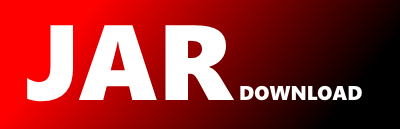
com.pulumi.azurenative.apimanagement.IdentityProviderArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.apimanagement.enums.IdentityProviderType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class IdentityProviderArgs extends com.pulumi.resources.ResourceArgs {
public static final IdentityProviderArgs Empty = new IdentityProviderArgs();
/**
* List of Allowed Tenants when configuring Azure Active Directory login.
*
*/
@Import(name="allowedTenants")
private @Nullable Output> allowedTenants;
/**
* @return List of Allowed Tenants when configuring Azure Active Directory login.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy