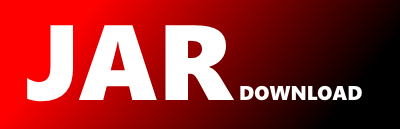
com.pulumi.azurenative.apimanagement.outputs.GetSubscriptionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetSubscriptionResult {
/**
* @return Determines whether tracing is enabled
*
*/
private @Nullable Boolean allowTracing;
/**
* @return Subscription creation date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
private String createdDate;
/**
* @return The name of the subscription, or null if the subscription has no name.
*
*/
private @Nullable String displayName;
/**
* @return Date when subscription was cancelled or expired. The setting is for audit purposes only and the subscription is not automatically cancelled. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
private @Nullable String endDate;
/**
* @return Subscription expiration date. The setting is for audit purposes only and the subscription is not automatically expired. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
private @Nullable String expirationDate;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Upcoming subscription expiration notification date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
private @Nullable String notificationDate;
/**
* @return The user resource identifier of the subscription owner. The value is a valid relative URL in the format of /users/{userId} where {userId} is a user identifier.
*
*/
private @Nullable String ownerId;
/**
* @return Subscription primary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
private @Nullable String primaryKey;
/**
* @return Scope like /products/{productId} or /apis or /apis/{apiId}.
*
*/
private String scope;
/**
* @return Subscription secondary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
private @Nullable String secondaryKey;
/**
* @return Subscription activation date. The setting is for audit purposes only and the subscription is not automatically activated. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
private @Nullable String startDate;
/**
* @return Subscription state. Possible states are * active – the subscription is active, * suspended – the subscription is blocked, and the subscriber cannot call any APIs of the product, * submitted – the subscription request has been made by the developer, but has not yet been approved or rejected, * rejected – the subscription request has been denied by an administrator, * cancelled – the subscription has been cancelled by the developer or administrator, * expired – the subscription reached its expiration date and was deactivated.
*
*/
private String state;
/**
* @return Optional subscription comment added by an administrator when the state is changed to the 'rejected'.
*
*/
private @Nullable String stateComment;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetSubscriptionResult() {}
/**
* @return Determines whether tracing is enabled
*
*/
public Optional allowTracing() {
return Optional.ofNullable(this.allowTracing);
}
/**
* @return Subscription creation date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public String createdDate() {
return this.createdDate;
}
/**
* @return The name of the subscription, or null if the subscription has no name.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return Date when subscription was cancelled or expired. The setting is for audit purposes only and the subscription is not automatically cancelled. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Optional endDate() {
return Optional.ofNullable(this.endDate);
}
/**
* @return Subscription expiration date. The setting is for audit purposes only and the subscription is not automatically expired. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Optional expirationDate() {
return Optional.ofNullable(this.expirationDate);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Upcoming subscription expiration notification date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Optional notificationDate() {
return Optional.ofNullable(this.notificationDate);
}
/**
* @return The user resource identifier of the subscription owner. The value is a valid relative URL in the format of /users/{userId} where {userId} is a user identifier.
*
*/
public Optional ownerId() {
return Optional.ofNullable(this.ownerId);
}
/**
* @return Subscription primary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
public Optional primaryKey() {
return Optional.ofNullable(this.primaryKey);
}
/**
* @return Scope like /products/{productId} or /apis or /apis/{apiId}.
*
*/
public String scope() {
return this.scope;
}
/**
* @return Subscription secondary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
public Optional secondaryKey() {
return Optional.ofNullable(this.secondaryKey);
}
/**
* @return Subscription activation date. The setting is for audit purposes only and the subscription is not automatically activated. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Optional startDate() {
return Optional.ofNullable(this.startDate);
}
/**
* @return Subscription state. Possible states are * active – the subscription is active, * suspended – the subscription is blocked, and the subscriber cannot call any APIs of the product, * submitted – the subscription request has been made by the developer, but has not yet been approved or rejected, * rejected – the subscription request has been denied by an administrator, * cancelled – the subscription has been cancelled by the developer or administrator, * expired – the subscription reached its expiration date and was deactivated.
*
*/
public String state() {
return this.state;
}
/**
* @return Optional subscription comment added by an administrator when the state is changed to the 'rejected'.
*
*/
public Optional stateComment() {
return Optional.ofNullable(this.stateComment);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetSubscriptionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowTracing;
private String createdDate;
private @Nullable String displayName;
private @Nullable String endDate;
private @Nullable String expirationDate;
private String id;
private String name;
private @Nullable String notificationDate;
private @Nullable String ownerId;
private @Nullable String primaryKey;
private String scope;
private @Nullable String secondaryKey;
private @Nullable String startDate;
private String state;
private @Nullable String stateComment;
private String type;
public Builder() {}
public Builder(GetSubscriptionResult defaults) {
Objects.requireNonNull(defaults);
this.allowTracing = defaults.allowTracing;
this.createdDate = defaults.createdDate;
this.displayName = defaults.displayName;
this.endDate = defaults.endDate;
this.expirationDate = defaults.expirationDate;
this.id = defaults.id;
this.name = defaults.name;
this.notificationDate = defaults.notificationDate;
this.ownerId = defaults.ownerId;
this.primaryKey = defaults.primaryKey;
this.scope = defaults.scope;
this.secondaryKey = defaults.secondaryKey;
this.startDate = defaults.startDate;
this.state = defaults.state;
this.stateComment = defaults.stateComment;
this.type = defaults.type;
}
@CustomType.Setter
public Builder allowTracing(@Nullable Boolean allowTracing) {
this.allowTracing = allowTracing;
return this;
}
@CustomType.Setter
public Builder createdDate(String createdDate) {
if (createdDate == null) {
throw new MissingRequiredPropertyException("GetSubscriptionResult", "createdDate");
}
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder endDate(@Nullable String endDate) {
this.endDate = endDate;
return this;
}
@CustomType.Setter
public Builder expirationDate(@Nullable String expirationDate) {
this.expirationDate = expirationDate;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetSubscriptionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetSubscriptionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder notificationDate(@Nullable String notificationDate) {
this.notificationDate = notificationDate;
return this;
}
@CustomType.Setter
public Builder ownerId(@Nullable String ownerId) {
this.ownerId = ownerId;
return this;
}
@CustomType.Setter
public Builder primaryKey(@Nullable String primaryKey) {
this.primaryKey = primaryKey;
return this;
}
@CustomType.Setter
public Builder scope(String scope) {
if (scope == null) {
throw new MissingRequiredPropertyException("GetSubscriptionResult", "scope");
}
this.scope = scope;
return this;
}
@CustomType.Setter
public Builder secondaryKey(@Nullable String secondaryKey) {
this.secondaryKey = secondaryKey;
return this;
}
@CustomType.Setter
public Builder startDate(@Nullable String startDate) {
this.startDate = startDate;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetSubscriptionResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder stateComment(@Nullable String stateComment) {
this.stateComment = stateComment;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetSubscriptionResult", "type");
}
this.type = type;
return this;
}
public GetSubscriptionResult build() {
final var _resultValue = new GetSubscriptionResult();
_resultValue.allowTracing = allowTracing;
_resultValue.createdDate = createdDate;
_resultValue.displayName = displayName;
_resultValue.endDate = endDate;
_resultValue.expirationDate = expirationDate;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.notificationDate = notificationDate;
_resultValue.ownerId = ownerId;
_resultValue.primaryKey = primaryKey;
_resultValue.scope = scope;
_resultValue.secondaryKey = secondaryKey;
_resultValue.startDate = startDate;
_resultValue.state = state;
_resultValue.stateComment = stateComment;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy