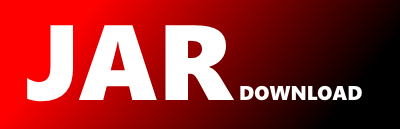
com.pulumi.azurenative.app.inputs.GithubActionConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.inputs;
import com.pulumi.azurenative.app.inputs.AzureCredentialsArgs;
import com.pulumi.azurenative.app.inputs.RegistryInfoArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Configuration properties that define the mutable settings of a Container App SourceControl
*
*/
public final class GithubActionConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final GithubActionConfigurationArgs Empty = new GithubActionConfigurationArgs();
/**
* AzureCredentials configurations.
*
*/
@Import(name="azureCredentials")
private @Nullable Output azureCredentials;
/**
* @return AzureCredentials configurations.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy