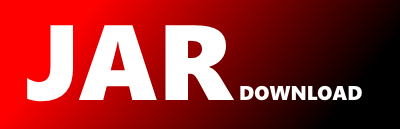
com.pulumi.azurenative.authorization.outputs.GetRoleDefinitionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization.outputs;
import com.pulumi.azurenative.authorization.outputs.PermissionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetRoleDefinitionResult {
/**
* @return Role definition assignable scopes.
*
*/
private @Nullable List assignableScopes;
/**
* @return Id of the user who created the assignment
*
*/
private String createdBy;
/**
* @return Time it was created
*
*/
private String createdOn;
/**
* @return The role definition description.
*
*/
private @Nullable String description;
/**
* @return The role definition ID.
*
*/
private String id;
/**
* @return The role definition name.
*
*/
private String name;
/**
* @return Role definition permissions.
*
*/
private @Nullable List permissions;
/**
* @return The role name.
*
*/
private @Nullable String roleName;
/**
* @return The role type.
*
*/
private @Nullable String roleType;
/**
* @return The role definition type.
*
*/
private String type;
/**
* @return Id of the user who updated the assignment
*
*/
private String updatedBy;
/**
* @return Time it was updated
*
*/
private String updatedOn;
private GetRoleDefinitionResult() {}
/**
* @return Role definition assignable scopes.
*
*/
public List assignableScopes() {
return this.assignableScopes == null ? List.of() : this.assignableScopes;
}
/**
* @return Id of the user who created the assignment
*
*/
public String createdBy() {
return this.createdBy;
}
/**
* @return Time it was created
*
*/
public String createdOn() {
return this.createdOn;
}
/**
* @return The role definition description.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The role definition ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The role definition name.
*
*/
public String name() {
return this.name;
}
/**
* @return Role definition permissions.
*
*/
public List permissions() {
return this.permissions == null ? List.of() : this.permissions;
}
/**
* @return The role name.
*
*/
public Optional roleName() {
return Optional.ofNullable(this.roleName);
}
/**
* @return The role type.
*
*/
public Optional roleType() {
return Optional.ofNullable(this.roleType);
}
/**
* @return The role definition type.
*
*/
public String type() {
return this.type;
}
/**
* @return Id of the user who updated the assignment
*
*/
public String updatedBy() {
return this.updatedBy;
}
/**
* @return Time it was updated
*
*/
public String updatedOn() {
return this.updatedOn;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRoleDefinitionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List assignableScopes;
private String createdBy;
private String createdOn;
private @Nullable String description;
private String id;
private String name;
private @Nullable List permissions;
private @Nullable String roleName;
private @Nullable String roleType;
private String type;
private String updatedBy;
private String updatedOn;
public Builder() {}
public Builder(GetRoleDefinitionResult defaults) {
Objects.requireNonNull(defaults);
this.assignableScopes = defaults.assignableScopes;
this.createdBy = defaults.createdBy;
this.createdOn = defaults.createdOn;
this.description = defaults.description;
this.id = defaults.id;
this.name = defaults.name;
this.permissions = defaults.permissions;
this.roleName = defaults.roleName;
this.roleType = defaults.roleType;
this.type = defaults.type;
this.updatedBy = defaults.updatedBy;
this.updatedOn = defaults.updatedOn;
}
@CustomType.Setter
public Builder assignableScopes(@Nullable List assignableScopes) {
this.assignableScopes = assignableScopes;
return this;
}
public Builder assignableScopes(String... assignableScopes) {
return assignableScopes(List.of(assignableScopes));
}
@CustomType.Setter
public Builder createdBy(String createdBy) {
if (createdBy == null) {
throw new MissingRequiredPropertyException("GetRoleDefinitionResult", "createdBy");
}
this.createdBy = createdBy;
return this;
}
@CustomType.Setter
public Builder createdOn(String createdOn) {
if (createdOn == null) {
throw new MissingRequiredPropertyException("GetRoleDefinitionResult", "createdOn");
}
this.createdOn = createdOn;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetRoleDefinitionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetRoleDefinitionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder permissions(@Nullable List permissions) {
this.permissions = permissions;
return this;
}
public Builder permissions(PermissionResponse... permissions) {
return permissions(List.of(permissions));
}
@CustomType.Setter
public Builder roleName(@Nullable String roleName) {
this.roleName = roleName;
return this;
}
@CustomType.Setter
public Builder roleType(@Nullable String roleType) {
this.roleType = roleType;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetRoleDefinitionResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder updatedBy(String updatedBy) {
if (updatedBy == null) {
throw new MissingRequiredPropertyException("GetRoleDefinitionResult", "updatedBy");
}
this.updatedBy = updatedBy;
return this;
}
@CustomType.Setter
public Builder updatedOn(String updatedOn) {
if (updatedOn == null) {
throw new MissingRequiredPropertyException("GetRoleDefinitionResult", "updatedOn");
}
this.updatedOn = updatedOn;
return this;
}
public GetRoleDefinitionResult build() {
final var _resultValue = new GetRoleDefinitionResult();
_resultValue.assignableScopes = assignableScopes;
_resultValue.createdBy = createdBy;
_resultValue.createdOn = createdOn;
_resultValue.description = description;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.permissions = permissions;
_resultValue.roleName = roleName;
_resultValue.roleType = roleType;
_resultValue.type = type;
_resultValue.updatedBy = updatedBy;
_resultValue.updatedOn = updatedOn;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy