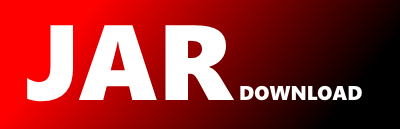
com.pulumi.azurenative.analysisservices.outputs.GetServerDetailsResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.analysisservices.outputs;
import com.pulumi.azurenative.analysisservices.outputs.GatewayDetailsResponse;
import com.pulumi.azurenative.analysisservices.outputs.IPv4FirewallSettingsResponse;
import com.pulumi.azurenative.analysisservices.outputs.ResourceSkuResponse;
import com.pulumi.azurenative.analysisservices.outputs.ServerAdministratorsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetServerDetailsResult {
/**
* @return A collection of AS server administrators
*
*/
private @Nullable ServerAdministratorsResponse asAdministrators;
/**
* @return The SAS container URI to the backup container.
*
*/
private @Nullable String backupBlobContainerUri;
/**
* @return The gateway details configured for the AS server.
*
*/
private @Nullable GatewayDetailsResponse gatewayDetails;
/**
* @return An identifier that represents the Analysis Services resource.
*
*/
private String id;
/**
* @return The firewall settings for the AS server.
*
*/
private @Nullable IPv4FirewallSettingsResponse ipV4FirewallSettings;
/**
* @return Location of the Analysis Services resource.
*
*/
private String location;
/**
* @return The managed mode of the server (0 = not managed, 1 = managed).
*
*/
private @Nullable Integer managedMode;
/**
* @return The name of the Analysis Services resource.
*
*/
private String name;
/**
* @return The current deployment state of Analysis Services resource. The provisioningState is to indicate states for resource provisioning.
*
*/
private String provisioningState;
/**
* @return How the read-write server's participation in the query pool is controlled.<br/>It can have the following values: <ul><li>readOnly - indicates that the read-write server is intended not to participate in query operations</li><li>all - indicates that the read-write server can participate in query operations</li></ul>Specifying readOnly when capacity is 1 results in error.
*
*/
private @Nullable String querypoolConnectionMode;
/**
* @return The full name of the Analysis Services resource.
*
*/
private String serverFullName;
/**
* @return The server monitor mode for AS server
*
*/
private @Nullable Integer serverMonitorMode;
/**
* @return The SKU of the Analysis Services resource.
*
*/
private ResourceSkuResponse sku;
/**
* @return The current state of Analysis Services resource. The state is to indicate more states outside of resource provisioning.
*
*/
private String state;
/**
* @return Key-value pairs of additional resource provisioning properties.
*
*/
private @Nullable Map tags;
/**
* @return The type of the Analysis Services resource.
*
*/
private String type;
private GetServerDetailsResult() {}
/**
* @return A collection of AS server administrators
*
*/
public Optional asAdministrators() {
return Optional.ofNullable(this.asAdministrators);
}
/**
* @return The SAS container URI to the backup container.
*
*/
public Optional backupBlobContainerUri() {
return Optional.ofNullable(this.backupBlobContainerUri);
}
/**
* @return The gateway details configured for the AS server.
*
*/
public Optional gatewayDetails() {
return Optional.ofNullable(this.gatewayDetails);
}
/**
* @return An identifier that represents the Analysis Services resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The firewall settings for the AS server.
*
*/
public Optional ipV4FirewallSettings() {
return Optional.ofNullable(this.ipV4FirewallSettings);
}
/**
* @return Location of the Analysis Services resource.
*
*/
public String location() {
return this.location;
}
/**
* @return The managed mode of the server (0 = not managed, 1 = managed).
*
*/
public Optional managedMode() {
return Optional.ofNullable(this.managedMode);
}
/**
* @return The name of the Analysis Services resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The current deployment state of Analysis Services resource. The provisioningState is to indicate states for resource provisioning.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return How the read-write server's participation in the query pool is controlled.<br/>It can have the following values: <ul><li>readOnly - indicates that the read-write server is intended not to participate in query operations</li><li>all - indicates that the read-write server can participate in query operations</li></ul>Specifying readOnly when capacity is 1 results in error.
*
*/
public Optional querypoolConnectionMode() {
return Optional.ofNullable(this.querypoolConnectionMode);
}
/**
* @return The full name of the Analysis Services resource.
*
*/
public String serverFullName() {
return this.serverFullName;
}
/**
* @return The server monitor mode for AS server
*
*/
public Optional serverMonitorMode() {
return Optional.ofNullable(this.serverMonitorMode);
}
/**
* @return The SKU of the Analysis Services resource.
*
*/
public ResourceSkuResponse sku() {
return this.sku;
}
/**
* @return The current state of Analysis Services resource. The state is to indicate more states outside of resource provisioning.
*
*/
public String state() {
return this.state;
}
/**
* @return Key-value pairs of additional resource provisioning properties.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the Analysis Services resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServerDetailsResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ServerAdministratorsResponse asAdministrators;
private @Nullable String backupBlobContainerUri;
private @Nullable GatewayDetailsResponse gatewayDetails;
private String id;
private @Nullable IPv4FirewallSettingsResponse ipV4FirewallSettings;
private String location;
private @Nullable Integer managedMode;
private String name;
private String provisioningState;
private @Nullable String querypoolConnectionMode;
private String serverFullName;
private @Nullable Integer serverMonitorMode;
private ResourceSkuResponse sku;
private String state;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetServerDetailsResult defaults) {
Objects.requireNonNull(defaults);
this.asAdministrators = defaults.asAdministrators;
this.backupBlobContainerUri = defaults.backupBlobContainerUri;
this.gatewayDetails = defaults.gatewayDetails;
this.id = defaults.id;
this.ipV4FirewallSettings = defaults.ipV4FirewallSettings;
this.location = defaults.location;
this.managedMode = defaults.managedMode;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.querypoolConnectionMode = defaults.querypoolConnectionMode;
this.serverFullName = defaults.serverFullName;
this.serverMonitorMode = defaults.serverMonitorMode;
this.sku = defaults.sku;
this.state = defaults.state;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder asAdministrators(@Nullable ServerAdministratorsResponse asAdministrators) {
this.asAdministrators = asAdministrators;
return this;
}
@CustomType.Setter
public Builder backupBlobContainerUri(@Nullable String backupBlobContainerUri) {
this.backupBlobContainerUri = backupBlobContainerUri;
return this;
}
@CustomType.Setter
public Builder gatewayDetails(@Nullable GatewayDetailsResponse gatewayDetails) {
this.gatewayDetails = gatewayDetails;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipV4FirewallSettings(@Nullable IPv4FirewallSettingsResponse ipV4FirewallSettings) {
this.ipV4FirewallSettings = ipV4FirewallSettings;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedMode(@Nullable Integer managedMode) {
this.managedMode = managedMode;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder querypoolConnectionMode(@Nullable String querypoolConnectionMode) {
this.querypoolConnectionMode = querypoolConnectionMode;
return this;
}
@CustomType.Setter
public Builder serverFullName(String serverFullName) {
if (serverFullName == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "serverFullName");
}
this.serverFullName = serverFullName;
return this;
}
@CustomType.Setter
public Builder serverMonitorMode(@Nullable Integer serverMonitorMode) {
this.serverMonitorMode = serverMonitorMode;
return this;
}
@CustomType.Setter
public Builder sku(ResourceSkuResponse sku) {
if (sku == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "sku");
}
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetServerDetailsResult", "type");
}
this.type = type;
return this;
}
public GetServerDetailsResult build() {
final var _resultValue = new GetServerDetailsResult();
_resultValue.asAdministrators = asAdministrators;
_resultValue.backupBlobContainerUri = backupBlobContainerUri;
_resultValue.gatewayDetails = gatewayDetails;
_resultValue.id = id;
_resultValue.ipV4FirewallSettings = ipV4FirewallSettings;
_resultValue.location = location;
_resultValue.managedMode = managedMode;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.querypoolConnectionMode = querypoolConnectionMode;
_resultValue.serverFullName = serverFullName;
_resultValue.serverMonitorMode = serverMonitorMode;
_resultValue.sku = sku;
_resultValue.state = state;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy