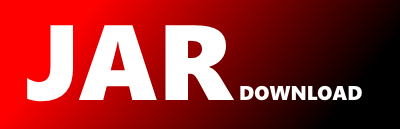
com.pulumi.azurenative.videoanalyzer.inputs.RtspSourceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.inputs;
import com.pulumi.azurenative.videoanalyzer.enums.RtspTransport;
import com.pulumi.azurenative.videoanalyzer.inputs.TlsEndpointArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.UnsecuredEndpointArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* RTSP source allows for media from an RTSP camera or generic RTSP server to be ingested into a pipeline.
*
*/
public final class RtspSourceArgs extends com.pulumi.resources.ResourceArgs {
public static final RtspSourceArgs Empty = new RtspSourceArgs();
/**
* RTSP endpoint information for Video Analyzer to connect to. This contains the required information for Video Analyzer to connect to RTSP cameras and/or generic RTSP servers.
*
*/
@Import(name="endpoint", required=true)
private Output> endpoint;
/**
* @return RTSP endpoint information for Video Analyzer to connect to. This contains the required information for Video Analyzer to connect to RTSP cameras and/or generic RTSP servers.
*
*/
public Output> endpoint() {
return this.endpoint;
}
/**
* Node name. Must be unique within the topology.
*
*/
@Import(name="name", required=true)
private Output name;
/**
* @return Node name. Must be unique within the topology.
*
*/
public Output name() {
return this.name;
}
/**
* Network transport utilized by the RTSP and RTP exchange: TCP or HTTP. When using TCP, the RTP packets are interleaved on the TCP RTSP connection. When using HTTP, the RTSP messages are exchanged through long lived HTTP connections, and the RTP packages are interleaved in the HTTP connections alongside the RTSP messages.
*
*/
@Import(name="transport")
private @Nullable Output> transport;
/**
* @return Network transport utilized by the RTSP and RTP exchange: TCP or HTTP. When using TCP, the RTP packets are interleaved on the TCP RTSP connection. When using HTTP, the RTSP messages are exchanged through long lived HTTP connections, and the RTP packages are interleaved in the HTTP connections alongside the RTSP messages.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy