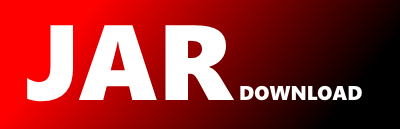
com.pulumi.azurenative.apimanagement.outputs.HostnameConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement.outputs;
import com.pulumi.azurenative.apimanagement.outputs.CertificateInformationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class HostnameConfigurationResponse {
/**
* @return Certificate information.
*
*/
private @Nullable CertificateInformationResponse certificate;
/**
* @return Certificate Password.
*
*/
private @Nullable String certificatePassword;
/**
* @return Certificate Source.
*
*/
private @Nullable String certificateSource;
/**
* @return Certificate Status.
*
*/
private @Nullable String certificateStatus;
/**
* @return Specify true to setup the certificate associated with this Hostname as the Default SSL Certificate. If a client does not send the SNI header, then this will be the certificate that will be challenged. The property is useful if a service has multiple custom hostname enabled and it needs to decide on the default ssl certificate. The setting only applied to gateway Hostname Type.
*
*/
private @Nullable Boolean defaultSslBinding;
/**
* @return Base64 Encoded certificate.
*
*/
private @Nullable String encodedCertificate;
/**
* @return Hostname to configure on the Api Management service.
*
*/
private String hostName;
/**
* @return System or User Assigned Managed identity clientId as generated by Azure AD, which has GET access to the keyVault containing the SSL certificate.
*
*/
private @Nullable String identityClientId;
/**
* @return Url to the KeyVault Secret containing the Ssl Certificate. If absolute Url containing version is provided, auto-update of ssl certificate will not work. This requires Api Management service to be configured with aka.ms/apimmsi. The secret should be of type *application/x-pkcs12*
*
*/
private @Nullable String keyVaultId;
/**
* @return Specify true to always negotiate client certificate on the hostname. Default Value is false.
*
*/
private @Nullable Boolean negotiateClientCertificate;
/**
* @return Hostname type.
*
*/
private String type;
private HostnameConfigurationResponse() {}
/**
* @return Certificate information.
*
*/
public Optional certificate() {
return Optional.ofNullable(this.certificate);
}
/**
* @return Certificate Password.
*
*/
public Optional certificatePassword() {
return Optional.ofNullable(this.certificatePassword);
}
/**
* @return Certificate Source.
*
*/
public Optional certificateSource() {
return Optional.ofNullable(this.certificateSource);
}
/**
* @return Certificate Status.
*
*/
public Optional certificateStatus() {
return Optional.ofNullable(this.certificateStatus);
}
/**
* @return Specify true to setup the certificate associated with this Hostname as the Default SSL Certificate. If a client does not send the SNI header, then this will be the certificate that will be challenged. The property is useful if a service has multiple custom hostname enabled and it needs to decide on the default ssl certificate. The setting only applied to gateway Hostname Type.
*
*/
public Optional defaultSslBinding() {
return Optional.ofNullable(this.defaultSslBinding);
}
/**
* @return Base64 Encoded certificate.
*
*/
public Optional encodedCertificate() {
return Optional.ofNullable(this.encodedCertificate);
}
/**
* @return Hostname to configure on the Api Management service.
*
*/
public String hostName() {
return this.hostName;
}
/**
* @return System or User Assigned Managed identity clientId as generated by Azure AD, which has GET access to the keyVault containing the SSL certificate.
*
*/
public Optional identityClientId() {
return Optional.ofNullable(this.identityClientId);
}
/**
* @return Url to the KeyVault Secret containing the Ssl Certificate. If absolute Url containing version is provided, auto-update of ssl certificate will not work. This requires Api Management service to be configured with aka.ms/apimmsi. The secret should be of type *application/x-pkcs12*
*
*/
public Optional keyVaultId() {
return Optional.ofNullable(this.keyVaultId);
}
/**
* @return Specify true to always negotiate client certificate on the hostname. Default Value is false.
*
*/
public Optional negotiateClientCertificate() {
return Optional.ofNullable(this.negotiateClientCertificate);
}
/**
* @return Hostname type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HostnameConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable CertificateInformationResponse certificate;
private @Nullable String certificatePassword;
private @Nullable String certificateSource;
private @Nullable String certificateStatus;
private @Nullable Boolean defaultSslBinding;
private @Nullable String encodedCertificate;
private String hostName;
private @Nullable String identityClientId;
private @Nullable String keyVaultId;
private @Nullable Boolean negotiateClientCertificate;
private String type;
public Builder() {}
public Builder(HostnameConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.certificate = defaults.certificate;
this.certificatePassword = defaults.certificatePassword;
this.certificateSource = defaults.certificateSource;
this.certificateStatus = defaults.certificateStatus;
this.defaultSslBinding = defaults.defaultSslBinding;
this.encodedCertificate = defaults.encodedCertificate;
this.hostName = defaults.hostName;
this.identityClientId = defaults.identityClientId;
this.keyVaultId = defaults.keyVaultId;
this.negotiateClientCertificate = defaults.negotiateClientCertificate;
this.type = defaults.type;
}
@CustomType.Setter
public Builder certificate(@Nullable CertificateInformationResponse certificate) {
this.certificate = certificate;
return this;
}
@CustomType.Setter
public Builder certificatePassword(@Nullable String certificatePassword) {
this.certificatePassword = certificatePassword;
return this;
}
@CustomType.Setter
public Builder certificateSource(@Nullable String certificateSource) {
this.certificateSource = certificateSource;
return this;
}
@CustomType.Setter
public Builder certificateStatus(@Nullable String certificateStatus) {
this.certificateStatus = certificateStatus;
return this;
}
@CustomType.Setter
public Builder defaultSslBinding(@Nullable Boolean defaultSslBinding) {
this.defaultSslBinding = defaultSslBinding;
return this;
}
@CustomType.Setter
public Builder encodedCertificate(@Nullable String encodedCertificate) {
this.encodedCertificate = encodedCertificate;
return this;
}
@CustomType.Setter
public Builder hostName(String hostName) {
if (hostName == null) {
throw new MissingRequiredPropertyException("HostnameConfigurationResponse", "hostName");
}
this.hostName = hostName;
return this;
}
@CustomType.Setter
public Builder identityClientId(@Nullable String identityClientId) {
this.identityClientId = identityClientId;
return this;
}
@CustomType.Setter
public Builder keyVaultId(@Nullable String keyVaultId) {
this.keyVaultId = keyVaultId;
return this;
}
@CustomType.Setter
public Builder negotiateClientCertificate(@Nullable Boolean negotiateClientCertificate) {
this.negotiateClientCertificate = negotiateClientCertificate;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("HostnameConfigurationResponse", "type");
}
this.type = type;
return this;
}
public HostnameConfigurationResponse build() {
final var _resultValue = new HostnameConfigurationResponse();
_resultValue.certificate = certificate;
_resultValue.certificatePassword = certificatePassword;
_resultValue.certificateSource = certificateSource;
_resultValue.certificateStatus = certificateStatus;
_resultValue.defaultSslBinding = defaultSslBinding;
_resultValue.encodedCertificate = encodedCertificate;
_resultValue.hostName = hostName;
_resultValue.identityClientId = identityClientId;
_resultValue.keyVaultId = keyVaultId;
_resultValue.negotiateClientCertificate = negotiateClientCertificate;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy