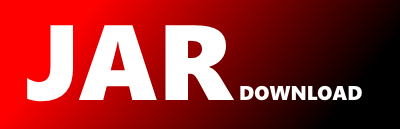
com.pulumi.azurenative.aad.outputs.HealthAlertResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.aad.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class HealthAlertResponse {
/**
* @return Health Alert Id
*
*/
private String id;
/**
* @return Health Alert Issue
*
*/
private String issue;
/**
* @return Health Alert Last Detected DateTime
*
*/
private String lastDetected;
/**
* @return Health Alert Name
*
*/
private String name;
/**
* @return Health Alert Raised DateTime
*
*/
private String raised;
/**
* @return Health Alert TSG Link
*
*/
private String resolutionUri;
/**
* @return Health Alert Severity
*
*/
private String severity;
private HealthAlertResponse() {}
/**
* @return Health Alert Id
*
*/
public String id() {
return this.id;
}
/**
* @return Health Alert Issue
*
*/
public String issue() {
return this.issue;
}
/**
* @return Health Alert Last Detected DateTime
*
*/
public String lastDetected() {
return this.lastDetected;
}
/**
* @return Health Alert Name
*
*/
public String name() {
return this.name;
}
/**
* @return Health Alert Raised DateTime
*
*/
public String raised() {
return this.raised;
}
/**
* @return Health Alert TSG Link
*
*/
public String resolutionUri() {
return this.resolutionUri;
}
/**
* @return Health Alert Severity
*
*/
public String severity() {
return this.severity;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HealthAlertResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String id;
private String issue;
private String lastDetected;
private String name;
private String raised;
private String resolutionUri;
private String severity;
public Builder() {}
public Builder(HealthAlertResponse defaults) {
Objects.requireNonNull(defaults);
this.id = defaults.id;
this.issue = defaults.issue;
this.lastDetected = defaults.lastDetected;
this.name = defaults.name;
this.raised = defaults.raised;
this.resolutionUri = defaults.resolutionUri;
this.severity = defaults.severity;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("HealthAlertResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder issue(String issue) {
if (issue == null) {
throw new MissingRequiredPropertyException("HealthAlertResponse", "issue");
}
this.issue = issue;
return this;
}
@CustomType.Setter
public Builder lastDetected(String lastDetected) {
if (lastDetected == null) {
throw new MissingRequiredPropertyException("HealthAlertResponse", "lastDetected");
}
this.lastDetected = lastDetected;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("HealthAlertResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder raised(String raised) {
if (raised == null) {
throw new MissingRequiredPropertyException("HealthAlertResponse", "raised");
}
this.raised = raised;
return this;
}
@CustomType.Setter
public Builder resolutionUri(String resolutionUri) {
if (resolutionUri == null) {
throw new MissingRequiredPropertyException("HealthAlertResponse", "resolutionUri");
}
this.resolutionUri = resolutionUri;
return this;
}
@CustomType.Setter
public Builder severity(String severity) {
if (severity == null) {
throw new MissingRequiredPropertyException("HealthAlertResponse", "severity");
}
this.severity = severity;
return this;
}
public HealthAlertResponse build() {
final var _resultValue = new HealthAlertResponse();
_resultValue.id = id;
_resultValue.issue = issue;
_resultValue.lastDetected = lastDetected;
_resultValue.name = name;
_resultValue.raised = raised;
_resultValue.resolutionUri = resolutionUri;
_resultValue.severity = severity;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy