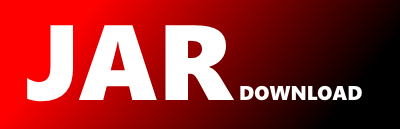
com.pulumi.azurenative.web.outputs.GetWebAppResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.outputs;
import com.pulumi.azurenative.web.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.web.outputs.HostNameSslStateResponse;
import com.pulumi.azurenative.web.outputs.HostingEnvironmentProfileResponse;
import com.pulumi.azurenative.web.outputs.ManagedServiceIdentityResponse;
import com.pulumi.azurenative.web.outputs.SiteConfigResponse;
import com.pulumi.azurenative.web.outputs.SlotSwapStatusResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWebAppResult {
/**
* @return Management information availability state for the app.
*
*/
private String availabilityState;
/**
* @return <code>true</code> to enable client affinity; <code>false</code> to stop sending session affinity cookies, which route client requests in the same session to the same instance. Default is <code>true</code>.
*
*/
private @Nullable Boolean clientAffinityEnabled;
/**
* @return <code>true</code> to enable client certificate authentication (TLS mutual authentication); otherwise, <code>false</code>. Default is <code>false</code>.
*
*/
private @Nullable Boolean clientCertEnabled;
/**
* @return client certificate authentication comma-separated exclusion paths
*
*/
private @Nullable String clientCertExclusionPaths;
/**
* @return This composes with ClientCertEnabled setting.
* - ClientCertEnabled: false means ClientCert is ignored.
* - ClientCertEnabled: true and ClientCertMode: Required means ClientCert is required.
* - ClientCertEnabled: true and ClientCertMode: Optional means ClientCert is optional or accepted.
*
*/
private @Nullable String clientCertMode;
/**
* @return Size of the function container.
*
*/
private @Nullable Integer containerSize;
/**
* @return Unique identifier that verifies the custom domains assigned to the app. Customer will add this id to a txt record for verification.
*
*/
private @Nullable String customDomainVerificationId;
/**
* @return Maximum allowed daily memory-time quota (applicable on dynamic apps only).
*
*/
private @Nullable Integer dailyMemoryTimeQuota;
/**
* @return Default hostname of the app. Read-only.
*
*/
private String defaultHostName;
/**
* @return <code>true</code> if the app is enabled; otherwise, <code>false</code>. Setting this value to false disables the app (takes the app offline).
*
*/
private @Nullable Boolean enabled;
/**
* @return Enabled hostnames for the app.Hostnames need to be assigned (see HostNames) AND enabled. Otherwise,
* the app is not served on those hostnames.
*
*/
private List enabledHostNames;
/**
* @return Extended Location.
*
*/
private @Nullable ExtendedLocationResponse extendedLocation;
/**
* @return Hostname SSL states are used to manage the SSL bindings for app's hostnames.
*
*/
private @Nullable List hostNameSslStates;
/**
* @return Hostnames associated with the app.
*
*/
private List hostNames;
/**
* @return <code>true</code> to disable the public hostnames of the app; otherwise, <code>false</code>.
* If <code>true</code>, the app is only accessible via API management process.
*
*/
private @Nullable Boolean hostNamesDisabled;
/**
* @return App Service Environment to use for the app.
*
*/
private @Nullable HostingEnvironmentProfileResponse hostingEnvironmentProfile;
/**
* @return HttpsOnly: configures a web site to accept only https requests. Issues redirect for
* http requests
*
*/
private @Nullable Boolean httpsOnly;
/**
* @return Hyper-V sandbox.
*
*/
private @Nullable Boolean hyperV;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return Managed service identity.
*
*/
private @Nullable ManagedServiceIdentityResponse identity;
/**
* @return Specifies an operation id if this site has a pending operation.
*
*/
private String inProgressOperationId;
/**
* @return <code>true</code> if the app is a default container; otherwise, <code>false</code>.
*
*/
private Boolean isDefaultContainer;
/**
* @return Obsolete: Hyper-V sandbox.
*
*/
private @Nullable Boolean isXenon;
/**
* @return Identity to use for Key Vault Reference authentication.
*
*/
private @Nullable String keyVaultReferenceIdentity;
/**
* @return Kind of resource.
*
*/
private @Nullable String kind;
/**
* @return Last time the app was modified, in UTC. Read-only.
*
*/
private String lastModifiedTimeUtc;
/**
* @return Resource Location.
*
*/
private String location;
/**
* @return Azure Resource Manager ID of the customer's selected Managed Environment on which to host this app. This must be of the form /subscriptions/{subscriptionId}/resourceGroups/{resourceGroup}/providers/Microsoft.App/managedEnvironments/{managedEnvironmentName}
*
*/
private @Nullable String managedEnvironmentId;
/**
* @return Maximum number of workers.
* This only applies to Functions container.
*
*/
private Integer maxNumberOfWorkers;
/**
* @return Resource Name.
*
*/
private String name;
/**
* @return List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from tenants that site can be hosted with current settings. Read-only.
*
*/
private String outboundIpAddresses;
/**
* @return List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from all tenants except dataComponent. Read-only.
*
*/
private String possibleOutboundIpAddresses;
/**
* @return Property to allow or block all public traffic. Allowed Values: 'Enabled', 'Disabled' or an empty string.
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Site redundancy mode
*
*/
private @Nullable String redundancyMode;
/**
* @return Name of the repository site.
*
*/
private String repositorySiteName;
/**
* @return <code>true</code> if reserved; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean reserved;
/**
* @return Name of the resource group the app belongs to. Read-only.
*
*/
private String resourceGroup;
/**
* @return <code>true</code> to stop SCM (KUDU) site when the app is stopped; otherwise, <code>false</code>. The default is <code>false</code>.
*
*/
private @Nullable Boolean scmSiteAlsoStopped;
/**
* @return Resource ID of the associated App Service plan, formatted as: "/subscriptions/{subscriptionID}/resourceGroups/{groupName}/providers/Microsoft.Web/serverfarms/{appServicePlanName}".
*
*/
private @Nullable String serverFarmId;
/**
* @return Configuration of the app.
*
*/
private @Nullable SiteConfigResponse siteConfig;
/**
* @return Status of the last deployment slot swap operation.
*
*/
private SlotSwapStatusResponse slotSwapStatus;
/**
* @return Current state of the app.
*
*/
private String state;
/**
* @return Checks if Customer provided storage account is required
*
*/
private @Nullable Boolean storageAccountRequired;
/**
* @return App suspended till in case memory-time quota is exceeded.
*
*/
private String suspendedTill;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Specifies which deployment slot this app will swap into. Read-only.
*
*/
private String targetSwapSlot;
/**
* @return Azure Traffic Manager hostnames associated with the app. Read-only.
*
*/
private List trafficManagerHostNames;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return State indicating whether the app has exceeded its quota usage. Read-only.
*
*/
private String usageState;
/**
* @return Azure Resource Manager ID of the Virtual network and subnet to be joined by Regional VNET Integration.
* This must be of the form /subscriptions/{subscriptionName}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{vnetName}/subnets/{subnetName}
*
*/
private @Nullable String virtualNetworkSubnetId;
/**
* @return To enable accessing content over virtual network
*
*/
private @Nullable Boolean vnetContentShareEnabled;
/**
* @return To enable pulling image over Virtual Network
*
*/
private @Nullable Boolean vnetImagePullEnabled;
/**
* @return Virtual Network Route All enabled. This causes all outbound traffic to have Virtual Network Security Groups and User Defined Routes applied.
*
*/
private @Nullable Boolean vnetRouteAllEnabled;
private GetWebAppResult() {}
/**
* @return Management information availability state for the app.
*
*/
public String availabilityState() {
return this.availabilityState;
}
/**
* @return <code>true</code> to enable client affinity; <code>false</code> to stop sending session affinity cookies, which route client requests in the same session to the same instance. Default is <code>true</code>.
*
*/
public Optional clientAffinityEnabled() {
return Optional.ofNullable(this.clientAffinityEnabled);
}
/**
* @return <code>true</code> to enable client certificate authentication (TLS mutual authentication); otherwise, <code>false</code>. Default is <code>false</code>.
*
*/
public Optional clientCertEnabled() {
return Optional.ofNullable(this.clientCertEnabled);
}
/**
* @return client certificate authentication comma-separated exclusion paths
*
*/
public Optional clientCertExclusionPaths() {
return Optional.ofNullable(this.clientCertExclusionPaths);
}
/**
* @return This composes with ClientCertEnabled setting.
* - ClientCertEnabled: false means ClientCert is ignored.
* - ClientCertEnabled: true and ClientCertMode: Required means ClientCert is required.
* - ClientCertEnabled: true and ClientCertMode: Optional means ClientCert is optional or accepted.
*
*/
public Optional clientCertMode() {
return Optional.ofNullable(this.clientCertMode);
}
/**
* @return Size of the function container.
*
*/
public Optional containerSize() {
return Optional.ofNullable(this.containerSize);
}
/**
* @return Unique identifier that verifies the custom domains assigned to the app. Customer will add this id to a txt record for verification.
*
*/
public Optional customDomainVerificationId() {
return Optional.ofNullable(this.customDomainVerificationId);
}
/**
* @return Maximum allowed daily memory-time quota (applicable on dynamic apps only).
*
*/
public Optional dailyMemoryTimeQuota() {
return Optional.ofNullable(this.dailyMemoryTimeQuota);
}
/**
* @return Default hostname of the app. Read-only.
*
*/
public String defaultHostName() {
return this.defaultHostName;
}
/**
* @return <code>true</code> if the app is enabled; otherwise, <code>false</code>. Setting this value to false disables the app (takes the app offline).
*
*/
public Optional enabled() {
return Optional.ofNullable(this.enabled);
}
/**
* @return Enabled hostnames for the app.Hostnames need to be assigned (see HostNames) AND enabled. Otherwise,
* the app is not served on those hostnames.
*
*/
public List enabledHostNames() {
return this.enabledHostNames;
}
/**
* @return Extended Location.
*
*/
public Optional extendedLocation() {
return Optional.ofNullable(this.extendedLocation);
}
/**
* @return Hostname SSL states are used to manage the SSL bindings for app's hostnames.
*
*/
public List hostNameSslStates() {
return this.hostNameSslStates == null ? List.of() : this.hostNameSslStates;
}
/**
* @return Hostnames associated with the app.
*
*/
public List hostNames() {
return this.hostNames;
}
/**
* @return <code>true</code> to disable the public hostnames of the app; otherwise, <code>false</code>.
* If <code>true</code>, the app is only accessible via API management process.
*
*/
public Optional hostNamesDisabled() {
return Optional.ofNullable(this.hostNamesDisabled);
}
/**
* @return App Service Environment to use for the app.
*
*/
public Optional hostingEnvironmentProfile() {
return Optional.ofNullable(this.hostingEnvironmentProfile);
}
/**
* @return HttpsOnly: configures a web site to accept only https requests. Issues redirect for
* http requests
*
*/
public Optional httpsOnly() {
return Optional.ofNullable(this.httpsOnly);
}
/**
* @return Hyper-V sandbox.
*
*/
public Optional hyperV() {
return Optional.ofNullable(this.hyperV);
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return Managed service identity.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return Specifies an operation id if this site has a pending operation.
*
*/
public String inProgressOperationId() {
return this.inProgressOperationId;
}
/**
* @return <code>true</code> if the app is a default container; otherwise, <code>false</code>.
*
*/
public Boolean isDefaultContainer() {
return this.isDefaultContainer;
}
/**
* @return Obsolete: Hyper-V sandbox.
*
*/
public Optional isXenon() {
return Optional.ofNullable(this.isXenon);
}
/**
* @return Identity to use for Key Vault Reference authentication.
*
*/
public Optional keyVaultReferenceIdentity() {
return Optional.ofNullable(this.keyVaultReferenceIdentity);
}
/**
* @return Kind of resource.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return Last time the app was modified, in UTC. Read-only.
*
*/
public String lastModifiedTimeUtc() {
return this.lastModifiedTimeUtc;
}
/**
* @return Resource Location.
*
*/
public String location() {
return this.location;
}
/**
* @return Azure Resource Manager ID of the customer's selected Managed Environment on which to host this app. This must be of the form /subscriptions/{subscriptionId}/resourceGroups/{resourceGroup}/providers/Microsoft.App/managedEnvironments/{managedEnvironmentName}
*
*/
public Optional managedEnvironmentId() {
return Optional.ofNullable(this.managedEnvironmentId);
}
/**
* @return Maximum number of workers.
* This only applies to Functions container.
*
*/
public Integer maxNumberOfWorkers() {
return this.maxNumberOfWorkers;
}
/**
* @return Resource Name.
*
*/
public String name() {
return this.name;
}
/**
* @return List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from tenants that site can be hosted with current settings. Read-only.
*
*/
public String outboundIpAddresses() {
return this.outboundIpAddresses;
}
/**
* @return List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from all tenants except dataComponent. Read-only.
*
*/
public String possibleOutboundIpAddresses() {
return this.possibleOutboundIpAddresses;
}
/**
* @return Property to allow or block all public traffic. Allowed Values: 'Enabled', 'Disabled' or an empty string.
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Site redundancy mode
*
*/
public Optional redundancyMode() {
return Optional.ofNullable(this.redundancyMode);
}
/**
* @return Name of the repository site.
*
*/
public String repositorySiteName() {
return this.repositorySiteName;
}
/**
* @return <code>true</code> if reserved; otherwise, <code>false</code>.
*
*/
public Optional reserved() {
return Optional.ofNullable(this.reserved);
}
/**
* @return Name of the resource group the app belongs to. Read-only.
*
*/
public String resourceGroup() {
return this.resourceGroup;
}
/**
* @return <code>true</code> to stop SCM (KUDU) site when the app is stopped; otherwise, <code>false</code>. The default is <code>false</code>.
*
*/
public Optional scmSiteAlsoStopped() {
return Optional.ofNullable(this.scmSiteAlsoStopped);
}
/**
* @return Resource ID of the associated App Service plan, formatted as: "/subscriptions/{subscriptionID}/resourceGroups/{groupName}/providers/Microsoft.Web/serverfarms/{appServicePlanName}".
*
*/
public Optional serverFarmId() {
return Optional.ofNullable(this.serverFarmId);
}
/**
* @return Configuration of the app.
*
*/
public Optional siteConfig() {
return Optional.ofNullable(this.siteConfig);
}
/**
* @return Status of the last deployment slot swap operation.
*
*/
public SlotSwapStatusResponse slotSwapStatus() {
return this.slotSwapStatus;
}
/**
* @return Current state of the app.
*
*/
public String state() {
return this.state;
}
/**
* @return Checks if Customer provided storage account is required
*
*/
public Optional storageAccountRequired() {
return Optional.ofNullable(this.storageAccountRequired);
}
/**
* @return App suspended till in case memory-time quota is exceeded.
*
*/
public String suspendedTill() {
return this.suspendedTill;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Specifies which deployment slot this app will swap into. Read-only.
*
*/
public String targetSwapSlot() {
return this.targetSwapSlot;
}
/**
* @return Azure Traffic Manager hostnames associated with the app. Read-only.
*
*/
public List trafficManagerHostNames() {
return this.trafficManagerHostNames;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return State indicating whether the app has exceeded its quota usage. Read-only.
*
*/
public String usageState() {
return this.usageState;
}
/**
* @return Azure Resource Manager ID of the Virtual network and subnet to be joined by Regional VNET Integration.
* This must be of the form /subscriptions/{subscriptionName}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{vnetName}/subnets/{subnetName}
*
*/
public Optional virtualNetworkSubnetId() {
return Optional.ofNullable(this.virtualNetworkSubnetId);
}
/**
* @return To enable accessing content over virtual network
*
*/
public Optional vnetContentShareEnabled() {
return Optional.ofNullable(this.vnetContentShareEnabled);
}
/**
* @return To enable pulling image over Virtual Network
*
*/
public Optional vnetImagePullEnabled() {
return Optional.ofNullable(this.vnetImagePullEnabled);
}
/**
* @return Virtual Network Route All enabled. This causes all outbound traffic to have Virtual Network Security Groups and User Defined Routes applied.
*
*/
public Optional vnetRouteAllEnabled() {
return Optional.ofNullable(this.vnetRouteAllEnabled);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWebAppResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String availabilityState;
private @Nullable Boolean clientAffinityEnabled;
private @Nullable Boolean clientCertEnabled;
private @Nullable String clientCertExclusionPaths;
private @Nullable String clientCertMode;
private @Nullable Integer containerSize;
private @Nullable String customDomainVerificationId;
private @Nullable Integer dailyMemoryTimeQuota;
private String defaultHostName;
private @Nullable Boolean enabled;
private List enabledHostNames;
private @Nullable ExtendedLocationResponse extendedLocation;
private @Nullable List hostNameSslStates;
private List hostNames;
private @Nullable Boolean hostNamesDisabled;
private @Nullable HostingEnvironmentProfileResponse hostingEnvironmentProfile;
private @Nullable Boolean httpsOnly;
private @Nullable Boolean hyperV;
private String id;
private @Nullable ManagedServiceIdentityResponse identity;
private String inProgressOperationId;
private Boolean isDefaultContainer;
private @Nullable Boolean isXenon;
private @Nullable String keyVaultReferenceIdentity;
private @Nullable String kind;
private String lastModifiedTimeUtc;
private String location;
private @Nullable String managedEnvironmentId;
private Integer maxNumberOfWorkers;
private String name;
private String outboundIpAddresses;
private String possibleOutboundIpAddresses;
private @Nullable String publicNetworkAccess;
private @Nullable String redundancyMode;
private String repositorySiteName;
private @Nullable Boolean reserved;
private String resourceGroup;
private @Nullable Boolean scmSiteAlsoStopped;
private @Nullable String serverFarmId;
private @Nullable SiteConfigResponse siteConfig;
private SlotSwapStatusResponse slotSwapStatus;
private String state;
private @Nullable Boolean storageAccountRequired;
private String suspendedTill;
private @Nullable Map tags;
private String targetSwapSlot;
private List trafficManagerHostNames;
private String type;
private String usageState;
private @Nullable String virtualNetworkSubnetId;
private @Nullable Boolean vnetContentShareEnabled;
private @Nullable Boolean vnetImagePullEnabled;
private @Nullable Boolean vnetRouteAllEnabled;
public Builder() {}
public Builder(GetWebAppResult defaults) {
Objects.requireNonNull(defaults);
this.availabilityState = defaults.availabilityState;
this.clientAffinityEnabled = defaults.clientAffinityEnabled;
this.clientCertEnabled = defaults.clientCertEnabled;
this.clientCertExclusionPaths = defaults.clientCertExclusionPaths;
this.clientCertMode = defaults.clientCertMode;
this.containerSize = defaults.containerSize;
this.customDomainVerificationId = defaults.customDomainVerificationId;
this.dailyMemoryTimeQuota = defaults.dailyMemoryTimeQuota;
this.defaultHostName = defaults.defaultHostName;
this.enabled = defaults.enabled;
this.enabledHostNames = defaults.enabledHostNames;
this.extendedLocation = defaults.extendedLocation;
this.hostNameSslStates = defaults.hostNameSslStates;
this.hostNames = defaults.hostNames;
this.hostNamesDisabled = defaults.hostNamesDisabled;
this.hostingEnvironmentProfile = defaults.hostingEnvironmentProfile;
this.httpsOnly = defaults.httpsOnly;
this.hyperV = defaults.hyperV;
this.id = defaults.id;
this.identity = defaults.identity;
this.inProgressOperationId = defaults.inProgressOperationId;
this.isDefaultContainer = defaults.isDefaultContainer;
this.isXenon = defaults.isXenon;
this.keyVaultReferenceIdentity = defaults.keyVaultReferenceIdentity;
this.kind = defaults.kind;
this.lastModifiedTimeUtc = defaults.lastModifiedTimeUtc;
this.location = defaults.location;
this.managedEnvironmentId = defaults.managedEnvironmentId;
this.maxNumberOfWorkers = defaults.maxNumberOfWorkers;
this.name = defaults.name;
this.outboundIpAddresses = defaults.outboundIpAddresses;
this.possibleOutboundIpAddresses = defaults.possibleOutboundIpAddresses;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.redundancyMode = defaults.redundancyMode;
this.repositorySiteName = defaults.repositorySiteName;
this.reserved = defaults.reserved;
this.resourceGroup = defaults.resourceGroup;
this.scmSiteAlsoStopped = defaults.scmSiteAlsoStopped;
this.serverFarmId = defaults.serverFarmId;
this.siteConfig = defaults.siteConfig;
this.slotSwapStatus = defaults.slotSwapStatus;
this.state = defaults.state;
this.storageAccountRequired = defaults.storageAccountRequired;
this.suspendedTill = defaults.suspendedTill;
this.tags = defaults.tags;
this.targetSwapSlot = defaults.targetSwapSlot;
this.trafficManagerHostNames = defaults.trafficManagerHostNames;
this.type = defaults.type;
this.usageState = defaults.usageState;
this.virtualNetworkSubnetId = defaults.virtualNetworkSubnetId;
this.vnetContentShareEnabled = defaults.vnetContentShareEnabled;
this.vnetImagePullEnabled = defaults.vnetImagePullEnabled;
this.vnetRouteAllEnabled = defaults.vnetRouteAllEnabled;
}
@CustomType.Setter
public Builder availabilityState(String availabilityState) {
if (availabilityState == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "availabilityState");
}
this.availabilityState = availabilityState;
return this;
}
@CustomType.Setter
public Builder clientAffinityEnabled(@Nullable Boolean clientAffinityEnabled) {
this.clientAffinityEnabled = clientAffinityEnabled;
return this;
}
@CustomType.Setter
public Builder clientCertEnabled(@Nullable Boolean clientCertEnabled) {
this.clientCertEnabled = clientCertEnabled;
return this;
}
@CustomType.Setter
public Builder clientCertExclusionPaths(@Nullable String clientCertExclusionPaths) {
this.clientCertExclusionPaths = clientCertExclusionPaths;
return this;
}
@CustomType.Setter
public Builder clientCertMode(@Nullable String clientCertMode) {
this.clientCertMode = clientCertMode;
return this;
}
@CustomType.Setter
public Builder containerSize(@Nullable Integer containerSize) {
this.containerSize = containerSize;
return this;
}
@CustomType.Setter
public Builder customDomainVerificationId(@Nullable String customDomainVerificationId) {
this.customDomainVerificationId = customDomainVerificationId;
return this;
}
@CustomType.Setter
public Builder dailyMemoryTimeQuota(@Nullable Integer dailyMemoryTimeQuota) {
this.dailyMemoryTimeQuota = dailyMemoryTimeQuota;
return this;
}
@CustomType.Setter
public Builder defaultHostName(String defaultHostName) {
if (defaultHostName == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "defaultHostName");
}
this.defaultHostName = defaultHostName;
return this;
}
@CustomType.Setter
public Builder enabled(@Nullable Boolean enabled) {
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder enabledHostNames(List enabledHostNames) {
if (enabledHostNames == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "enabledHostNames");
}
this.enabledHostNames = enabledHostNames;
return this;
}
public Builder enabledHostNames(String... enabledHostNames) {
return enabledHostNames(List.of(enabledHostNames));
}
@CustomType.Setter
public Builder extendedLocation(@Nullable ExtendedLocationResponse extendedLocation) {
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder hostNameSslStates(@Nullable List hostNameSslStates) {
this.hostNameSslStates = hostNameSslStates;
return this;
}
public Builder hostNameSslStates(HostNameSslStateResponse... hostNameSslStates) {
return hostNameSslStates(List.of(hostNameSslStates));
}
@CustomType.Setter
public Builder hostNames(List hostNames) {
if (hostNames == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "hostNames");
}
this.hostNames = hostNames;
return this;
}
public Builder hostNames(String... hostNames) {
return hostNames(List.of(hostNames));
}
@CustomType.Setter
public Builder hostNamesDisabled(@Nullable Boolean hostNamesDisabled) {
this.hostNamesDisabled = hostNamesDisabled;
return this;
}
@CustomType.Setter
public Builder hostingEnvironmentProfile(@Nullable HostingEnvironmentProfileResponse hostingEnvironmentProfile) {
this.hostingEnvironmentProfile = hostingEnvironmentProfile;
return this;
}
@CustomType.Setter
public Builder httpsOnly(@Nullable Boolean httpsOnly) {
this.httpsOnly = httpsOnly;
return this;
}
@CustomType.Setter
public Builder hyperV(@Nullable Boolean hyperV) {
this.hyperV = hyperV;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable ManagedServiceIdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder inProgressOperationId(String inProgressOperationId) {
if (inProgressOperationId == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "inProgressOperationId");
}
this.inProgressOperationId = inProgressOperationId;
return this;
}
@CustomType.Setter
public Builder isDefaultContainer(Boolean isDefaultContainer) {
if (isDefaultContainer == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "isDefaultContainer");
}
this.isDefaultContainer = isDefaultContainer;
return this;
}
@CustomType.Setter
public Builder isXenon(@Nullable Boolean isXenon) {
this.isXenon = isXenon;
return this;
}
@CustomType.Setter
public Builder keyVaultReferenceIdentity(@Nullable String keyVaultReferenceIdentity) {
this.keyVaultReferenceIdentity = keyVaultReferenceIdentity;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder lastModifiedTimeUtc(String lastModifiedTimeUtc) {
if (lastModifiedTimeUtc == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "lastModifiedTimeUtc");
}
this.lastModifiedTimeUtc = lastModifiedTimeUtc;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedEnvironmentId(@Nullable String managedEnvironmentId) {
this.managedEnvironmentId = managedEnvironmentId;
return this;
}
@CustomType.Setter
public Builder maxNumberOfWorkers(Integer maxNumberOfWorkers) {
if (maxNumberOfWorkers == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "maxNumberOfWorkers");
}
this.maxNumberOfWorkers = maxNumberOfWorkers;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder outboundIpAddresses(String outboundIpAddresses) {
if (outboundIpAddresses == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "outboundIpAddresses");
}
this.outboundIpAddresses = outboundIpAddresses;
return this;
}
@CustomType.Setter
public Builder possibleOutboundIpAddresses(String possibleOutboundIpAddresses) {
if (possibleOutboundIpAddresses == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "possibleOutboundIpAddresses");
}
this.possibleOutboundIpAddresses = possibleOutboundIpAddresses;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder redundancyMode(@Nullable String redundancyMode) {
this.redundancyMode = redundancyMode;
return this;
}
@CustomType.Setter
public Builder repositorySiteName(String repositorySiteName) {
if (repositorySiteName == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "repositorySiteName");
}
this.repositorySiteName = repositorySiteName;
return this;
}
@CustomType.Setter
public Builder reserved(@Nullable Boolean reserved) {
this.reserved = reserved;
return this;
}
@CustomType.Setter
public Builder resourceGroup(String resourceGroup) {
if (resourceGroup == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "resourceGroup");
}
this.resourceGroup = resourceGroup;
return this;
}
@CustomType.Setter
public Builder scmSiteAlsoStopped(@Nullable Boolean scmSiteAlsoStopped) {
this.scmSiteAlsoStopped = scmSiteAlsoStopped;
return this;
}
@CustomType.Setter
public Builder serverFarmId(@Nullable String serverFarmId) {
this.serverFarmId = serverFarmId;
return this;
}
@CustomType.Setter
public Builder siteConfig(@Nullable SiteConfigResponse siteConfig) {
this.siteConfig = siteConfig;
return this;
}
@CustomType.Setter
public Builder slotSwapStatus(SlotSwapStatusResponse slotSwapStatus) {
if (slotSwapStatus == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "slotSwapStatus");
}
this.slotSwapStatus = slotSwapStatus;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder storageAccountRequired(@Nullable Boolean storageAccountRequired) {
this.storageAccountRequired = storageAccountRequired;
return this;
}
@CustomType.Setter
public Builder suspendedTill(String suspendedTill) {
if (suspendedTill == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "suspendedTill");
}
this.suspendedTill = suspendedTill;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder targetSwapSlot(String targetSwapSlot) {
if (targetSwapSlot == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "targetSwapSlot");
}
this.targetSwapSlot = targetSwapSlot;
return this;
}
@CustomType.Setter
public Builder trafficManagerHostNames(List trafficManagerHostNames) {
if (trafficManagerHostNames == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "trafficManagerHostNames");
}
this.trafficManagerHostNames = trafficManagerHostNames;
return this;
}
public Builder trafficManagerHostNames(String... trafficManagerHostNames) {
return trafficManagerHostNames(List.of(trafficManagerHostNames));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder usageState(String usageState) {
if (usageState == null) {
throw new MissingRequiredPropertyException("GetWebAppResult", "usageState");
}
this.usageState = usageState;
return this;
}
@CustomType.Setter
public Builder virtualNetworkSubnetId(@Nullable String virtualNetworkSubnetId) {
this.virtualNetworkSubnetId = virtualNetworkSubnetId;
return this;
}
@CustomType.Setter
public Builder vnetContentShareEnabled(@Nullable Boolean vnetContentShareEnabled) {
this.vnetContentShareEnabled = vnetContentShareEnabled;
return this;
}
@CustomType.Setter
public Builder vnetImagePullEnabled(@Nullable Boolean vnetImagePullEnabled) {
this.vnetImagePullEnabled = vnetImagePullEnabled;
return this;
}
@CustomType.Setter
public Builder vnetRouteAllEnabled(@Nullable Boolean vnetRouteAllEnabled) {
this.vnetRouteAllEnabled = vnetRouteAllEnabled;
return this;
}
public GetWebAppResult build() {
final var _resultValue = new GetWebAppResult();
_resultValue.availabilityState = availabilityState;
_resultValue.clientAffinityEnabled = clientAffinityEnabled;
_resultValue.clientCertEnabled = clientCertEnabled;
_resultValue.clientCertExclusionPaths = clientCertExclusionPaths;
_resultValue.clientCertMode = clientCertMode;
_resultValue.containerSize = containerSize;
_resultValue.customDomainVerificationId = customDomainVerificationId;
_resultValue.dailyMemoryTimeQuota = dailyMemoryTimeQuota;
_resultValue.defaultHostName = defaultHostName;
_resultValue.enabled = enabled;
_resultValue.enabledHostNames = enabledHostNames;
_resultValue.extendedLocation = extendedLocation;
_resultValue.hostNameSslStates = hostNameSslStates;
_resultValue.hostNames = hostNames;
_resultValue.hostNamesDisabled = hostNamesDisabled;
_resultValue.hostingEnvironmentProfile = hostingEnvironmentProfile;
_resultValue.httpsOnly = httpsOnly;
_resultValue.hyperV = hyperV;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.inProgressOperationId = inProgressOperationId;
_resultValue.isDefaultContainer = isDefaultContainer;
_resultValue.isXenon = isXenon;
_resultValue.keyVaultReferenceIdentity = keyVaultReferenceIdentity;
_resultValue.kind = kind;
_resultValue.lastModifiedTimeUtc = lastModifiedTimeUtc;
_resultValue.location = location;
_resultValue.managedEnvironmentId = managedEnvironmentId;
_resultValue.maxNumberOfWorkers = maxNumberOfWorkers;
_resultValue.name = name;
_resultValue.outboundIpAddresses = outboundIpAddresses;
_resultValue.possibleOutboundIpAddresses = possibleOutboundIpAddresses;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.redundancyMode = redundancyMode;
_resultValue.repositorySiteName = repositorySiteName;
_resultValue.reserved = reserved;
_resultValue.resourceGroup = resourceGroup;
_resultValue.scmSiteAlsoStopped = scmSiteAlsoStopped;
_resultValue.serverFarmId = serverFarmId;
_resultValue.siteConfig = siteConfig;
_resultValue.slotSwapStatus = slotSwapStatus;
_resultValue.state = state;
_resultValue.storageAccountRequired = storageAccountRequired;
_resultValue.suspendedTill = suspendedTill;
_resultValue.tags = tags;
_resultValue.targetSwapSlot = targetSwapSlot;
_resultValue.trafficManagerHostNames = trafficManagerHostNames;
_resultValue.type = type;
_resultValue.usageState = usageState;
_resultValue.virtualNetworkSubnetId = virtualNetworkSubnetId;
_resultValue.vnetContentShareEnabled = vnetContentShareEnabled;
_resultValue.vnetImagePullEnabled = vnetImagePullEnabled;
_resultValue.vnetRouteAllEnabled = vnetRouteAllEnabled;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy