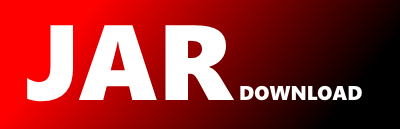
com.pulumi.azurenative.web.inputs.ContainerAppsConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ContainerAppsConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final ContainerAppsConfigurationArgs Empty = new ContainerAppsConfigurationArgs();
/**
* Resource ID of a subnet for control plane infrastructure components. This subnet must be in the same VNET as the subnet defined in appSubnetResourceId. Must not overlap with the IP range defined in platformReservedCidr, if defined.
*
*/
@Import(name="appSubnetResourceId")
private @Nullable Output appSubnetResourceId;
/**
* @return Resource ID of a subnet for control plane infrastructure components. This subnet must be in the same VNET as the subnet defined in appSubnetResourceId. Must not overlap with the IP range defined in platformReservedCidr, if defined.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy