationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.inputs;
import com.pulumi.azurenative.videoanalyzer.inputs.EccTokenKeyArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.RsaTokenKeyArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.TokenClaimArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties for access validation based on JSON Web Tokens (JWT).
*
*/
public final class JwtAuthenticationArgs extends com.pulumi.resources.ResourceArgs {
public static final JwtAuthenticationArgs Empty = new JwtAuthenticationArgs();
/**
* List of expected token audiences. Token audience is valid if it matches at least one of the given values.
*
*/
@Import(name="audiences")
private @Nullable Output> audiences;
/**
* @return List of expected token audiences. Token audience is valid if it matches at least one of the given values.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy