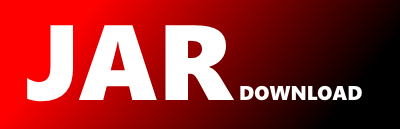
com.pulumi.azurenative.web.inputs.RampUpRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Routing rules for ramp up testing. This rule allows to redirect static traffic % to a slot or to gradually change routing % based on performance.
*
*/
public final class RampUpRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final RampUpRuleArgs Empty = new RampUpRuleArgs();
/**
* Hostname of a slot to which the traffic will be redirected if decided to. E.g. myapp-stage.azurewebsites.net.
*
*/
@Import(name="actionHostName")
private @Nullable Output actionHostName;
/**
* @return Hostname of a slot to which the traffic will be redirected if decided to. E.g. myapp-stage.azurewebsites.net.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy