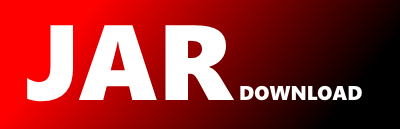
com.pulumi.azurenative.authorization.inputs.AccessReviewScopeArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Descriptor for what needs to be reviewed
*
*/
public final class AccessReviewScopeArgs extends com.pulumi.resources.ResourceArgs {
public static final AccessReviewScopeArgs Empty = new AccessReviewScopeArgs();
/**
* This is used to indicate the resource id(s) to exclude
*
*/
@Import(name="excludeResourceId")
private @Nullable Output excludeResourceId;
/**
* @return This is used to indicate the resource id(s) to exclude
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy