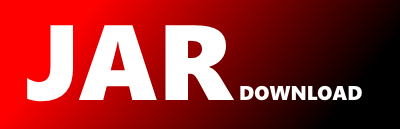
com.pulumi.azurenative.web.outputs.SiteConfigResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.outputs;
import com.pulumi.azurenative.web.outputs.ApiDefinitionInfoResponse;
import com.pulumi.azurenative.web.outputs.ApiManagementConfigResponse;
import com.pulumi.azurenative.web.outputs.AutoHealRulesResponse;
import com.pulumi.azurenative.web.outputs.AzureStorageInfoValueResponse;
import com.pulumi.azurenative.web.outputs.ConnStringInfoResponse;
import com.pulumi.azurenative.web.outputs.CorsSettingsResponse;
import com.pulumi.azurenative.web.outputs.ExperimentsResponse;
import com.pulumi.azurenative.web.outputs.HandlerMappingResponse;
import com.pulumi.azurenative.web.outputs.IpSecurityRestrictionResponse;
import com.pulumi.azurenative.web.outputs.NameValuePairResponse;
import com.pulumi.azurenative.web.outputs.PushSettingsResponse;
import com.pulumi.azurenative.web.outputs.SiteLimitsResponse;
import com.pulumi.azurenative.web.outputs.SiteMachineKeyResponse;
import com.pulumi.azurenative.web.outputs.VirtualApplicationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SiteConfigResponse {
/**
* @return Flag to use Managed Identity Creds for ACR pull
*
*/
private @Nullable Boolean acrUseManagedIdentityCreds;
/**
* @return If using user managed identity, the user managed identity ClientId
*
*/
private @Nullable String acrUserManagedIdentityID;
/**
* @return <code>true</code> if Always On is enabled; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean alwaysOn;
/**
* @return Information about the formal API definition for the app.
*
*/
private @Nullable ApiDefinitionInfoResponse apiDefinition;
/**
* @return Azure API management settings linked to the app.
*
*/
private @Nullable ApiManagementConfigResponse apiManagementConfig;
/**
* @return App command line to launch.
*
*/
private @Nullable String appCommandLine;
/**
* @return Application settings.
*
*/
private @Nullable List appSettings;
/**
* @return <code>true</code> if Auto Heal is enabled; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean autoHealEnabled;
/**
* @return Auto Heal rules.
*
*/
private @Nullable AutoHealRulesResponse autoHealRules;
/**
* @return Auto-swap slot name.
*
*/
private @Nullable String autoSwapSlotName;
/**
* @return List of Azure Storage Accounts.
*
*/
private @Nullable Map azureStorageAccounts;
/**
* @return Connection strings.
*
*/
private @Nullable List connectionStrings;
/**
* @return Cross-Origin Resource Sharing (CORS) settings.
*
*/
private @Nullable CorsSettingsResponse cors;
/**
* @return Default documents.
*
*/
private @Nullable List defaultDocuments;
/**
* @return <code>true</code> if detailed error logging is enabled; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean detailedErrorLoggingEnabled;
/**
* @return Document root.
*
*/
private @Nullable String documentRoot;
/**
* @return Maximum number of workers that a site can scale out to.
* This setting only applies to apps in plans where ElasticScaleEnabled is <code>true</code>
*
*/
private @Nullable Integer elasticWebAppScaleLimit;
/**
* @return This is work around for polymorphic types.
*
*/
private @Nullable ExperimentsResponse experiments;
/**
* @return State of FTP / FTPS service
*
*/
private @Nullable String ftpsState;
/**
* @return Maximum number of workers that a site can scale out to.
* This setting only applies to the Consumption and Elastic Premium Plans
*
*/
private @Nullable Integer functionAppScaleLimit;
/**
* @return Gets or sets a value indicating whether functions runtime scale monitoring is enabled. When enabled,
* the ScaleController will not monitor event sources directly, but will instead call to the
* runtime to get scale status.
*
*/
private @Nullable Boolean functionsRuntimeScaleMonitoringEnabled;
/**
* @return Handler mappings.
*
*/
private @Nullable List handlerMappings;
/**
* @return Health check path
*
*/
private @Nullable String healthCheckPath;
/**
* @return Http20Enabled: configures a web site to allow clients to connect over http2.0
*
*/
private @Nullable Boolean http20Enabled;
/**
* @return <code>true</code> if HTTP logging is enabled; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean httpLoggingEnabled;
/**
* @return IP security restrictions for main.
*
*/
private @Nullable List ipSecurityRestrictions;
/**
* @return Default action for main access restriction if no rules are matched.
*
*/
private @Nullable String ipSecurityRestrictionsDefaultAction;
/**
* @return Java container.
*
*/
private @Nullable String javaContainer;
/**
* @return Java container version.
*
*/
private @Nullable String javaContainerVersion;
/**
* @return Java version.
*
*/
private @Nullable String javaVersion;
/**
* @return Identity to use for Key Vault Reference authentication.
*
*/
private @Nullable String keyVaultReferenceIdentity;
/**
* @return Site limits.
*
*/
private @Nullable SiteLimitsResponse limits;
/**
* @return Linux App Framework and version
*
*/
private @Nullable String linuxFxVersion;
/**
* @return Site load balancing.
*
*/
private @Nullable String loadBalancing;
/**
* @return <code>true</code> to enable local MySQL; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean localMySqlEnabled;
/**
* @return HTTP logs directory size limit.
*
*/
private @Nullable Integer logsDirectorySizeLimit;
/**
* @return Site MachineKey.
*
*/
private SiteMachineKeyResponse machineKey;
/**
* @return Managed pipeline mode.
*
*/
private @Nullable String managedPipelineMode;
/**
* @return Managed Service Identity Id
*
*/
private @Nullable Integer managedServiceIdentityId;
/**
* @return MinTlsVersion: configures the minimum version of TLS required for SSL requests
*
*/
private @Nullable String minTlsVersion;
/**
* @return Number of minimum instance count for a site
* This setting only applies to the Elastic Plans
*
*/
private @Nullable Integer minimumElasticInstanceCount;
/**
* @return .NET Framework version.
*
*/
private @Nullable String netFrameworkVersion;
/**
* @return Version of Node.js.
*
*/
private @Nullable String nodeVersion;
/**
* @return Number of workers.
*
*/
private @Nullable Integer numberOfWorkers;
/**
* @return Version of PHP.
*
*/
private @Nullable String phpVersion;
/**
* @return Version of PowerShell.
*
*/
private @Nullable String powerShellVersion;
/**
* @return Number of preWarmed instances.
* This setting only applies to the Consumption and Elastic Plans
*
*/
private @Nullable Integer preWarmedInstanceCount;
/**
* @return Property to allow or block all public traffic.
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Publishing user name.
*
*/
private @Nullable String publishingUsername;
/**
* @return Push endpoint settings.
*
*/
private @Nullable PushSettingsResponse push;
/**
* @return Version of Python.
*
*/
private @Nullable String pythonVersion;
/**
* @return <code>true</code> if remote debugging is enabled; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean remoteDebuggingEnabled;
/**
* @return Remote debugging version.
*
*/
private @Nullable String remoteDebuggingVersion;
/**
* @return <code>true</code> if request tracing is enabled; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean requestTracingEnabled;
/**
* @return Request tracing expiration time.
*
*/
private @Nullable String requestTracingExpirationTime;
/**
* @return IP security restrictions for scm.
*
*/
private @Nullable List scmIpSecurityRestrictions;
/**
* @return Default action for scm access restriction if no rules are matched.
*
*/
private @Nullable String scmIpSecurityRestrictionsDefaultAction;
/**
* @return IP security restrictions for scm to use main.
*
*/
private @Nullable Boolean scmIpSecurityRestrictionsUseMain;
/**
* @return ScmMinTlsVersion: configures the minimum version of TLS required for SSL requests for SCM site
*
*/
private @Nullable String scmMinTlsVersion;
/**
* @return SCM type.
*
*/
private @Nullable String scmType;
/**
* @return Tracing options.
*
*/
private @Nullable String tracingOptions;
/**
* @return <code>true</code> to use 32-bit worker process; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean use32BitWorkerProcess;
/**
* @return Virtual applications.
*
*/
private @Nullable List virtualApplications;
/**
* @return Virtual Network name.
*
*/
private @Nullable String vnetName;
/**
* @return The number of private ports assigned to this app. These will be assigned dynamically on runtime.
*
*/
private @Nullable Integer vnetPrivatePortsCount;
/**
* @return Virtual Network Route All enabled. This causes all outbound traffic to have Virtual Network Security Groups and User Defined Routes applied.
*
*/
private @Nullable Boolean vnetRouteAllEnabled;
/**
* @return <code>true</code> if WebSocket is enabled; otherwise, <code>false</code>.
*
*/
private @Nullable Boolean webSocketsEnabled;
/**
* @return Sets the time zone a site uses for generating timestamps. Compatible with Linux and Windows App Service. Setting the WEBSITE_TIME_ZONE app setting takes precedence over this config. For Linux, expects tz database values https://www.iana.org/time-zones (for a quick reference see https://en.wikipedia.org/wiki/List_of_tz_database_time_zones). For Windows, expects one of the time zones listed under HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\Time Zones
*
*/
private @Nullable String websiteTimeZone;
/**
* @return Xenon App Framework and version
*
*/
private @Nullable String windowsFxVersion;
/**
* @return Explicit Managed Service Identity Id
*
*/
private @Nullable Integer xManagedServiceIdentityId;
private SiteConfigResponse() {}
/**
* @return Flag to use Managed Identity Creds for ACR pull
*
*/
public Optional acrUseManagedIdentityCreds() {
return Optional.ofNullable(this.acrUseManagedIdentityCreds);
}
/**
* @return If using user managed identity, the user managed identity ClientId
*
*/
public Optional acrUserManagedIdentityID() {
return Optional.ofNullable(this.acrUserManagedIdentityID);
}
/**
* @return <code>true</code> if Always On is enabled; otherwise, <code>false</code>.
*
*/
public Optional alwaysOn() {
return Optional.ofNullable(this.alwaysOn);
}
/**
* @return Information about the formal API definition for the app.
*
*/
public Optional apiDefinition() {
return Optional.ofNullable(this.apiDefinition);
}
/**
* @return Azure API management settings linked to the app.
*
*/
public Optional apiManagementConfig() {
return Optional.ofNullable(this.apiManagementConfig);
}
/**
* @return App command line to launch.
*
*/
public Optional appCommandLine() {
return Optional.ofNullable(this.appCommandLine);
}
/**
* @return Application settings.
*
*/
public List appSettings() {
return this.appSettings == null ? List.of() : this.appSettings;
}
/**
* @return <code>true</code> if Auto Heal is enabled; otherwise, <code>false</code>.
*
*/
public Optional autoHealEnabled() {
return Optional.ofNullable(this.autoHealEnabled);
}
/**
* @return Auto Heal rules.
*
*/
public Optional autoHealRules() {
return Optional.ofNullable(this.autoHealRules);
}
/**
* @return Auto-swap slot name.
*
*/
public Optional autoSwapSlotName() {
return Optional.ofNullable(this.autoSwapSlotName);
}
/**
* @return List of Azure Storage Accounts.
*
*/
public Map azureStorageAccounts() {
return this.azureStorageAccounts == null ? Map.of() : this.azureStorageAccounts;
}
/**
* @return Connection strings.
*
*/
public List connectionStrings() {
return this.connectionStrings == null ? List.of() : this.connectionStrings;
}
/**
* @return Cross-Origin Resource Sharing (CORS) settings.
*
*/
public Optional cors() {
return Optional.ofNullable(this.cors);
}
/**
* @return Default documents.
*
*/
public List defaultDocuments() {
return this.defaultDocuments == null ? List.of() : this.defaultDocuments;
}
/**
* @return <code>true</code> if detailed error logging is enabled; otherwise, <code>false</code>.
*
*/
public Optional detailedErrorLoggingEnabled() {
return Optional.ofNullable(this.detailedErrorLoggingEnabled);
}
/**
* @return Document root.
*
*/
public Optional documentRoot() {
return Optional.ofNullable(this.documentRoot);
}
/**
* @return Maximum number of workers that a site can scale out to.
* This setting only applies to apps in plans where ElasticScaleEnabled is <code>true</code>
*
*/
public Optional elasticWebAppScaleLimit() {
return Optional.ofNullable(this.elasticWebAppScaleLimit);
}
/**
* @return This is work around for polymorphic types.
*
*/
public Optional experiments() {
return Optional.ofNullable(this.experiments);
}
/**
* @return State of FTP / FTPS service
*
*/
public Optional ftpsState() {
return Optional.ofNullable(this.ftpsState);
}
/**
* @return Maximum number of workers that a site can scale out to.
* This setting only applies to the Consumption and Elastic Premium Plans
*
*/
public Optional functionAppScaleLimit() {
return Optional.ofNullable(this.functionAppScaleLimit);
}
/**
* @return Gets or sets a value indicating whether functions runtime scale monitoring is enabled. When enabled,
* the ScaleController will not monitor event sources directly, but will instead call to the
* runtime to get scale status.
*
*/
public Optional functionsRuntimeScaleMonitoringEnabled() {
return Optional.ofNullable(this.functionsRuntimeScaleMonitoringEnabled);
}
/**
* @return Handler mappings.
*
*/
public List handlerMappings() {
return this.handlerMappings == null ? List.of() : this.handlerMappings;
}
/**
* @return Health check path
*
*/
public Optional healthCheckPath() {
return Optional.ofNullable(this.healthCheckPath);
}
/**
* @return Http20Enabled: configures a web site to allow clients to connect over http2.0
*
*/
public Optional http20Enabled() {
return Optional.ofNullable(this.http20Enabled);
}
/**
* @return <code>true</code> if HTTP logging is enabled; otherwise, <code>false</code>.
*
*/
public Optional httpLoggingEnabled() {
return Optional.ofNullable(this.httpLoggingEnabled);
}
/**
* @return IP security restrictions for main.
*
*/
public List ipSecurityRestrictions() {
return this.ipSecurityRestrictions == null ? List.of() : this.ipSecurityRestrictions;
}
/**
* @return Default action for main access restriction if no rules are matched.
*
*/
public Optional ipSecurityRestrictionsDefaultAction() {
return Optional.ofNullable(this.ipSecurityRestrictionsDefaultAction);
}
/**
* @return Java container.
*
*/
public Optional javaContainer() {
return Optional.ofNullable(this.javaContainer);
}
/**
* @return Java container version.
*
*/
public Optional javaContainerVersion() {
return Optional.ofNullable(this.javaContainerVersion);
}
/**
* @return Java version.
*
*/
public Optional javaVersion() {
return Optional.ofNullable(this.javaVersion);
}
/**
* @return Identity to use for Key Vault Reference authentication.
*
*/
public Optional keyVaultReferenceIdentity() {
return Optional.ofNullable(this.keyVaultReferenceIdentity);
}
/**
* @return Site limits.
*
*/
public Optional limits() {
return Optional.ofNullable(this.limits);
}
/**
* @return Linux App Framework and version
*
*/
public Optional linuxFxVersion() {
return Optional.ofNullable(this.linuxFxVersion);
}
/**
* @return Site load balancing.
*
*/
public Optional loadBalancing() {
return Optional.ofNullable(this.loadBalancing);
}
/**
* @return <code>true</code> to enable local MySQL; otherwise, <code>false</code>.
*
*/
public Optional localMySqlEnabled() {
return Optional.ofNullable(this.localMySqlEnabled);
}
/**
* @return HTTP logs directory size limit.
*
*/
public Optional logsDirectorySizeLimit() {
return Optional.ofNullable(this.logsDirectorySizeLimit);
}
/**
* @return Site MachineKey.
*
*/
public SiteMachineKeyResponse machineKey() {
return this.machineKey;
}
/**
* @return Managed pipeline mode.
*
*/
public Optional managedPipelineMode() {
return Optional.ofNullable(this.managedPipelineMode);
}
/**
* @return Managed Service Identity Id
*
*/
public Optional managedServiceIdentityId() {
return Optional.ofNullable(this.managedServiceIdentityId);
}
/**
* @return MinTlsVersion: configures the minimum version of TLS required for SSL requests
*
*/
public Optional minTlsVersion() {
return Optional.ofNullable(this.minTlsVersion);
}
/**
* @return Number of minimum instance count for a site
* This setting only applies to the Elastic Plans
*
*/
public Optional minimumElasticInstanceCount() {
return Optional.ofNullable(this.minimumElasticInstanceCount);
}
/**
* @return .NET Framework version.
*
*/
public Optional netFrameworkVersion() {
return Optional.ofNullable(this.netFrameworkVersion);
}
/**
* @return Version of Node.js.
*
*/
public Optional nodeVersion() {
return Optional.ofNullable(this.nodeVersion);
}
/**
* @return Number of workers.
*
*/
public Optional numberOfWorkers() {
return Optional.ofNullable(this.numberOfWorkers);
}
/**
* @return Version of PHP.
*
*/
public Optional phpVersion() {
return Optional.ofNullable(this.phpVersion);
}
/**
* @return Version of PowerShell.
*
*/
public Optional powerShellVersion() {
return Optional.ofNullable(this.powerShellVersion);
}
/**
* @return Number of preWarmed instances.
* This setting only applies to the Consumption and Elastic Plans
*
*/
public Optional preWarmedInstanceCount() {
return Optional.ofNullable(this.preWarmedInstanceCount);
}
/**
* @return Property to allow or block all public traffic.
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Publishing user name.
*
*/
public Optional publishingUsername() {
return Optional.ofNullable(this.publishingUsername);
}
/**
* @return Push endpoint settings.
*
*/
public Optional push() {
return Optional.ofNullable(this.push);
}
/**
* @return Version of Python.
*
*/
public Optional pythonVersion() {
return Optional.ofNullable(this.pythonVersion);
}
/**
* @return <code>true</code> if remote debugging is enabled; otherwise, <code>false</code>.
*
*/
public Optional remoteDebuggingEnabled() {
return Optional.ofNullable(this.remoteDebuggingEnabled);
}
/**
* @return Remote debugging version.
*
*/
public Optional remoteDebuggingVersion() {
return Optional.ofNullable(this.remoteDebuggingVersion);
}
/**
* @return <code>true</code> if request tracing is enabled; otherwise, <code>false</code>.
*
*/
public Optional requestTracingEnabled() {
return Optional.ofNullable(this.requestTracingEnabled);
}
/**
* @return Request tracing expiration time.
*
*/
public Optional requestTracingExpirationTime() {
return Optional.ofNullable(this.requestTracingExpirationTime);
}
/**
* @return IP security restrictions for scm.
*
*/
public List scmIpSecurityRestrictions() {
return this.scmIpSecurityRestrictions == null ? List.of() : this.scmIpSecurityRestrictions;
}
/**
* @return Default action for scm access restriction if no rules are matched.
*
*/
public Optional scmIpSecurityRestrictionsDefaultAction() {
return Optional.ofNullable(this.scmIpSecurityRestrictionsDefaultAction);
}
/**
* @return IP security restrictions for scm to use main.
*
*/
public Optional scmIpSecurityRestrictionsUseMain() {
return Optional.ofNullable(this.scmIpSecurityRestrictionsUseMain);
}
/**
* @return ScmMinTlsVersion: configures the minimum version of TLS required for SSL requests for SCM site
*
*/
public Optional scmMinTlsVersion() {
return Optional.ofNullable(this.scmMinTlsVersion);
}
/**
* @return SCM type.
*
*/
public Optional scmType() {
return Optional.ofNullable(this.scmType);
}
/**
* @return Tracing options.
*
*/
public Optional tracingOptions() {
return Optional.ofNullable(this.tracingOptions);
}
/**
* @return <code>true</code> to use 32-bit worker process; otherwise, <code>false</code>.
*
*/
public Optional use32BitWorkerProcess() {
return Optional.ofNullable(this.use32BitWorkerProcess);
}
/**
* @return Virtual applications.
*
*/
public List virtualApplications() {
return this.virtualApplications == null ? List.of() : this.virtualApplications;
}
/**
* @return Virtual Network name.
*
*/
public Optional vnetName() {
return Optional.ofNullable(this.vnetName);
}
/**
* @return The number of private ports assigned to this app. These will be assigned dynamically on runtime.
*
*/
public Optional vnetPrivatePortsCount() {
return Optional.ofNullable(this.vnetPrivatePortsCount);
}
/**
* @return Virtual Network Route All enabled. This causes all outbound traffic to have Virtual Network Security Groups and User Defined Routes applied.
*
*/
public Optional vnetRouteAllEnabled() {
return Optional.ofNullable(this.vnetRouteAllEnabled);
}
/**
* @return <code>true</code> if WebSocket is enabled; otherwise, <code>false</code>.
*
*/
public Optional webSocketsEnabled() {
return Optional.ofNullable(this.webSocketsEnabled);
}
/**
* @return Sets the time zone a site uses for generating timestamps. Compatible with Linux and Windows App Service. Setting the WEBSITE_TIME_ZONE app setting takes precedence over this config. For Linux, expects tz database values https://www.iana.org/time-zones (for a quick reference see https://en.wikipedia.org/wiki/List_of_tz_database_time_zones). For Windows, expects one of the time zones listed under HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\Time Zones
*
*/
public Optional websiteTimeZone() {
return Optional.ofNullable(this.websiteTimeZone);
}
/**
* @return Xenon App Framework and version
*
*/
public Optional windowsFxVersion() {
return Optional.ofNullable(this.windowsFxVersion);
}
/**
* @return Explicit Managed Service Identity Id
*
*/
public Optional xManagedServiceIdentityId() {
return Optional.ofNullable(this.xManagedServiceIdentityId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SiteConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean acrUseManagedIdentityCreds;
private @Nullable String acrUserManagedIdentityID;
private @Nullable Boolean alwaysOn;
private @Nullable ApiDefinitionInfoResponse apiDefinition;
private @Nullable ApiManagementConfigResponse apiManagementConfig;
private @Nullable String appCommandLine;
private @Nullable List appSettings;
private @Nullable Boolean autoHealEnabled;
private @Nullable AutoHealRulesResponse autoHealRules;
private @Nullable String autoSwapSlotName;
private @Nullable Map azureStorageAccounts;
private @Nullable List connectionStrings;
private @Nullable CorsSettingsResponse cors;
private @Nullable List defaultDocuments;
private @Nullable Boolean detailedErrorLoggingEnabled;
private @Nullable String documentRoot;
private @Nullable Integer elasticWebAppScaleLimit;
private @Nullable ExperimentsResponse experiments;
private @Nullable String ftpsState;
private @Nullable Integer functionAppScaleLimit;
private @Nullable Boolean functionsRuntimeScaleMonitoringEnabled;
private @Nullable List handlerMappings;
private @Nullable String healthCheckPath;
private @Nullable Boolean http20Enabled;
private @Nullable Boolean httpLoggingEnabled;
private @Nullable List ipSecurityRestrictions;
private @Nullable String ipSecurityRestrictionsDefaultAction;
private @Nullable String javaContainer;
private @Nullable String javaContainerVersion;
private @Nullable String javaVersion;
private @Nullable String keyVaultReferenceIdentity;
private @Nullable SiteLimitsResponse limits;
private @Nullable String linuxFxVersion;
private @Nullable String loadBalancing;
private @Nullable Boolean localMySqlEnabled;
private @Nullable Integer logsDirectorySizeLimit;
private SiteMachineKeyResponse machineKey;
private @Nullable String managedPipelineMode;
private @Nullable Integer managedServiceIdentityId;
private @Nullable String minTlsVersion;
private @Nullable Integer minimumElasticInstanceCount;
private @Nullable String netFrameworkVersion;
private @Nullable String nodeVersion;
private @Nullable Integer numberOfWorkers;
private @Nullable String phpVersion;
private @Nullable String powerShellVersion;
private @Nullable Integer preWarmedInstanceCount;
private @Nullable String publicNetworkAccess;
private @Nullable String publishingUsername;
private @Nullable PushSettingsResponse push;
private @Nullable String pythonVersion;
private @Nullable Boolean remoteDebuggingEnabled;
private @Nullable String remoteDebuggingVersion;
private @Nullable Boolean requestTracingEnabled;
private @Nullable String requestTracingExpirationTime;
private @Nullable List scmIpSecurityRestrictions;
private @Nullable String scmIpSecurityRestrictionsDefaultAction;
private @Nullable Boolean scmIpSecurityRestrictionsUseMain;
private @Nullable String scmMinTlsVersion;
private @Nullable String scmType;
private @Nullable String tracingOptions;
private @Nullable Boolean use32BitWorkerProcess;
private @Nullable List virtualApplications;
private @Nullable String vnetName;
private @Nullable Integer vnetPrivatePortsCount;
private @Nullable Boolean vnetRouteAllEnabled;
private @Nullable Boolean webSocketsEnabled;
private @Nullable String websiteTimeZone;
private @Nullable String windowsFxVersion;
private @Nullable Integer xManagedServiceIdentityId;
public Builder() {}
public Builder(SiteConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.acrUseManagedIdentityCreds = defaults.acrUseManagedIdentityCreds;
this.acrUserManagedIdentityID = defaults.acrUserManagedIdentityID;
this.alwaysOn = defaults.alwaysOn;
this.apiDefinition = defaults.apiDefinition;
this.apiManagementConfig = defaults.apiManagementConfig;
this.appCommandLine = defaults.appCommandLine;
this.appSettings = defaults.appSettings;
this.autoHealEnabled = defaults.autoHealEnabled;
this.autoHealRules = defaults.autoHealRules;
this.autoSwapSlotName = defaults.autoSwapSlotName;
this.azureStorageAccounts = defaults.azureStorageAccounts;
this.connectionStrings = defaults.connectionStrings;
this.cors = defaults.cors;
this.defaultDocuments = defaults.defaultDocuments;
this.detailedErrorLoggingEnabled = defaults.detailedErrorLoggingEnabled;
this.documentRoot = defaults.documentRoot;
this.elasticWebAppScaleLimit = defaults.elasticWebAppScaleLimit;
this.experiments = defaults.experiments;
this.ftpsState = defaults.ftpsState;
this.functionAppScaleLimit = defaults.functionAppScaleLimit;
this.functionsRuntimeScaleMonitoringEnabled = defaults.functionsRuntimeScaleMonitoringEnabled;
this.handlerMappings = defaults.handlerMappings;
this.healthCheckPath = defaults.healthCheckPath;
this.http20Enabled = defaults.http20Enabled;
this.httpLoggingEnabled = defaults.httpLoggingEnabled;
this.ipSecurityRestrictions = defaults.ipSecurityRestrictions;
this.ipSecurityRestrictionsDefaultAction = defaults.ipSecurityRestrictionsDefaultAction;
this.javaContainer = defaults.javaContainer;
this.javaContainerVersion = defaults.javaContainerVersion;
this.javaVersion = defaults.javaVersion;
this.keyVaultReferenceIdentity = defaults.keyVaultReferenceIdentity;
this.limits = defaults.limits;
this.linuxFxVersion = defaults.linuxFxVersion;
this.loadBalancing = defaults.loadBalancing;
this.localMySqlEnabled = defaults.localMySqlEnabled;
this.logsDirectorySizeLimit = defaults.logsDirectorySizeLimit;
this.machineKey = defaults.machineKey;
this.managedPipelineMode = defaults.managedPipelineMode;
this.managedServiceIdentityId = defaults.managedServiceIdentityId;
this.minTlsVersion = defaults.minTlsVersion;
this.minimumElasticInstanceCount = defaults.minimumElasticInstanceCount;
this.netFrameworkVersion = defaults.netFrameworkVersion;
this.nodeVersion = defaults.nodeVersion;
this.numberOfWorkers = defaults.numberOfWorkers;
this.phpVersion = defaults.phpVersion;
this.powerShellVersion = defaults.powerShellVersion;
this.preWarmedInstanceCount = defaults.preWarmedInstanceCount;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.publishingUsername = defaults.publishingUsername;
this.push = defaults.push;
this.pythonVersion = defaults.pythonVersion;
this.remoteDebuggingEnabled = defaults.remoteDebuggingEnabled;
this.remoteDebuggingVersion = defaults.remoteDebuggingVersion;
this.requestTracingEnabled = defaults.requestTracingEnabled;
this.requestTracingExpirationTime = defaults.requestTracingExpirationTime;
this.scmIpSecurityRestrictions = defaults.scmIpSecurityRestrictions;
this.scmIpSecurityRestrictionsDefaultAction = defaults.scmIpSecurityRestrictionsDefaultAction;
this.scmIpSecurityRestrictionsUseMain = defaults.scmIpSecurityRestrictionsUseMain;
this.scmMinTlsVersion = defaults.scmMinTlsVersion;
this.scmType = defaults.scmType;
this.tracingOptions = defaults.tracingOptions;
this.use32BitWorkerProcess = defaults.use32BitWorkerProcess;
this.virtualApplications = defaults.virtualApplications;
this.vnetName = defaults.vnetName;
this.vnetPrivatePortsCount = defaults.vnetPrivatePortsCount;
this.vnetRouteAllEnabled = defaults.vnetRouteAllEnabled;
this.webSocketsEnabled = defaults.webSocketsEnabled;
this.websiteTimeZone = defaults.websiteTimeZone;
this.windowsFxVersion = defaults.windowsFxVersion;
this.xManagedServiceIdentityId = defaults.xManagedServiceIdentityId;
}
@CustomType.Setter
public Builder acrUseManagedIdentityCreds(@Nullable Boolean acrUseManagedIdentityCreds) {
this.acrUseManagedIdentityCreds = acrUseManagedIdentityCreds;
return this;
}
@CustomType.Setter
public Builder acrUserManagedIdentityID(@Nullable String acrUserManagedIdentityID) {
this.acrUserManagedIdentityID = acrUserManagedIdentityID;
return this;
}
@CustomType.Setter
public Builder alwaysOn(@Nullable Boolean alwaysOn) {
this.alwaysOn = alwaysOn;
return this;
}
@CustomType.Setter
public Builder apiDefinition(@Nullable ApiDefinitionInfoResponse apiDefinition) {
this.apiDefinition = apiDefinition;
return this;
}
@CustomType.Setter
public Builder apiManagementConfig(@Nullable ApiManagementConfigResponse apiManagementConfig) {
this.apiManagementConfig = apiManagementConfig;
return this;
}
@CustomType.Setter
public Builder appCommandLine(@Nullable String appCommandLine) {
this.appCommandLine = appCommandLine;
return this;
}
@CustomType.Setter
public Builder appSettings(@Nullable List appSettings) {
this.appSettings = appSettings;
return this;
}
public Builder appSettings(NameValuePairResponse... appSettings) {
return appSettings(List.of(appSettings));
}
@CustomType.Setter
public Builder autoHealEnabled(@Nullable Boolean autoHealEnabled) {
this.autoHealEnabled = autoHealEnabled;
return this;
}
@CustomType.Setter
public Builder autoHealRules(@Nullable AutoHealRulesResponse autoHealRules) {
this.autoHealRules = autoHealRules;
return this;
}
@CustomType.Setter
public Builder autoSwapSlotName(@Nullable String autoSwapSlotName) {
this.autoSwapSlotName = autoSwapSlotName;
return this;
}
@CustomType.Setter
public Builder azureStorageAccounts(@Nullable Map azureStorageAccounts) {
this.azureStorageAccounts = azureStorageAccounts;
return this;
}
@CustomType.Setter
public Builder connectionStrings(@Nullable List connectionStrings) {
this.connectionStrings = connectionStrings;
return this;
}
public Builder connectionStrings(ConnStringInfoResponse... connectionStrings) {
return connectionStrings(List.of(connectionStrings));
}
@CustomType.Setter
public Builder cors(@Nullable CorsSettingsResponse cors) {
this.cors = cors;
return this;
}
@CustomType.Setter
public Builder defaultDocuments(@Nullable List defaultDocuments) {
this.defaultDocuments = defaultDocuments;
return this;
}
public Builder defaultDocuments(String... defaultDocuments) {
return defaultDocuments(List.of(defaultDocuments));
}
@CustomType.Setter
public Builder detailedErrorLoggingEnabled(@Nullable Boolean detailedErrorLoggingEnabled) {
this.detailedErrorLoggingEnabled = detailedErrorLoggingEnabled;
return this;
}
@CustomType.Setter
public Builder documentRoot(@Nullable String documentRoot) {
this.documentRoot = documentRoot;
return this;
}
@CustomType.Setter
public Builder elasticWebAppScaleLimit(@Nullable Integer elasticWebAppScaleLimit) {
this.elasticWebAppScaleLimit = elasticWebAppScaleLimit;
return this;
}
@CustomType.Setter
public Builder experiments(@Nullable ExperimentsResponse experiments) {
this.experiments = experiments;
return this;
}
@CustomType.Setter
public Builder ftpsState(@Nullable String ftpsState) {
this.ftpsState = ftpsState;
return this;
}
@CustomType.Setter
public Builder functionAppScaleLimit(@Nullable Integer functionAppScaleLimit) {
this.functionAppScaleLimit = functionAppScaleLimit;
return this;
}
@CustomType.Setter
public Builder functionsRuntimeScaleMonitoringEnabled(@Nullable Boolean functionsRuntimeScaleMonitoringEnabled) {
this.functionsRuntimeScaleMonitoringEnabled = functionsRuntimeScaleMonitoringEnabled;
return this;
}
@CustomType.Setter
public Builder handlerMappings(@Nullable List handlerMappings) {
this.handlerMappings = handlerMappings;
return this;
}
public Builder handlerMappings(HandlerMappingResponse... handlerMappings) {
return handlerMappings(List.of(handlerMappings));
}
@CustomType.Setter
public Builder healthCheckPath(@Nullable String healthCheckPath) {
this.healthCheckPath = healthCheckPath;
return this;
}
@CustomType.Setter
public Builder http20Enabled(@Nullable Boolean http20Enabled) {
this.http20Enabled = http20Enabled;
return this;
}
@CustomType.Setter
public Builder httpLoggingEnabled(@Nullable Boolean httpLoggingEnabled) {
this.httpLoggingEnabled = httpLoggingEnabled;
return this;
}
@CustomType.Setter
public Builder ipSecurityRestrictions(@Nullable List ipSecurityRestrictions) {
this.ipSecurityRestrictions = ipSecurityRestrictions;
return this;
}
public Builder ipSecurityRestrictions(IpSecurityRestrictionResponse... ipSecurityRestrictions) {
return ipSecurityRestrictions(List.of(ipSecurityRestrictions));
}
@CustomType.Setter
public Builder ipSecurityRestrictionsDefaultAction(@Nullable String ipSecurityRestrictionsDefaultAction) {
this.ipSecurityRestrictionsDefaultAction = ipSecurityRestrictionsDefaultAction;
return this;
}
@CustomType.Setter
public Builder javaContainer(@Nullable String javaContainer) {
this.javaContainer = javaContainer;
return this;
}
@CustomType.Setter
public Builder javaContainerVersion(@Nullable String javaContainerVersion) {
this.javaContainerVersion = javaContainerVersion;
return this;
}
@CustomType.Setter
public Builder javaVersion(@Nullable String javaVersion) {
this.javaVersion = javaVersion;
return this;
}
@CustomType.Setter
public Builder keyVaultReferenceIdentity(@Nullable String keyVaultReferenceIdentity) {
this.keyVaultReferenceIdentity = keyVaultReferenceIdentity;
return this;
}
@CustomType.Setter
public Builder limits(@Nullable SiteLimitsResponse limits) {
this.limits = limits;
return this;
}
@CustomType.Setter
public Builder linuxFxVersion(@Nullable String linuxFxVersion) {
this.linuxFxVersion = linuxFxVersion;
return this;
}
@CustomType.Setter
public Builder loadBalancing(@Nullable String loadBalancing) {
this.loadBalancing = loadBalancing;
return this;
}
@CustomType.Setter
public Builder localMySqlEnabled(@Nullable Boolean localMySqlEnabled) {
this.localMySqlEnabled = localMySqlEnabled;
return this;
}
@CustomType.Setter
public Builder logsDirectorySizeLimit(@Nullable Integer logsDirectorySizeLimit) {
this.logsDirectorySizeLimit = logsDirectorySizeLimit;
return this;
}
@CustomType.Setter
public Builder machineKey(SiteMachineKeyResponse machineKey) {
if (machineKey == null) {
throw new MissingRequiredPropertyException("SiteConfigResponse", "machineKey");
}
this.machineKey = machineKey;
return this;
}
@CustomType.Setter
public Builder managedPipelineMode(@Nullable String managedPipelineMode) {
this.managedPipelineMode = managedPipelineMode;
return this;
}
@CustomType.Setter
public Builder managedServiceIdentityId(@Nullable Integer managedServiceIdentityId) {
this.managedServiceIdentityId = managedServiceIdentityId;
return this;
}
@CustomType.Setter
public Builder minTlsVersion(@Nullable String minTlsVersion) {
this.minTlsVersion = minTlsVersion;
return this;
}
@CustomType.Setter
public Builder minimumElasticInstanceCount(@Nullable Integer minimumElasticInstanceCount) {
this.minimumElasticInstanceCount = minimumElasticInstanceCount;
return this;
}
@CustomType.Setter
public Builder netFrameworkVersion(@Nullable String netFrameworkVersion) {
this.netFrameworkVersion = netFrameworkVersion;
return this;
}
@CustomType.Setter
public Builder nodeVersion(@Nullable String nodeVersion) {
this.nodeVersion = nodeVersion;
return this;
}
@CustomType.Setter
public Builder numberOfWorkers(@Nullable Integer numberOfWorkers) {
this.numberOfWorkers = numberOfWorkers;
return this;
}
@CustomType.Setter
public Builder phpVersion(@Nullable String phpVersion) {
this.phpVersion = phpVersion;
return this;
}
@CustomType.Setter
public Builder powerShellVersion(@Nullable String powerShellVersion) {
this.powerShellVersion = powerShellVersion;
return this;
}
@CustomType.Setter
public Builder preWarmedInstanceCount(@Nullable Integer preWarmedInstanceCount) {
this.preWarmedInstanceCount = preWarmedInstanceCount;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder publishingUsername(@Nullable String publishingUsername) {
this.publishingUsername = publishingUsername;
return this;
}
@CustomType.Setter
public Builder push(@Nullable PushSettingsResponse push) {
this.push = push;
return this;
}
@CustomType.Setter
public Builder pythonVersion(@Nullable String pythonVersion) {
this.pythonVersion = pythonVersion;
return this;
}
@CustomType.Setter
public Builder remoteDebuggingEnabled(@Nullable Boolean remoteDebuggingEnabled) {
this.remoteDebuggingEnabled = remoteDebuggingEnabled;
return this;
}
@CustomType.Setter
public Builder remoteDebuggingVersion(@Nullable String remoteDebuggingVersion) {
this.remoteDebuggingVersion = remoteDebuggingVersion;
return this;
}
@CustomType.Setter
public Builder requestTracingEnabled(@Nullable Boolean requestTracingEnabled) {
this.requestTracingEnabled = requestTracingEnabled;
return this;
}
@CustomType.Setter
public Builder requestTracingExpirationTime(@Nullable String requestTracingExpirationTime) {
this.requestTracingExpirationTime = requestTracingExpirationTime;
return this;
}
@CustomType.Setter
public Builder scmIpSecurityRestrictions(@Nullable List scmIpSecurityRestrictions) {
this.scmIpSecurityRestrictions = scmIpSecurityRestrictions;
return this;
}
public Builder scmIpSecurityRestrictions(IpSecurityRestrictionResponse... scmIpSecurityRestrictions) {
return scmIpSecurityRestrictions(List.of(scmIpSecurityRestrictions));
}
@CustomType.Setter
public Builder scmIpSecurityRestrictionsDefaultAction(@Nullable String scmIpSecurityRestrictionsDefaultAction) {
this.scmIpSecurityRestrictionsDefaultAction = scmIpSecurityRestrictionsDefaultAction;
return this;
}
@CustomType.Setter
public Builder scmIpSecurityRestrictionsUseMain(@Nullable Boolean scmIpSecurityRestrictionsUseMain) {
this.scmIpSecurityRestrictionsUseMain = scmIpSecurityRestrictionsUseMain;
return this;
}
@CustomType.Setter
public Builder scmMinTlsVersion(@Nullable String scmMinTlsVersion) {
this.scmMinTlsVersion = scmMinTlsVersion;
return this;
}
@CustomType.Setter
public Builder scmType(@Nullable String scmType) {
this.scmType = scmType;
return this;
}
@CustomType.Setter
public Builder tracingOptions(@Nullable String tracingOptions) {
this.tracingOptions = tracingOptions;
return this;
}
@CustomType.Setter
public Builder use32BitWorkerProcess(@Nullable Boolean use32BitWorkerProcess) {
this.use32BitWorkerProcess = use32BitWorkerProcess;
return this;
}
@CustomType.Setter
public Builder virtualApplications(@Nullable List virtualApplications) {
this.virtualApplications = virtualApplications;
return this;
}
public Builder virtualApplications(VirtualApplicationResponse... virtualApplications) {
return virtualApplications(List.of(virtualApplications));
}
@CustomType.Setter
public Builder vnetName(@Nullable String vnetName) {
this.vnetName = vnetName;
return this;
}
@CustomType.Setter
public Builder vnetPrivatePortsCount(@Nullable Integer vnetPrivatePortsCount) {
this.vnetPrivatePortsCount = vnetPrivatePortsCount;
return this;
}
@CustomType.Setter
public Builder vnetRouteAllEnabled(@Nullable Boolean vnetRouteAllEnabled) {
this.vnetRouteAllEnabled = vnetRouteAllEnabled;
return this;
}
@CustomType.Setter
public Builder webSocketsEnabled(@Nullable Boolean webSocketsEnabled) {
this.webSocketsEnabled = webSocketsEnabled;
return this;
}
@CustomType.Setter
public Builder websiteTimeZone(@Nullable String websiteTimeZone) {
this.websiteTimeZone = websiteTimeZone;
return this;
}
@CustomType.Setter
public Builder windowsFxVersion(@Nullable String windowsFxVersion) {
this.windowsFxVersion = windowsFxVersion;
return this;
}
@CustomType.Setter
public Builder xManagedServiceIdentityId(@Nullable Integer xManagedServiceIdentityId) {
this.xManagedServiceIdentityId = xManagedServiceIdentityId;
return this;
}
public SiteConfigResponse build() {
final var _resultValue = new SiteConfigResponse();
_resultValue.acrUseManagedIdentityCreds = acrUseManagedIdentityCreds;
_resultValue.acrUserManagedIdentityID = acrUserManagedIdentityID;
_resultValue.alwaysOn = alwaysOn;
_resultValue.apiDefinition = apiDefinition;
_resultValue.apiManagementConfig = apiManagementConfig;
_resultValue.appCommandLine = appCommandLine;
_resultValue.appSettings = appSettings;
_resultValue.autoHealEnabled = autoHealEnabled;
_resultValue.autoHealRules = autoHealRules;
_resultValue.autoSwapSlotName = autoSwapSlotName;
_resultValue.azureStorageAccounts = azureStorageAccounts;
_resultValue.connectionStrings = connectionStrings;
_resultValue.cors = cors;
_resultValue.defaultDocuments = defaultDocuments;
_resultValue.detailedErrorLoggingEnabled = detailedErrorLoggingEnabled;
_resultValue.documentRoot = documentRoot;
_resultValue.elasticWebAppScaleLimit = elasticWebAppScaleLimit;
_resultValue.experiments = experiments;
_resultValue.ftpsState = ftpsState;
_resultValue.functionAppScaleLimit = functionAppScaleLimit;
_resultValue.functionsRuntimeScaleMonitoringEnabled = functionsRuntimeScaleMonitoringEnabled;
_resultValue.handlerMappings = handlerMappings;
_resultValue.healthCheckPath = healthCheckPath;
_resultValue.http20Enabled = http20Enabled;
_resultValue.httpLoggingEnabled = httpLoggingEnabled;
_resultValue.ipSecurityRestrictions = ipSecurityRestrictions;
_resultValue.ipSecurityRestrictionsDefaultAction = ipSecurityRestrictionsDefaultAction;
_resultValue.javaContainer = javaContainer;
_resultValue.javaContainerVersion = javaContainerVersion;
_resultValue.javaVersion = javaVersion;
_resultValue.keyVaultReferenceIdentity = keyVaultReferenceIdentity;
_resultValue.limits = limits;
_resultValue.linuxFxVersion = linuxFxVersion;
_resultValue.loadBalancing = loadBalancing;
_resultValue.localMySqlEnabled = localMySqlEnabled;
_resultValue.logsDirectorySizeLimit = logsDirectorySizeLimit;
_resultValue.machineKey = machineKey;
_resultValue.managedPipelineMode = managedPipelineMode;
_resultValue.managedServiceIdentityId = managedServiceIdentityId;
_resultValue.minTlsVersion = minTlsVersion;
_resultValue.minimumElasticInstanceCount = minimumElasticInstanceCount;
_resultValue.netFrameworkVersion = netFrameworkVersion;
_resultValue.nodeVersion = nodeVersion;
_resultValue.numberOfWorkers = numberOfWorkers;
_resultValue.phpVersion = phpVersion;
_resultValue.powerShellVersion = powerShellVersion;
_resultValue.preWarmedInstanceCount = preWarmedInstanceCount;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.publishingUsername = publishingUsername;
_resultValue.push = push;
_resultValue.pythonVersion = pythonVersion;
_resultValue.remoteDebuggingEnabled = remoteDebuggingEnabled;
_resultValue.remoteDebuggingVersion = remoteDebuggingVersion;
_resultValue.requestTracingEnabled = requestTracingEnabled;
_resultValue.requestTracingExpirationTime = requestTracingExpirationTime;
_resultValue.scmIpSecurityRestrictions = scmIpSecurityRestrictions;
_resultValue.scmIpSecurityRestrictionsDefaultAction = scmIpSecurityRestrictionsDefaultAction;
_resultValue.scmIpSecurityRestrictionsUseMain = scmIpSecurityRestrictionsUseMain;
_resultValue.scmMinTlsVersion = scmMinTlsVersion;
_resultValue.scmType = scmType;
_resultValue.tracingOptions = tracingOptions;
_resultValue.use32BitWorkerProcess = use32BitWorkerProcess;
_resultValue.virtualApplications = virtualApplications;
_resultValue.vnetName = vnetName;
_resultValue.vnetPrivatePortsCount = vnetPrivatePortsCount;
_resultValue.vnetRouteAllEnabled = vnetRouteAllEnabled;
_resultValue.webSocketsEnabled = webSocketsEnabled;
_resultValue.websiteTimeZone = websiteTimeZone;
_resultValue.windowsFxVersion = windowsFxVersion;
_resultValue.xManagedServiceIdentityId = xManagedServiceIdentityId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy