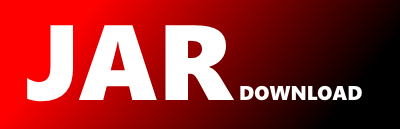
com.pulumi.azurenative.authorization.AccessReviewHistoryDefinitionById Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.authorization.AccessReviewHistoryDefinitionByIdArgs;
import com.pulumi.azurenative.authorization.outputs.AccessReviewHistoryInstanceResponse;
import com.pulumi.azurenative.authorization.outputs.AccessReviewScopeResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Access Review History Definition.
* Azure REST API version: 2021-12-01-preview. Prior API version in Azure Native 1.x: 2021-11-16-preview.
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:authorization:AccessReviewHistoryDefinitionById myresource1 /subscriptions/{subscriptionId}/providers/Microsoft.Authorization/accessReviewHistoryDefinitions/{historyDefinitionId}
* ```
*
*/
@ResourceType(type="azure-native:authorization:AccessReviewHistoryDefinitionById")
public class AccessReviewHistoryDefinitionById extends com.pulumi.resources.CustomResource {
/**
* Date time when history definition was created
*
*/
@Export(name="createdDateTime", refs={String.class}, tree="[0]")
private Output createdDateTime;
/**
* @return Date time when history definition was created
*
*/
public Output createdDateTime() {
return this.createdDateTime;
}
/**
* Collection of review decisions which the history data should be filtered on. For example if Approve and Deny are supplied the data will only contain review results in which the decision maker approved or denied a review request.
*
*/
@Export(name="decisions", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> decisions;
/**
* @return Collection of review decisions which the history data should be filtered on. For example if Approve and Deny are supplied the data will only contain review results in which the decision maker approved or denied a review request.
*
*/
public Output>> decisions() {
return Codegen.optional(this.decisions);
}
/**
* The display name for the history definition.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return The display name for the history definition.
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* The DateTime when the review is scheduled to end. Required if type is endDate
*
*/
@Export(name="endDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> endDate;
/**
* @return The DateTime when the review is scheduled to end. Required if type is endDate
*
*/
public Output> endDate() {
return Codegen.optional(this.endDate);
}
/**
* Set of access review history instances for this history definition.
*
*/
@Export(name="instances", refs={List.class,AccessReviewHistoryInstanceResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> instances;
/**
* @return Set of access review history instances for this history definition.
*
*/
public Output>> instances() {
return Codegen.optional(this.instances);
}
/**
* The interval for recurrence. For a quarterly review, the interval is 3 for type : absoluteMonthly.
*
*/
@Export(name="interval", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> interval;
/**
* @return The interval for recurrence. For a quarterly review, the interval is 3 for type : absoluteMonthly.
*
*/
public Output> interval() {
return Codegen.optional(this.interval);
}
/**
* The access review history definition unique id.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The access review history definition unique id.
*
*/
public Output name() {
return this.name;
}
/**
* The number of times to repeat the access review. Required and must be positive if type is numbered.
*
*/
@Export(name="numberOfOccurrences", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> numberOfOccurrences;
/**
* @return The number of times to repeat the access review. Required and must be positive if type is numbered.
*
*/
public Output> numberOfOccurrences() {
return Codegen.optional(this.numberOfOccurrences);
}
/**
* The identity id
*
*/
@Export(name="principalId", refs={String.class}, tree="[0]")
private Output principalId;
/**
* @return The identity id
*
*/
public Output principalId() {
return this.principalId;
}
/**
* The identity display name
*
*/
@Export(name="principalName", refs={String.class}, tree="[0]")
private Output principalName;
/**
* @return The identity display name
*
*/
public Output principalName() {
return this.principalName;
}
/**
* The identity type : user/servicePrincipal
*
*/
@Export(name="principalType", refs={String.class}, tree="[0]")
private Output principalType;
/**
* @return The identity type : user/servicePrincipal
*
*/
public Output principalType() {
return this.principalType;
}
/**
* Date time used when selecting review data, all reviews included in data end on or before this date. For use only with one-time/non-recurring reports.
*
*/
@Export(name="reviewHistoryPeriodEndDateTime", refs={String.class}, tree="[0]")
private Output reviewHistoryPeriodEndDateTime;
/**
* @return Date time used when selecting review data, all reviews included in data end on or before this date. For use only with one-time/non-recurring reports.
*
*/
public Output reviewHistoryPeriodEndDateTime() {
return this.reviewHistoryPeriodEndDateTime;
}
/**
* Date time used when selecting review data, all reviews included in data start on or after this date. For use only with one-time/non-recurring reports.
*
*/
@Export(name="reviewHistoryPeriodStartDateTime", refs={String.class}, tree="[0]")
private Output reviewHistoryPeriodStartDateTime;
/**
* @return Date time used when selecting review data, all reviews included in data start on or after this date. For use only with one-time/non-recurring reports.
*
*/
public Output reviewHistoryPeriodStartDateTime() {
return this.reviewHistoryPeriodStartDateTime;
}
/**
* A collection of scopes used when selecting review history data
*
*/
@Export(name="scopes", refs={List.class,AccessReviewScopeResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> scopes;
/**
* @return A collection of scopes used when selecting review history data
*
*/
public Output>> scopes() {
return Codegen.optional(this.scopes);
}
/**
* The DateTime when the review is scheduled to be start. This could be a date in the future. Required on create.
*
*/
@Export(name="startDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> startDate;
/**
* @return The DateTime when the review is scheduled to be start. This could be a date in the future. Required on create.
*
*/
public Output> startDate() {
return Codegen.optional(this.startDate);
}
/**
* This read-only field specifies the of the requested review history data. This is either requested, in-progress, done or error.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return This read-only field specifies the of the requested review history data. This is either requested, in-progress, done or error.
*
*/
public Output status() {
return this.status;
}
/**
* The resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The resource type.
*
*/
public Output type() {
return this.type;
}
/**
* The user principal name(if valid)
*
*/
@Export(name="userPrincipalName", refs={String.class}, tree="[0]")
private Output userPrincipalName;
/**
* @return The user principal name(if valid)
*
*/
public Output userPrincipalName() {
return this.userPrincipalName;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AccessReviewHistoryDefinitionById(java.lang.String name) {
this(name, AccessReviewHistoryDefinitionByIdArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AccessReviewHistoryDefinitionById(java.lang.String name, @Nullable AccessReviewHistoryDefinitionByIdArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AccessReviewHistoryDefinitionById(java.lang.String name, @Nullable AccessReviewHistoryDefinitionByIdArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:authorization:AccessReviewHistoryDefinitionById", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AccessReviewHistoryDefinitionById(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:authorization:AccessReviewHistoryDefinitionById", name, null, makeResourceOptions(options, id), false);
}
private static AccessReviewHistoryDefinitionByIdArgs makeArgs(@Nullable AccessReviewHistoryDefinitionByIdArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AccessReviewHistoryDefinitionByIdArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:authorization/v20211116preview:AccessReviewHistoryDefinitionById").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20211201preview:AccessReviewHistoryDefinitionById").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AccessReviewHistoryDefinitionById get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AccessReviewHistoryDefinitionById(name, id, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy