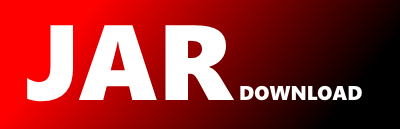
com.pulumi.azurenative.workloads.inputs.GetSAPSizingRecommendationsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.workloads.inputs;
import com.pulumi.azurenative.workloads.enums.SAPDatabaseScaleMethod;
import com.pulumi.azurenative.workloads.enums.SAPDatabaseType;
import com.pulumi.azurenative.workloads.enums.SAPDeploymentType;
import com.pulumi.azurenative.workloads.enums.SAPEnvironmentType;
import com.pulumi.azurenative.workloads.enums.SAPHighAvailabilityType;
import com.pulumi.azurenative.workloads.enums.SAPProductType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetSAPSizingRecommendationsArgs extends com.pulumi.resources.InvokeArgs {
public static final GetSAPSizingRecommendationsArgs Empty = new GetSAPSizingRecommendationsArgs();
/**
* The geo-location where the resource is to be created.
*
*/
@Import(name="appLocation", required=true)
private Output appLocation;
/**
* @return The geo-location where the resource is to be created.
*
*/
public Output appLocation() {
return this.appLocation;
}
/**
* The database type.
*
*/
@Import(name="databaseType", required=true)
private Output> databaseType;
/**
* @return The database type.
*
*/
public Output> databaseType() {
return this.databaseType;
}
/**
* The database memory configuration.
*
*/
@Import(name="dbMemory", required=true)
private Output dbMemory;
/**
* @return The database memory configuration.
*
*/
public Output dbMemory() {
return this.dbMemory;
}
/**
* The DB scale method.
*
*/
@Import(name="dbScaleMethod")
private @Nullable Output> dbScaleMethod;
/**
* @return The DB scale method.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy