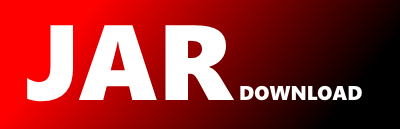
com.pulumi.azurenative.apimanagement.ApiGateway Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.apimanagement.ApiGatewayArgs;
import com.pulumi.azurenative.apimanagement.outputs.ApiManagementGatewaySkuPropertiesResponse;
import com.pulumi.azurenative.apimanagement.outputs.BackendConfigurationResponse;
import com.pulumi.azurenative.apimanagement.outputs.FrontendConfigurationResponse;
import com.pulumi.azurenative.apimanagement.outputs.GatewayConfigurationApiResponse;
import com.pulumi.azurenative.apimanagement.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A single API Management gateway resource in List or Get response.
* Azure REST API version: 2023-09-01-preview.
*
* Other available API versions: 2024-05-01.
*
* ## Example Usage
* ### ApiManagementCreateStandardGateway
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.ApiGateway;
* import com.pulumi.azurenative.apimanagement.ApiGatewayArgs;
* import com.pulumi.azurenative.apimanagement.inputs.BackendConfigurationArgs;
* import com.pulumi.azurenative.apimanagement.inputs.BackendSubnetConfigurationArgs;
* import com.pulumi.azurenative.apimanagement.inputs.ApiManagementGatewaySkuPropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var apiGateway = new ApiGateway("apiGateway", ApiGatewayArgs.builder()
* .backend(BackendConfigurationArgs.builder()
* .subnet(BackendSubnetConfigurationArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vn1/subnets/sn1")
* .build())
* .build())
* .gatewayName("apimGateway1")
* .location("South Central US")
* .resourceGroupName("rg1")
* .sku(ApiManagementGatewaySkuPropertiesArgs.builder()
* .capacity(1)
* .name("Standard")
* .build())
* .tags(Map.ofEntries(
* Map.entry("Name", "Contoso"),
* Map.entry("Test", "User")
* ))
* .build());
*
* }
* }
*
* }
*
* ### ApiManagementCreateWorkspacePremiumGateway
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.ApiGateway;
* import com.pulumi.azurenative.apimanagement.ApiGatewayArgs;
* import com.pulumi.azurenative.apimanagement.inputs.BackendConfigurationArgs;
* import com.pulumi.azurenative.apimanagement.inputs.BackendSubnetConfigurationArgs;
* import com.pulumi.azurenative.apimanagement.inputs.ApiManagementGatewaySkuPropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var apiGateway = new ApiGateway("apiGateway", ApiGatewayArgs.builder()
* .backend(BackendConfigurationArgs.builder()
* .subnet(BackendSubnetConfigurationArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.Network/virtualNetworks/vn1/subnets/sn1")
* .build())
* .build())
* .gatewayName("apimGateway1")
* .location("South Central US")
* .resourceGroupName("rg1")
* .sku(ApiManagementGatewaySkuPropertiesArgs.builder()
* .capacity(1)
* .name("WorkspaceGatewayPremium")
* .build())
* .tags(Map.ofEntries(
* Map.entry("Name", "Contoso"),
* Map.entry("Test", "User")
* ))
* .virtualNetworkType("External")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:apimanagement:ApiGateway apimGateway1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/gateways/{gatewayName}
* ```
*
*/
@ResourceType(type="azure-native:apimanagement:ApiGateway")
public class ApiGateway extends com.pulumi.resources.CustomResource {
/**
* Information regarding how the gateway should integrate with backend systems.
*
*/
@Export(name="backend", refs={BackendConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ BackendConfigurationResponse> backend;
/**
* @return Information regarding how the gateway should integrate with backend systems.
*
*/
public Output> backend() {
return Codegen.optional(this.backend);
}
/**
* Information regarding the Configuration API of the API Management gateway. This is only applicable for API gateway with Standard SKU.
*
*/
@Export(name="configurationApi", refs={GatewayConfigurationApiResponse.class}, tree="[0]")
private Output* @Nullable */ GatewayConfigurationApiResponse> configurationApi;
/**
* @return Information regarding the Configuration API of the API Management gateway. This is only applicable for API gateway with Standard SKU.
*
*/
public Output> configurationApi() {
return Codegen.optional(this.configurationApi);
}
/**
* Creation UTC date of the API Management gateway.The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
@Export(name="createdAtUtc", refs={String.class}, tree="[0]")
private Output createdAtUtc;
/**
* @return Creation UTC date of the API Management gateway.The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Output createdAtUtc() {
return this.createdAtUtc;
}
/**
* ETag of the resource.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return ETag of the resource.
*
*/
public Output etag() {
return this.etag;
}
/**
* Information regarding how the gateway should be exposed.
*
*/
@Export(name="frontend", refs={FrontendConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ FrontendConfigurationResponse> frontend;
/**
* @return Information regarding how the gateway should be exposed.
*
*/
public Output> frontend() {
return Codegen.optional(this.frontend);
}
/**
* Resource location.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location.
*
*/
public Output location() {
return this.location;
}
/**
* Resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name.
*
*/
public Output name() {
return this.name;
}
/**
* The current provisioning state of the API Management gateway which can be one of the following: Created/Activating/Succeeded/Updating/Failed/Stopped/Terminating/TerminationFailed/Deleted.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The current provisioning state of the API Management gateway which can be one of the following: Created/Activating/Succeeded/Updating/Failed/Stopped/Terminating/TerminationFailed/Deleted.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* SKU properties of the API Management gateway.
*
*/
@Export(name="sku", refs={ApiManagementGatewaySkuPropertiesResponse.class}, tree="[0]")
private Output sku;
/**
* @return SKU properties of the API Management gateway.
*
*/
public Output sku() {
return this.sku;
}
/**
* Metadata pertaining to creation and last modification of the resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The provisioning state of the API Management gateway, which is targeted by the long running operation started on the gateway.
*
*/
@Export(name="targetProvisioningState", refs={String.class}, tree="[0]")
private Output targetProvisioningState;
/**
* @return The provisioning state of the API Management gateway, which is targeted by the long running operation started on the gateway.
*
*/
public Output targetProvisioningState() {
return this.targetProvisioningState;
}
/**
* Resource type for API Management resource is set to Microsoft.ApiManagement.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type for API Management resource is set to Microsoft.ApiManagement.
*
*/
public Output type() {
return this.type;
}
/**
* The type of VPN in which API Management gateway needs to be configured in.
*
*/
@Export(name="virtualNetworkType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> virtualNetworkType;
/**
* @return The type of VPN in which API Management gateway needs to be configured in.
*
*/
public Output> virtualNetworkType() {
return Codegen.optional(this.virtualNetworkType);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ApiGateway(java.lang.String name) {
this(name, ApiGatewayArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ApiGateway(java.lang.String name, ApiGatewayArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ApiGateway(java.lang.String name, ApiGatewayArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:ApiGateway", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ApiGateway(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:ApiGateway", name, null, makeResourceOptions(options, id), false);
}
private static ApiGatewayArgs makeArgs(ApiGatewayArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ApiGatewayArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:apimanagement/v20230901preview:ApiGateway").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20240501:ApiGateway").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ApiGateway get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ApiGateway(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy