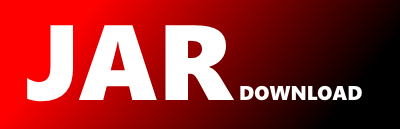
com.pulumi.azurenative.appconfiguration.ConfigurationStoreArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appconfiguration;
import com.pulumi.azurenative.appconfiguration.enums.CreateMode;
import com.pulumi.azurenative.appconfiguration.enums.PublicNetworkAccess;
import com.pulumi.azurenative.appconfiguration.inputs.EncryptionPropertiesArgs;
import com.pulumi.azurenative.appconfiguration.inputs.ResourceIdentityArgs;
import com.pulumi.azurenative.appconfiguration.inputs.SkuArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ConfigurationStoreArgs extends com.pulumi.resources.ResourceArgs {
public static final ConfigurationStoreArgs Empty = new ConfigurationStoreArgs();
/**
* The name of the configuration store.
*
*/
@Import(name="configStoreName")
private @Nullable Output configStoreName;
/**
* @return The name of the configuration store.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy