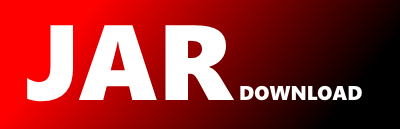
com.pulumi.azurenative.web.inputs.IdentityProvidersArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.inputs;
import com.pulumi.azurenative.web.inputs.AppleArgs;
import com.pulumi.azurenative.web.inputs.AzureActiveDirectoryArgs;
import com.pulumi.azurenative.web.inputs.AzureStaticWebAppsArgs;
import com.pulumi.azurenative.web.inputs.CustomOpenIdConnectProviderArgs;
import com.pulumi.azurenative.web.inputs.FacebookArgs;
import com.pulumi.azurenative.web.inputs.GitHubArgs;
import com.pulumi.azurenative.web.inputs.GoogleArgs;
import com.pulumi.azurenative.web.inputs.LegacyMicrosoftAccountArgs;
import com.pulumi.azurenative.web.inputs.TwitterArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The configuration settings of each of the identity providers used to configure App Service Authentication/Authorization.
*
*/
public final class IdentityProvidersArgs extends com.pulumi.resources.ResourceArgs {
public static final IdentityProvidersArgs Empty = new IdentityProvidersArgs();
/**
* The configuration settings of the Apple provider.
*
*/
@Import(name="apple")
private @Nullable Output apple;
/**
* @return The configuration settings of the Apple provider.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy