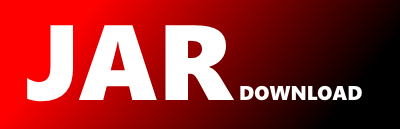
com.pulumi.azurenative.videoanalyzer.outputs.GetPipelineTopologyResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.outputs;
import com.pulumi.azurenative.videoanalyzer.outputs.EncoderProcessorResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.ParameterDeclarationResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.RtspSourceResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.SkuResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.SystemDataResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.VideoSinkResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.VideoSourceResponse;
import com.pulumi.core.Either;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPipelineTopologyResult {
/**
* @return An optional description of the pipeline topology. It is recommended that the expected use of the topology to be described here.
*
*/
private @Nullable String description;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Topology kind.
*
*/
private String kind;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return List of the topology parameter declarations. Parameters declared here can be referenced throughout the topology nodes through the use of "${PARAMETER_NAME}" string pattern. Parameters can have optional default values and can later be defined in individual instances of the pipeline.
*
*/
private @Nullable List parameters;
/**
* @return List of the topology processor nodes. Processor nodes enable pipeline data to be analyzed, processed or transformed.
*
*/
private @Nullable List processors;
/**
* @return List of the topology sink nodes. Sink nodes allow pipeline data to be stored or exported.
*
*/
private List sinks;
/**
* @return Describes the properties of a SKU.
*
*/
private SkuResponse sku;
/**
* @return List of the topology source nodes. Source nodes enable external data to be ingested by the pipeline.
*
*/
private List> sources;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetPipelineTopologyResult() {}
/**
* @return An optional description of the pipeline topology. It is recommended that the expected use of the topology to be described here.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Topology kind.
*
*/
public String kind() {
return this.kind;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return List of the topology parameter declarations. Parameters declared here can be referenced throughout the topology nodes through the use of "${PARAMETER_NAME}" string pattern. Parameters can have optional default values and can later be defined in individual instances of the pipeline.
*
*/
public List parameters() {
return this.parameters == null ? List.of() : this.parameters;
}
/**
* @return List of the topology processor nodes. Processor nodes enable pipeline data to be analyzed, processed or transformed.
*
*/
public List processors() {
return this.processors == null ? List.of() : this.processors;
}
/**
* @return List of the topology sink nodes. Sink nodes allow pipeline data to be stored or exported.
*
*/
public List sinks() {
return this.sinks;
}
/**
* @return Describes the properties of a SKU.
*
*/
public SkuResponse sku() {
return this.sku;
}
/**
* @return List of the topology source nodes. Source nodes enable external data to be ingested by the pipeline.
*
*/
public List> sources() {
return this.sources;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPipelineTopologyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String description;
private String id;
private String kind;
private String name;
private @Nullable List parameters;
private @Nullable List processors;
private List sinks;
private SkuResponse sku;
private List> sources;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetPipelineTopologyResult defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.id = defaults.id;
this.kind = defaults.kind;
this.name = defaults.name;
this.parameters = defaults.parameters;
this.processors = defaults.processors;
this.sinks = defaults.sinks;
this.sku = defaults.sku;
this.sources = defaults.sources;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder parameters(@Nullable List parameters) {
this.parameters = parameters;
return this;
}
public Builder parameters(ParameterDeclarationResponse... parameters) {
return parameters(List.of(parameters));
}
@CustomType.Setter
public Builder processors(@Nullable List processors) {
this.processors = processors;
return this;
}
public Builder processors(EncoderProcessorResponse... processors) {
return processors(List.of(processors));
}
@CustomType.Setter
public Builder sinks(List sinks) {
if (sinks == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "sinks");
}
this.sinks = sinks;
return this;
}
public Builder sinks(VideoSinkResponse... sinks) {
return sinks(List.of(sinks));
}
@CustomType.Setter
public Builder sku(SkuResponse sku) {
if (sku == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "sku");
}
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder sources(List> sources) {
if (sources == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "sources");
}
this.sources = sources;
return this;
}
public Builder sources(Either... sources) {
return sources(List.of(sources));
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetPipelineTopologyResult", "type");
}
this.type = type;
return this;
}
public GetPipelineTopologyResult build() {
final var _resultValue = new GetPipelineTopologyResult();
_resultValue.description = description;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.name = name;
_resultValue.parameters = parameters;
_resultValue.processors = processors;
_resultValue.sinks = sinks;
_resultValue.sku = sku;
_resultValue.sources = sources;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy