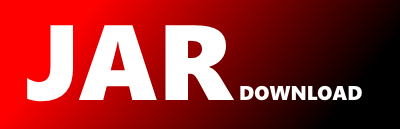
com.pulumi.azurenative.web.outputs.ListWebAppAuthSettingsResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ListWebAppAuthSettingsResult {
/**
* @return Gets a JSON string containing the Azure AD Acl settings.
*
*/
private @Nullable String aadClaimsAuthorization;
/**
* @return Login parameters to send to the OpenID Connect authorization endpoint when
* a user logs in. Each parameter must be in the form "key=value".
*
*/
private @Nullable List additionalLoginParams;
/**
* @return Allowed audience values to consider when validating JSON Web Tokens issued by
* Azure Active Directory. Note that the <code>ClientID</code> value is always considered an
* allowed audience, regardless of this setting.
*
*/
private @Nullable List allowedAudiences;
/**
* @return External URLs that can be redirected to as part of logging in or logging out of the app. Note that the query string part of the URL is ignored.
* This is an advanced setting typically only needed by Windows Store application backends.
* Note that URLs within the current domain are always implicitly allowed.
*
*/
private @Nullable List allowedExternalRedirectUrls;
/**
* @return The path of the config file containing auth settings.
* If the path is relative, base will the site's root directory.
*
*/
private @Nullable String authFilePath;
/**
* @return The Client ID of this relying party application, known as the client_id.
* This setting is required for enabling OpenID Connection authentication with Azure Active Directory or
* other 3rd party OpenID Connect providers.
* More information on OpenID Connect: http://openid.net/specs/openid-connect-core-1_0.html
*
*/
private @Nullable String clientId;
/**
* @return The Client Secret of this relying party application (in Azure Active Directory, this is also referred to as the Key).
* This setting is optional. If no client secret is configured, the OpenID Connect implicit auth flow is used to authenticate end users.
* Otherwise, the OpenID Connect Authorization Code Flow is used to authenticate end users.
* More information on OpenID Connect: http://openid.net/specs/openid-connect-core-1_0.html
*
*/
private @Nullable String clientSecret;
/**
* @return An alternative to the client secret, that is the thumbprint of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret. It is also optional.
*
*/
private @Nullable String clientSecretCertificateThumbprint;
/**
* @return The app setting name that contains the client secret of the relying party application.
*
*/
private @Nullable String clientSecretSettingName;
/**
* @return The ConfigVersion of the Authentication / Authorization feature in use for the current app.
* The setting in this value can control the behavior of the control plane for Authentication / Authorization.
*
*/
private @Nullable String configVersion;
/**
* @return The default authentication provider to use when multiple providers are configured.
* This setting is only needed if multiple providers are configured and the unauthenticated client
* action is set to "RedirectToLoginPage".
*
*/
private @Nullable String defaultProvider;
/**
* @return <code>true</code> if the Authentication / Authorization feature is enabled for the current app; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean enabled;
/**
* @return The App ID of the Facebook app used for login.
* This setting is required for enabling Facebook Login.
* Facebook Login documentation: https://developers.facebook.com/docs/facebook-login
*
*/
private @Nullable String facebookAppId;
/**
* @return The App Secret of the Facebook app used for Facebook Login.
* This setting is required for enabling Facebook Login.
* Facebook Login documentation: https://developers.facebook.com/docs/facebook-login
*
*/
private @Nullable String facebookAppSecret;
/**
* @return The app setting name that contains the app secret used for Facebook Login.
*
*/
private @Nullable String facebookAppSecretSettingName;
/**
* @return The OAuth 2.0 scopes that will be requested as part of Facebook Login authentication.
* This setting is optional.
* Facebook Login documentation: https://developers.facebook.com/docs/facebook-login
*
*/
private @Nullable List facebookOAuthScopes;
/**
* @return The Client Id of the GitHub app used for login.
* This setting is required for enabling Github login
*
*/
private @Nullable String gitHubClientId;
/**
* @return The Client Secret of the GitHub app used for Github Login.
* This setting is required for enabling Github login.
*
*/
private @Nullable String gitHubClientSecret;
/**
* @return The app setting name that contains the client secret of the Github
* app used for GitHub Login.
*
*/
private @Nullable String gitHubClientSecretSettingName;
/**
* @return The OAuth 2.0 scopes that will be requested as part of GitHub Login authentication.
* This setting is optional
*
*/
private @Nullable List gitHubOAuthScopes;
/**
* @return The OpenID Connect Client ID for the Google web application.
* This setting is required for enabling Google Sign-In.
* Google Sign-In documentation: https://developers.google.com/identity/sign-in/web/
*
*/
private @Nullable String googleClientId;
/**
* @return The client secret associated with the Google web application.
* This setting is required for enabling Google Sign-In.
* Google Sign-In documentation: https://developers.google.com/identity/sign-in/web/
*
*/
private @Nullable String googleClientSecret;
/**
* @return The app setting name that contains the client secret associated with
* the Google web application.
*
*/
private @Nullable String googleClientSecretSettingName;
/**
* @return The OAuth 2.0 scopes that will be requested as part of Google Sign-In authentication.
* This setting is optional. If not specified, "openid", "profile", and "email" are used as default scopes.
* Google Sign-In documentation: https://developers.google.com/identity/sign-in/web/
*
*/
private @Nullable List googleOAuthScopes;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return "true" if the auth config settings should be read from a file,
* "false" otherwise
*
*/
private @Nullable String isAuthFromFile;
/**
* @return The OpenID Connect Issuer URI that represents the entity which issues access tokens for this application.
* When using Azure Active Directory, this value is the URI of the directory tenant, e.g. https://sts.windows.net/{tenant-guid}/.
* This URI is a case-sensitive identifier for the token issuer.
* More information on OpenID Connect Discovery: http://openid.net/specs/openid-connect-discovery-1_0.html
*
*/
private @Nullable String issuer;
/**
* @return Kind of resource.
*
*/
private @Nullable String kind;
/**
* @return The OAuth 2.0 client ID that was created for the app used for authentication.
* This setting is required for enabling Microsoft Account authentication.
* Microsoft Account OAuth documentation: https://dev.onedrive.com/auth/msa_oauth.htm
*
*/
private @Nullable String microsoftAccountClientId;
/**
* @return The OAuth 2.0 client secret that was created for the app used for authentication.
* This setting is required for enabling Microsoft Account authentication.
* Microsoft Account OAuth documentation: https://dev.onedrive.com/auth/msa_oauth.htm
*
*/
private @Nullable String microsoftAccountClientSecret;
/**
* @return The app setting name containing the OAuth 2.0 client secret that was created for the
* app used for authentication.
*
*/
private @Nullable String microsoftAccountClientSecretSettingName;
/**
* @return The OAuth 2.0 scopes that will be requested as part of Microsoft Account authentication.
* This setting is optional. If not specified, "wl.basic" is used as the default scope.
* Microsoft Account Scopes and permissions documentation: https://msdn.microsoft.com/en-us/library/dn631845.aspx
*
*/
private @Nullable List microsoftAccountOAuthScopes;
/**
* @return Resource Name.
*
*/
private String name;
/**
* @return The RuntimeVersion of the Authentication / Authorization feature in use for the current app.
* The setting in this value can control the behavior of certain features in the Authentication / Authorization module.
*
*/
private @Nullable String runtimeVersion;
/**
* @return The number of hours after session token expiration that a session token can be used to
* call the token refresh API. The default is 72 hours.
*
*/
private @Nullable Double tokenRefreshExtensionHours;
/**
* @return <code>true</code> to durably store platform-specific security tokens that are obtained during login flows; otherwise, <code>false</code>.
* The default is <code>false</code>.
*
*/
private @Nullable Boolean tokenStoreEnabled;
/**
* @return The OAuth 1.0a consumer key of the Twitter application used for sign-in.
* This setting is required for enabling Twitter Sign-In.
* Twitter Sign-In documentation: https://dev.twitter.com/web/sign-in
*
*/
private @Nullable String twitterConsumerKey;
/**
* @return The OAuth 1.0a consumer secret of the Twitter application used for sign-in.
* This setting is required for enabling Twitter Sign-In.
* Twitter Sign-In documentation: https://dev.twitter.com/web/sign-in
*
*/
private @Nullable String twitterConsumerSecret;
/**
* @return The app setting name that contains the OAuth 1.0a consumer secret of the Twitter
* application used for sign-in.
*
*/
private @Nullable String twitterConsumerSecretSettingName;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return The action to take when an unauthenticated client attempts to access the app.
*
*/
private @Nullable String unauthenticatedClientAction;
/**
* @return Gets a value indicating whether the issuer should be a valid HTTPS url and be validated as such.
*
*/
private @Nullable Boolean validateIssuer;
private ListWebAppAuthSettingsResult() {}
/**
* @return Gets a JSON string containing the Azure AD Acl settings.
*
*/
public Optional aadClaimsAuthorization() {
return Optional.ofNullable(this.aadClaimsAuthorization);
}
/**
* @return Login parameters to send to the OpenID Connect authorization endpoint when
* a user logs in. Each parameter must be in the form "key=value".
*
*/
public List additionalLoginParams() {
return this.additionalLoginParams == null ? List.of() : this.additionalLoginParams;
}
/**
* @return Allowed audience values to consider when validating JSON Web Tokens issued by
* Azure Active Directory. Note that the <code>ClientID</code> value is always considered an
* allowed audience, regardless of this setting.
*
*/
public List allowedAudiences() {
return this.allowedAudiences == null ? List.of() : this.allowedAudiences;
}
/**
* @return External URLs that can be redirected to as part of logging in or logging out of the app. Note that the query string part of the URL is ignored.
* This is an advanced setting typically only needed by Windows Store application backends.
* Note that URLs within the current domain are always implicitly allowed.
*
*/
public List allowedExternalRedirectUrls() {
return this.allowedExternalRedirectUrls == null ? List.of() : this.allowedExternalRedirectUrls;
}
/**
* @return The path of the config file containing auth settings.
* If the path is relative, base will the site's root directory.
*
*/
public Optional authFilePath() {
return Optional.ofNullable(this.authFilePath);
}
/**
* @return The Client ID of this relying party application, known as the client_id.
* This setting is required for enabling OpenID Connection authentication with Azure Active Directory or
* other 3rd party OpenID Connect providers.
* More information on OpenID Connect: http://openid.net/specs/openid-connect-core-1_0.html
*
*/
public Optional clientId() {
return Optional.ofNullable(this.clientId);
}
/**
* @return The Client Secret of this relying party application (in Azure Active Directory, this is also referred to as the Key).
* This setting is optional. If no client secret is configured, the OpenID Connect implicit auth flow is used to authenticate end users.
* Otherwise, the OpenID Connect Authorization Code Flow is used to authenticate end users.
* More information on OpenID Connect: http://openid.net/specs/openid-connect-core-1_0.html
*
*/
public Optional clientSecret() {
return Optional.ofNullable(this.clientSecret);
}
/**
* @return An alternative to the client secret, that is the thumbprint of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret. It is also optional.
*
*/
public Optional clientSecretCertificateThumbprint() {
return Optional.ofNullable(this.clientSecretCertificateThumbprint);
}
/**
* @return The app setting name that contains the client secret of the relying party application.
*
*/
public Optional clientSecretSettingName() {
return Optional.ofNullable(this.clientSecretSettingName);
}
/**
* @return The ConfigVersion of the Authentication / Authorization feature in use for the current app.
* The setting in this value can control the behavior of the control plane for Authentication / Authorization.
*
*/
public Optional configVersion() {
return Optional.ofNullable(this.configVersion);
}
/**
* @return The default authentication provider to use when multiple providers are configured.
* This setting is only needed if multiple providers are configured and the unauthenticated client
* action is set to "RedirectToLoginPage".
*
*/
public Optional defaultProvider() {
return Optional.ofNullable(this.defaultProvider);
}
/**
* @return <code>true</code> if the Authentication / Authorization feature is enabled for the current app; otherwise, <code>false</code>.
*
*/
public Optional enabled() {
return Optional.ofNullable(this.enabled);
}
/**
* @return The App ID of the Facebook app used for login.
* This setting is required for enabling Facebook Login.
* Facebook Login documentation: https://developers.facebook.com/docs/facebook-login
*
*/
public Optional facebookAppId() {
return Optional.ofNullable(this.facebookAppId);
}
/**
* @return The App Secret of the Facebook app used for Facebook Login.
* This setting is required for enabling Facebook Login.
* Facebook Login documentation: https://developers.facebook.com/docs/facebook-login
*
*/
public Optional facebookAppSecret() {
return Optional.ofNullable(this.facebookAppSecret);
}
/**
* @return The app setting name that contains the app secret used for Facebook Login.
*
*/
public Optional facebookAppSecretSettingName() {
return Optional.ofNullable(this.facebookAppSecretSettingName);
}
/**
* @return The OAuth 2.0 scopes that will be requested as part of Facebook Login authentication.
* This setting is optional.
* Facebook Login documentation: https://developers.facebook.com/docs/facebook-login
*
*/
public List facebookOAuthScopes() {
return this.facebookOAuthScopes == null ? List.of() : this.facebookOAuthScopes;
}
/**
* @return The Client Id of the GitHub app used for login.
* This setting is required for enabling Github login
*
*/
public Optional gitHubClientId() {
return Optional.ofNullable(this.gitHubClientId);
}
/**
* @return The Client Secret of the GitHub app used for Github Login.
* This setting is required for enabling Github login.
*
*/
public Optional gitHubClientSecret() {
return Optional.ofNullable(this.gitHubClientSecret);
}
/**
* @return The app setting name that contains the client secret of the Github
* app used for GitHub Login.
*
*/
public Optional gitHubClientSecretSettingName() {
return Optional.ofNullable(this.gitHubClientSecretSettingName);
}
/**
* @return The OAuth 2.0 scopes that will be requested as part of GitHub Login authentication.
* This setting is optional
*
*/
public List gitHubOAuthScopes() {
return this.gitHubOAuthScopes == null ? List.of() : this.gitHubOAuthScopes;
}
/**
* @return The OpenID Connect Client ID for the Google web application.
* This setting is required for enabling Google Sign-In.
* Google Sign-In documentation: https://developers.google.com/identity/sign-in/web/
*
*/
public Optional googleClientId() {
return Optional.ofNullable(this.googleClientId);
}
/**
* @return The client secret associated with the Google web application.
* This setting is required for enabling Google Sign-In.
* Google Sign-In documentation: https://developers.google.com/identity/sign-in/web/
*
*/
public Optional googleClientSecret() {
return Optional.ofNullable(this.googleClientSecret);
}
/**
* @return The app setting name that contains the client secret associated with
* the Google web application.
*
*/
public Optional googleClientSecretSettingName() {
return Optional.ofNullable(this.googleClientSecretSettingName);
}
/**
* @return The OAuth 2.0 scopes that will be requested as part of Google Sign-In authentication.
* This setting is optional. If not specified, "openid", "profile", and "email" are used as default scopes.
* Google Sign-In documentation: https://developers.google.com/identity/sign-in/web/
*
*/
public List googleOAuthScopes() {
return this.googleOAuthScopes == null ? List.of() : this.googleOAuthScopes;
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return "true" if the auth config settings should be read from a file,
* "false" otherwise
*
*/
public Optional isAuthFromFile() {
return Optional.ofNullable(this.isAuthFromFile);
}
/**
* @return The OpenID Connect Issuer URI that represents the entity which issues access tokens for this application.
* When using Azure Active Directory, this value is the URI of the directory tenant, e.g. https://sts.windows.net/{tenant-guid}/.
* This URI is a case-sensitive identifier for the token issuer.
* More information on OpenID Connect Discovery: http://openid.net/specs/openid-connect-discovery-1_0.html
*
*/
public Optional issuer() {
return Optional.ofNullable(this.issuer);
}
/**
* @return Kind of resource.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return The OAuth 2.0 client ID that was created for the app used for authentication.
* This setting is required for enabling Microsoft Account authentication.
* Microsoft Account OAuth documentation: https://dev.onedrive.com/auth/msa_oauth.htm
*
*/
public Optional microsoftAccountClientId() {
return Optional.ofNullable(this.microsoftAccountClientId);
}
/**
* @return The OAuth 2.0 client secret that was created for the app used for authentication.
* This setting is required for enabling Microsoft Account authentication.
* Microsoft Account OAuth documentation: https://dev.onedrive.com/auth/msa_oauth.htm
*
*/
public Optional microsoftAccountClientSecret() {
return Optional.ofNullable(this.microsoftAccountClientSecret);
}
/**
* @return The app setting name containing the OAuth 2.0 client secret that was created for the
* app used for authentication.
*
*/
public Optional microsoftAccountClientSecretSettingName() {
return Optional.ofNullable(this.microsoftAccountClientSecretSettingName);
}
/**
* @return The OAuth 2.0 scopes that will be requested as part of Microsoft Account authentication.
* This setting is optional. If not specified, "wl.basic" is used as the default scope.
* Microsoft Account Scopes and permissions documentation: https://msdn.microsoft.com/en-us/library/dn631845.aspx
*
*/
public List microsoftAccountOAuthScopes() {
return this.microsoftAccountOAuthScopes == null ? List.of() : this.microsoftAccountOAuthScopes;
}
/**
* @return Resource Name.
*
*/
public String name() {
return this.name;
}
/**
* @return The RuntimeVersion of the Authentication / Authorization feature in use for the current app.
* The setting in this value can control the behavior of certain features in the Authentication / Authorization module.
*
*/
public Optional runtimeVersion() {
return Optional.ofNullable(this.runtimeVersion);
}
/**
* @return The number of hours after session token expiration that a session token can be used to
* call the token refresh API. The default is 72 hours.
*
*/
public Optional tokenRefreshExtensionHours() {
return Optional.ofNullable(this.tokenRefreshExtensionHours);
}
/**
* @return <code>true</code> to durably store platform-specific security tokens that are obtained during login flows; otherwise, <code>false</code>.
* The default is <code>false</code>.
*
*/
public Optional tokenStoreEnabled() {
return Optional.ofNullable(this.tokenStoreEnabled);
}
/**
* @return The OAuth 1.0a consumer key of the Twitter application used for sign-in.
* This setting is required for enabling Twitter Sign-In.
* Twitter Sign-In documentation: https://dev.twitter.com/web/sign-in
*
*/
public Optional twitterConsumerKey() {
return Optional.ofNullable(this.twitterConsumerKey);
}
/**
* @return The OAuth 1.0a consumer secret of the Twitter application used for sign-in.
* This setting is required for enabling Twitter Sign-In.
* Twitter Sign-In documentation: https://dev.twitter.com/web/sign-in
*
*/
public Optional twitterConsumerSecret() {
return Optional.ofNullable(this.twitterConsumerSecret);
}
/**
* @return The app setting name that contains the OAuth 1.0a consumer secret of the Twitter
* application used for sign-in.
*
*/
public Optional twitterConsumerSecretSettingName() {
return Optional.ofNullable(this.twitterConsumerSecretSettingName);
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return The action to take when an unauthenticated client attempts to access the app.
*
*/
public Optional unauthenticatedClientAction() {
return Optional.ofNullable(this.unauthenticatedClientAction);
}
/**
* @return Gets a value indicating whether the issuer should be a valid HTTPS url and be validated as such.
*
*/
public Optional validateIssuer() {
return Optional.ofNullable(this.validateIssuer);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ListWebAppAuthSettingsResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String aadClaimsAuthorization;
private @Nullable List additionalLoginParams;
private @Nullable List allowedAudiences;
private @Nullable List allowedExternalRedirectUrls;
private @Nullable String authFilePath;
private @Nullable String clientId;
private @Nullable String clientSecret;
private @Nullable String clientSecretCertificateThumbprint;
private @Nullable String clientSecretSettingName;
private @Nullable String configVersion;
private @Nullable String defaultProvider;
private @Nullable Boolean enabled;
private @Nullable String facebookAppId;
private @Nullable String facebookAppSecret;
private @Nullable String facebookAppSecretSettingName;
private @Nullable List facebookOAuthScopes;
private @Nullable String gitHubClientId;
private @Nullable String gitHubClientSecret;
private @Nullable String gitHubClientSecretSettingName;
private @Nullable List gitHubOAuthScopes;
private @Nullable String googleClientId;
private @Nullable String googleClientSecret;
private @Nullable String googleClientSecretSettingName;
private @Nullable List googleOAuthScopes;
private String id;
private @Nullable String isAuthFromFile;
private @Nullable String issuer;
private @Nullable String kind;
private @Nullable String microsoftAccountClientId;
private @Nullable String microsoftAccountClientSecret;
private @Nullable String microsoftAccountClientSecretSettingName;
private @Nullable List microsoftAccountOAuthScopes;
private String name;
private @Nullable String runtimeVersion;
private @Nullable Double tokenRefreshExtensionHours;
private @Nullable Boolean tokenStoreEnabled;
private @Nullable String twitterConsumerKey;
private @Nullable String twitterConsumerSecret;
private @Nullable String twitterConsumerSecretSettingName;
private String type;
private @Nullable String unauthenticatedClientAction;
private @Nullable Boolean validateIssuer;
public Builder() {}
public Builder(ListWebAppAuthSettingsResult defaults) {
Objects.requireNonNull(defaults);
this.aadClaimsAuthorization = defaults.aadClaimsAuthorization;
this.additionalLoginParams = defaults.additionalLoginParams;
this.allowedAudiences = defaults.allowedAudiences;
this.allowedExternalRedirectUrls = defaults.allowedExternalRedirectUrls;
this.authFilePath = defaults.authFilePath;
this.clientId = defaults.clientId;
this.clientSecret = defaults.clientSecret;
this.clientSecretCertificateThumbprint = defaults.clientSecretCertificateThumbprint;
this.clientSecretSettingName = defaults.clientSecretSettingName;
this.configVersion = defaults.configVersion;
this.defaultProvider = defaults.defaultProvider;
this.enabled = defaults.enabled;
this.facebookAppId = defaults.facebookAppId;
this.facebookAppSecret = defaults.facebookAppSecret;
this.facebookAppSecretSettingName = defaults.facebookAppSecretSettingName;
this.facebookOAuthScopes = defaults.facebookOAuthScopes;
this.gitHubClientId = defaults.gitHubClientId;
this.gitHubClientSecret = defaults.gitHubClientSecret;
this.gitHubClientSecretSettingName = defaults.gitHubClientSecretSettingName;
this.gitHubOAuthScopes = defaults.gitHubOAuthScopes;
this.googleClientId = defaults.googleClientId;
this.googleClientSecret = defaults.googleClientSecret;
this.googleClientSecretSettingName = defaults.googleClientSecretSettingName;
this.googleOAuthScopes = defaults.googleOAuthScopes;
this.id = defaults.id;
this.isAuthFromFile = defaults.isAuthFromFile;
this.issuer = defaults.issuer;
this.kind = defaults.kind;
this.microsoftAccountClientId = defaults.microsoftAccountClientId;
this.microsoftAccountClientSecret = defaults.microsoftAccountClientSecret;
this.microsoftAccountClientSecretSettingName = defaults.microsoftAccountClientSecretSettingName;
this.microsoftAccountOAuthScopes = defaults.microsoftAccountOAuthScopes;
this.name = defaults.name;
this.runtimeVersion = defaults.runtimeVersion;
this.tokenRefreshExtensionHours = defaults.tokenRefreshExtensionHours;
this.tokenStoreEnabled = defaults.tokenStoreEnabled;
this.twitterConsumerKey = defaults.twitterConsumerKey;
this.twitterConsumerSecret = defaults.twitterConsumerSecret;
this.twitterConsumerSecretSettingName = defaults.twitterConsumerSecretSettingName;
this.type = defaults.type;
this.unauthenticatedClientAction = defaults.unauthenticatedClientAction;
this.validateIssuer = defaults.validateIssuer;
}
@CustomType.Setter
public Builder aadClaimsAuthorization(@Nullable String aadClaimsAuthorization) {
this.aadClaimsAuthorization = aadClaimsAuthorization;
return this;
}
@CustomType.Setter
public Builder additionalLoginParams(@Nullable List additionalLoginParams) {
this.additionalLoginParams = additionalLoginParams;
return this;
}
public Builder additionalLoginParams(String... additionalLoginParams) {
return additionalLoginParams(List.of(additionalLoginParams));
}
@CustomType.Setter
public Builder allowedAudiences(@Nullable List allowedAudiences) {
this.allowedAudiences = allowedAudiences;
return this;
}
public Builder allowedAudiences(String... allowedAudiences) {
return allowedAudiences(List.of(allowedAudiences));
}
@CustomType.Setter
public Builder allowedExternalRedirectUrls(@Nullable List allowedExternalRedirectUrls) {
this.allowedExternalRedirectUrls = allowedExternalRedirectUrls;
return this;
}
public Builder allowedExternalRedirectUrls(String... allowedExternalRedirectUrls) {
return allowedExternalRedirectUrls(List.of(allowedExternalRedirectUrls));
}
@CustomType.Setter
public Builder authFilePath(@Nullable String authFilePath) {
this.authFilePath = authFilePath;
return this;
}
@CustomType.Setter
public Builder clientId(@Nullable String clientId) {
this.clientId = clientId;
return this;
}
@CustomType.Setter
public Builder clientSecret(@Nullable String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
@CustomType.Setter
public Builder clientSecretCertificateThumbprint(@Nullable String clientSecretCertificateThumbprint) {
this.clientSecretCertificateThumbprint = clientSecretCertificateThumbprint;
return this;
}
@CustomType.Setter
public Builder clientSecretSettingName(@Nullable String clientSecretSettingName) {
this.clientSecretSettingName = clientSecretSettingName;
return this;
}
@CustomType.Setter
public Builder configVersion(@Nullable String configVersion) {
this.configVersion = configVersion;
return this;
}
@CustomType.Setter
public Builder defaultProvider(@Nullable String defaultProvider) {
this.defaultProvider = defaultProvider;
return this;
}
@CustomType.Setter
public Builder enabled(@Nullable Boolean enabled) {
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder facebookAppId(@Nullable String facebookAppId) {
this.facebookAppId = facebookAppId;
return this;
}
@CustomType.Setter
public Builder facebookAppSecret(@Nullable String facebookAppSecret) {
this.facebookAppSecret = facebookAppSecret;
return this;
}
@CustomType.Setter
public Builder facebookAppSecretSettingName(@Nullable String facebookAppSecretSettingName) {
this.facebookAppSecretSettingName = facebookAppSecretSettingName;
return this;
}
@CustomType.Setter
public Builder facebookOAuthScopes(@Nullable List facebookOAuthScopes) {
this.facebookOAuthScopes = facebookOAuthScopes;
return this;
}
public Builder facebookOAuthScopes(String... facebookOAuthScopes) {
return facebookOAuthScopes(List.of(facebookOAuthScopes));
}
@CustomType.Setter
public Builder gitHubClientId(@Nullable String gitHubClientId) {
this.gitHubClientId = gitHubClientId;
return this;
}
@CustomType.Setter
public Builder gitHubClientSecret(@Nullable String gitHubClientSecret) {
this.gitHubClientSecret = gitHubClientSecret;
return this;
}
@CustomType.Setter
public Builder gitHubClientSecretSettingName(@Nullable String gitHubClientSecretSettingName) {
this.gitHubClientSecretSettingName = gitHubClientSecretSettingName;
return this;
}
@CustomType.Setter
public Builder gitHubOAuthScopes(@Nullable List gitHubOAuthScopes) {
this.gitHubOAuthScopes = gitHubOAuthScopes;
return this;
}
public Builder gitHubOAuthScopes(String... gitHubOAuthScopes) {
return gitHubOAuthScopes(List.of(gitHubOAuthScopes));
}
@CustomType.Setter
public Builder googleClientId(@Nullable String googleClientId) {
this.googleClientId = googleClientId;
return this;
}
@CustomType.Setter
public Builder googleClientSecret(@Nullable String googleClientSecret) {
this.googleClientSecret = googleClientSecret;
return this;
}
@CustomType.Setter
public Builder googleClientSecretSettingName(@Nullable String googleClientSecretSettingName) {
this.googleClientSecretSettingName = googleClientSecretSettingName;
return this;
}
@CustomType.Setter
public Builder googleOAuthScopes(@Nullable List googleOAuthScopes) {
this.googleOAuthScopes = googleOAuthScopes;
return this;
}
public Builder googleOAuthScopes(String... googleOAuthScopes) {
return googleOAuthScopes(List.of(googleOAuthScopes));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("ListWebAppAuthSettingsResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isAuthFromFile(@Nullable String isAuthFromFile) {
this.isAuthFromFile = isAuthFromFile;
return this;
}
@CustomType.Setter
public Builder issuer(@Nullable String issuer) {
this.issuer = issuer;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder microsoftAccountClientId(@Nullable String microsoftAccountClientId) {
this.microsoftAccountClientId = microsoftAccountClientId;
return this;
}
@CustomType.Setter
public Builder microsoftAccountClientSecret(@Nullable String microsoftAccountClientSecret) {
this.microsoftAccountClientSecret = microsoftAccountClientSecret;
return this;
}
@CustomType.Setter
public Builder microsoftAccountClientSecretSettingName(@Nullable String microsoftAccountClientSecretSettingName) {
this.microsoftAccountClientSecretSettingName = microsoftAccountClientSecretSettingName;
return this;
}
@CustomType.Setter
public Builder microsoftAccountOAuthScopes(@Nullable List microsoftAccountOAuthScopes) {
this.microsoftAccountOAuthScopes = microsoftAccountOAuthScopes;
return this;
}
public Builder microsoftAccountOAuthScopes(String... microsoftAccountOAuthScopes) {
return microsoftAccountOAuthScopes(List.of(microsoftAccountOAuthScopes));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("ListWebAppAuthSettingsResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder runtimeVersion(@Nullable String runtimeVersion) {
this.runtimeVersion = runtimeVersion;
return this;
}
@CustomType.Setter
public Builder tokenRefreshExtensionHours(@Nullable Double tokenRefreshExtensionHours) {
this.tokenRefreshExtensionHours = tokenRefreshExtensionHours;
return this;
}
@CustomType.Setter
public Builder tokenStoreEnabled(@Nullable Boolean tokenStoreEnabled) {
this.tokenStoreEnabled = tokenStoreEnabled;
return this;
}
@CustomType.Setter
public Builder twitterConsumerKey(@Nullable String twitterConsumerKey) {
this.twitterConsumerKey = twitterConsumerKey;
return this;
}
@CustomType.Setter
public Builder twitterConsumerSecret(@Nullable String twitterConsumerSecret) {
this.twitterConsumerSecret = twitterConsumerSecret;
return this;
}
@CustomType.Setter
public Builder twitterConsumerSecretSettingName(@Nullable String twitterConsumerSecretSettingName) {
this.twitterConsumerSecretSettingName = twitterConsumerSecretSettingName;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("ListWebAppAuthSettingsResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder unauthenticatedClientAction(@Nullable String unauthenticatedClientAction) {
this.unauthenticatedClientAction = unauthenticatedClientAction;
return this;
}
@CustomType.Setter
public Builder validateIssuer(@Nullable Boolean validateIssuer) {
this.validateIssuer = validateIssuer;
return this;
}
public ListWebAppAuthSettingsResult build() {
final var _resultValue = new ListWebAppAuthSettingsResult();
_resultValue.aadClaimsAuthorization = aadClaimsAuthorization;
_resultValue.additionalLoginParams = additionalLoginParams;
_resultValue.allowedAudiences = allowedAudiences;
_resultValue.allowedExternalRedirectUrls = allowedExternalRedirectUrls;
_resultValue.authFilePath = authFilePath;
_resultValue.clientId = clientId;
_resultValue.clientSecret = clientSecret;
_resultValue.clientSecretCertificateThumbprint = clientSecretCertificateThumbprint;
_resultValue.clientSecretSettingName = clientSecretSettingName;
_resultValue.configVersion = configVersion;
_resultValue.defaultProvider = defaultProvider;
_resultValue.enabled = enabled;
_resultValue.facebookAppId = facebookAppId;
_resultValue.facebookAppSecret = facebookAppSecret;
_resultValue.facebookAppSecretSettingName = facebookAppSecretSettingName;
_resultValue.facebookOAuthScopes = facebookOAuthScopes;
_resultValue.gitHubClientId = gitHubClientId;
_resultValue.gitHubClientSecret = gitHubClientSecret;
_resultValue.gitHubClientSecretSettingName = gitHubClientSecretSettingName;
_resultValue.gitHubOAuthScopes = gitHubOAuthScopes;
_resultValue.googleClientId = googleClientId;
_resultValue.googleClientSecret = googleClientSecret;
_resultValue.googleClientSecretSettingName = googleClientSecretSettingName;
_resultValue.googleOAuthScopes = googleOAuthScopes;
_resultValue.id = id;
_resultValue.isAuthFromFile = isAuthFromFile;
_resultValue.issuer = issuer;
_resultValue.kind = kind;
_resultValue.microsoftAccountClientId = microsoftAccountClientId;
_resultValue.microsoftAccountClientSecret = microsoftAccountClientSecret;
_resultValue.microsoftAccountClientSecretSettingName = microsoftAccountClientSecretSettingName;
_resultValue.microsoftAccountOAuthScopes = microsoftAccountOAuthScopes;
_resultValue.name = name;
_resultValue.runtimeVersion = runtimeVersion;
_resultValue.tokenRefreshExtensionHours = tokenRefreshExtensionHours;
_resultValue.tokenStoreEnabled = tokenStoreEnabled;
_resultValue.twitterConsumerKey = twitterConsumerKey;
_resultValue.twitterConsumerSecret = twitterConsumerSecret;
_resultValue.twitterConsumerSecretSettingName = twitterConsumerSecretSettingName;
_resultValue.type = type;
_resultValue.unauthenticatedClientAction = unauthenticatedClientAction;
_resultValue.validateIssuer = validateIssuer;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy