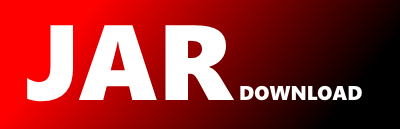
com.pulumi.azurenative.authorization.AccessReviewHistoryDefinitionByIdArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization;
import com.pulumi.azurenative.authorization.enums.AccessReviewRecurrenceRangeType;
import com.pulumi.azurenative.authorization.enums.AccessReviewResult;
import com.pulumi.azurenative.authorization.inputs.AccessReviewHistoryInstanceArgs;
import com.pulumi.azurenative.authorization.inputs.AccessReviewScopeArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AccessReviewHistoryDefinitionByIdArgs extends com.pulumi.resources.ResourceArgs {
public static final AccessReviewHistoryDefinitionByIdArgs Empty = new AccessReviewHistoryDefinitionByIdArgs();
/**
* Collection of review decisions which the history data should be filtered on. For example if Approve and Deny are supplied the data will only contain review results in which the decision maker approved or denied a review request.
*
*/
@Import(name="decisions")
private @Nullable Output>> decisions;
/**
* @return Collection of review decisions which the history data should be filtered on. For example if Approve and Deny are supplied the data will only contain review results in which the decision maker approved or denied a review request.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy