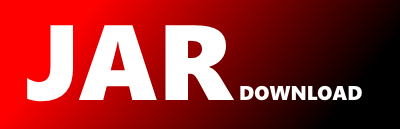
com.pulumi.azurenative.workloads.SAPCentralInstance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.workloads;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.workloads.SAPCentralInstanceArgs;
import com.pulumi.azurenative.workloads.outputs.CentralServerVmDetailsResponse;
import com.pulumi.azurenative.workloads.outputs.EnqueueReplicationServerPropertiesResponse;
import com.pulumi.azurenative.workloads.outputs.EnqueueServerPropertiesResponse;
import com.pulumi.azurenative.workloads.outputs.GatewayServerPropertiesResponse;
import com.pulumi.azurenative.workloads.outputs.LoadBalancerDetailsResponse;
import com.pulumi.azurenative.workloads.outputs.MessageServerPropertiesResponse;
import com.pulumi.azurenative.workloads.outputs.SAPVirtualInstanceErrorResponse;
import com.pulumi.azurenative.workloads.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Define the SAP Central Services Instance resource.
* Azure REST API version: 2023-04-01. Prior API version in Azure Native 1.x: 2021-12-01-preview.
*
* Other available API versions: 2023-10-01-preview.
*
* ## Example Usage
* ### Create SAP Central Instances for HA System with Availability Set
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.workloads.SAPCentralInstance;
* import com.pulumi.azurenative.workloads.SAPCentralInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var sapCentralInstance = new SAPCentralInstance("sapCentralInstance", SAPCentralInstanceArgs.builder()
* .centralInstanceName("centralServer")
* .location("westcentralus")
* .resourceGroupName("test-rg")
* .sapVirtualInstanceName("X00")
* .tags()
* .build());
*
* }
* }
*
* }
*
* ### SAPCentralInstances_Create
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.workloads.SAPCentralInstance;
* import com.pulumi.azurenative.workloads.SAPCentralInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var sapCentralInstance = new SAPCentralInstance("sapCentralInstance", SAPCentralInstanceArgs.builder()
* .centralInstanceName("centralServer")
* .location("westcentralus")
* .resourceGroupName("test-rg")
* .sapVirtualInstanceName("X00")
* .tags()
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:workloads:SAPCentralInstance centralServer /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Workloads/sapVirtualInstances/{sapVirtualInstanceName}/centralInstances/{centralInstanceName}
* ```
*
*/
@ResourceType(type="azure-native:workloads:SAPCentralInstance")
public class SAPCentralInstance extends com.pulumi.resources.CustomResource {
/**
* Defines the SAP Enqueue Replication Server (ERS) properties.
*
*/
@Export(name="enqueueReplicationServerProperties", refs={EnqueueReplicationServerPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ EnqueueReplicationServerPropertiesResponse> enqueueReplicationServerProperties;
/**
* @return Defines the SAP Enqueue Replication Server (ERS) properties.
*
*/
public Output> enqueueReplicationServerProperties() {
return Codegen.optional(this.enqueueReplicationServerProperties);
}
/**
* Defines the SAP Enqueue Server properties.
*
*/
@Export(name="enqueueServerProperties", refs={EnqueueServerPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ EnqueueServerPropertiesResponse> enqueueServerProperties;
/**
* @return Defines the SAP Enqueue Server properties.
*
*/
public Output> enqueueServerProperties() {
return Codegen.optional(this.enqueueServerProperties);
}
/**
* Defines the errors related to SAP Central Services Instance resource.
*
*/
@Export(name="errors", refs={SAPVirtualInstanceErrorResponse.class}, tree="[0]")
private Output errors;
/**
* @return Defines the errors related to SAP Central Services Instance resource.
*
*/
public Output errors() {
return this.errors;
}
/**
* Defines the SAP Gateway Server properties.
*
*/
@Export(name="gatewayServerProperties", refs={GatewayServerPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ GatewayServerPropertiesResponse> gatewayServerProperties;
/**
* @return Defines the SAP Gateway Server properties.
*
*/
public Output> gatewayServerProperties() {
return Codegen.optional(this.gatewayServerProperties);
}
/**
* Defines the health of SAP Instances.
*
*/
@Export(name="health", refs={String.class}, tree="[0]")
private Output health;
/**
* @return Defines the health of SAP Instances.
*
*/
public Output health() {
return this.health;
}
/**
* The central services instance number.
*
*/
@Export(name="instanceNo", refs={String.class}, tree="[0]")
private Output instanceNo;
/**
* @return The central services instance number.
*
*/
public Output instanceNo() {
return this.instanceNo;
}
/**
* The central services instance Kernel Patch level.
*
*/
@Export(name="kernelPatch", refs={String.class}, tree="[0]")
private Output kernelPatch;
/**
* @return The central services instance Kernel Patch level.
*
*/
public Output kernelPatch() {
return this.kernelPatch;
}
/**
* The central services instance Kernel Version.
*
*/
@Export(name="kernelVersion", refs={String.class}, tree="[0]")
private Output kernelVersion;
/**
* @return The central services instance Kernel Version.
*
*/
public Output kernelVersion() {
return this.kernelVersion;
}
/**
* The Load Balancer details such as LoadBalancer ID attached to ASCS Virtual Machines
*
*/
@Export(name="loadBalancerDetails", refs={LoadBalancerDetailsResponse.class}, tree="[0]")
private Output loadBalancerDetails;
/**
* @return The Load Balancer details such as LoadBalancer ID attached to ASCS Virtual Machines
*
*/
public Output loadBalancerDetails() {
return this.loadBalancerDetails;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* Defines the SAP Message Server properties.
*
*/
@Export(name="messageServerProperties", refs={MessageServerPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ MessageServerPropertiesResponse> messageServerProperties;
/**
* @return Defines the SAP Message Server properties.
*
*/
public Output> messageServerProperties() {
return Codegen.optional(this.messageServerProperties);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Defines the provisioning states.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Defines the provisioning states.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Defines the SAP Instance status.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Defines the SAP Instance status.
*
*/
public Output status() {
return this.status;
}
/**
* The central services instance subnet.
*
*/
@Export(name="subnet", refs={String.class}, tree="[0]")
private Output subnet;
/**
* @return The central services instance subnet.
*
*/
public Output subnet() {
return this.subnet;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* The list of virtual machines corresponding to the Central Services instance.
*
*/
@Export(name="vmDetails", refs={List.class,CentralServerVmDetailsResponse.class}, tree="[0,1]")
private Output> vmDetails;
/**
* @return The list of virtual machines corresponding to the Central Services instance.
*
*/
public Output> vmDetails() {
return this.vmDetails;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public SAPCentralInstance(java.lang.String name) {
this(name, SAPCentralInstanceArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public SAPCentralInstance(java.lang.String name, SAPCentralInstanceArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public SAPCentralInstance(java.lang.String name, SAPCentralInstanceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:workloads:SAPCentralInstance", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private SAPCentralInstance(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:workloads:SAPCentralInstance", name, null, makeResourceOptions(options, id), false);
}
private static SAPCentralInstanceArgs makeArgs(SAPCentralInstanceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SAPCentralInstanceArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:workloads/v20211201preview:SAPCentralInstance").build()),
Output.of(Alias.builder().type("azure-native:workloads/v20221101preview:SAPCentralInstance").build()),
Output.of(Alias.builder().type("azure-native:workloads/v20230401:SAPCentralInstance").build()),
Output.of(Alias.builder().type("azure-native:workloads/v20231001preview:SAPCentralInstance").build()),
Output.of(Alias.builder().type("azure-native:workloads/v20240901:SAPCentralInstance").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static SAPCentralInstance get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new SAPCentralInstance(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy