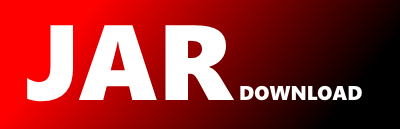
com.pulumi.azurenative.appplatform.inputs.GatewayPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appplatform.inputs;
import com.pulumi.azurenative.appplatform.enums.ApmType;
import com.pulumi.azurenative.appplatform.inputs.GatewayApiMetadataPropertiesArgs;
import com.pulumi.azurenative.appplatform.inputs.GatewayCorsPropertiesArgs;
import com.pulumi.azurenative.appplatform.inputs.GatewayPropertiesClientAuthArgs;
import com.pulumi.azurenative.appplatform.inputs.GatewayPropertiesEnvironmentVariablesArgs;
import com.pulumi.azurenative.appplatform.inputs.GatewayResourceRequestsArgs;
import com.pulumi.azurenative.appplatform.inputs.SsoPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Spring Cloud Gateway properties payload
*
*/
public final class GatewayPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final GatewayPropertiesArgs Empty = new GatewayPropertiesArgs();
/**
* Collection of addons for Spring Cloud Gateway
*
*/
@Import(name="addonConfigs")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy