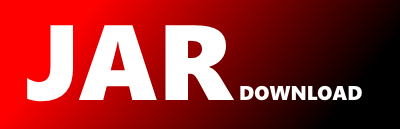
com.pulumi.azurenative.web.outputs.GetWebAppHostNameBindingResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWebAppHostNameBindingResult {
/**
* @return Azure resource name.
*
*/
private @Nullable String azureResourceName;
/**
* @return Azure resource type.
*
*/
private @Nullable String azureResourceType;
/**
* @return Custom DNS record type.
*
*/
private @Nullable String customHostNameDnsRecordType;
/**
* @return Fully qualified ARM domain resource URI.
*
*/
private @Nullable String domainId;
/**
* @return Hostname type.
*
*/
private @Nullable String hostNameType;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return Kind of resource.
*
*/
private @Nullable String kind;
/**
* @return Resource Name.
*
*/
private String name;
/**
* @return App Service app name.
*
*/
private @Nullable String siteName;
/**
* @return SSL type
*
*/
private @Nullable String sslState;
/**
* @return SSL certificate thumbprint
*
*/
private @Nullable String thumbprint;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return Virtual IP address assigned to the hostname if IP based SSL is enabled.
*
*/
private String virtualIP;
private GetWebAppHostNameBindingResult() {}
/**
* @return Azure resource name.
*
*/
public Optional azureResourceName() {
return Optional.ofNullable(this.azureResourceName);
}
/**
* @return Azure resource type.
*
*/
public Optional azureResourceType() {
return Optional.ofNullable(this.azureResourceType);
}
/**
* @return Custom DNS record type.
*
*/
public Optional customHostNameDnsRecordType() {
return Optional.ofNullable(this.customHostNameDnsRecordType);
}
/**
* @return Fully qualified ARM domain resource URI.
*
*/
public Optional domainId() {
return Optional.ofNullable(this.domainId);
}
/**
* @return Hostname type.
*
*/
public Optional hostNameType() {
return Optional.ofNullable(this.hostNameType);
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return Kind of resource.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return Resource Name.
*
*/
public String name() {
return this.name;
}
/**
* @return App Service app name.
*
*/
public Optional siteName() {
return Optional.ofNullable(this.siteName);
}
/**
* @return SSL type
*
*/
public Optional sslState() {
return Optional.ofNullable(this.sslState);
}
/**
* @return SSL certificate thumbprint
*
*/
public Optional thumbprint() {
return Optional.ofNullable(this.thumbprint);
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return Virtual IP address assigned to the hostname if IP based SSL is enabled.
*
*/
public String virtualIP() {
return this.virtualIP;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWebAppHostNameBindingResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String azureResourceName;
private @Nullable String azureResourceType;
private @Nullable String customHostNameDnsRecordType;
private @Nullable String domainId;
private @Nullable String hostNameType;
private String id;
private @Nullable String kind;
private String name;
private @Nullable String siteName;
private @Nullable String sslState;
private @Nullable String thumbprint;
private String type;
private String virtualIP;
public Builder() {}
public Builder(GetWebAppHostNameBindingResult defaults) {
Objects.requireNonNull(defaults);
this.azureResourceName = defaults.azureResourceName;
this.azureResourceType = defaults.azureResourceType;
this.customHostNameDnsRecordType = defaults.customHostNameDnsRecordType;
this.domainId = defaults.domainId;
this.hostNameType = defaults.hostNameType;
this.id = defaults.id;
this.kind = defaults.kind;
this.name = defaults.name;
this.siteName = defaults.siteName;
this.sslState = defaults.sslState;
this.thumbprint = defaults.thumbprint;
this.type = defaults.type;
this.virtualIP = defaults.virtualIP;
}
@CustomType.Setter
public Builder azureResourceName(@Nullable String azureResourceName) {
this.azureResourceName = azureResourceName;
return this;
}
@CustomType.Setter
public Builder azureResourceType(@Nullable String azureResourceType) {
this.azureResourceType = azureResourceType;
return this;
}
@CustomType.Setter
public Builder customHostNameDnsRecordType(@Nullable String customHostNameDnsRecordType) {
this.customHostNameDnsRecordType = customHostNameDnsRecordType;
return this;
}
@CustomType.Setter
public Builder domainId(@Nullable String domainId) {
this.domainId = domainId;
return this;
}
@CustomType.Setter
public Builder hostNameType(@Nullable String hostNameType) {
this.hostNameType = hostNameType;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWebAppHostNameBindingResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWebAppHostNameBindingResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder siteName(@Nullable String siteName) {
this.siteName = siteName;
return this;
}
@CustomType.Setter
public Builder sslState(@Nullable String sslState) {
this.sslState = sslState;
return this;
}
@CustomType.Setter
public Builder thumbprint(@Nullable String thumbprint) {
this.thumbprint = thumbprint;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWebAppHostNameBindingResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualIP(String virtualIP) {
if (virtualIP == null) {
throw new MissingRequiredPropertyException("GetWebAppHostNameBindingResult", "virtualIP");
}
this.virtualIP = virtualIP;
return this;
}
public GetWebAppHostNameBindingResult build() {
final var _resultValue = new GetWebAppHostNameBindingResult();
_resultValue.azureResourceName = azureResourceName;
_resultValue.azureResourceType = azureResourceType;
_resultValue.customHostNameDnsRecordType = customHostNameDnsRecordType;
_resultValue.domainId = domainId;
_resultValue.hostNameType = hostNameType;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.name = name;
_resultValue.siteName = siteName;
_resultValue.sslState = sslState;
_resultValue.thumbprint = thumbprint;
_resultValue.type = type;
_resultValue.virtualIP = virtualIP;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy