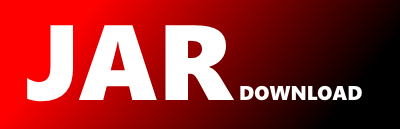
com.pulumi.azurenative.avs.inputs.IdentitySourceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.avs.inputs;
import com.pulumi.azurenative.avs.enums.SslEnum;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* vCenter Single Sign On Identity Source
*
*/
public final class IdentitySourceArgs extends com.pulumi.resources.ResourceArgs {
public static final IdentitySourceArgs Empty = new IdentitySourceArgs();
/**
* The domain's NetBIOS name
*
*/
@Import(name="alias")
private @Nullable Output alias;
/**
* @return The domain's NetBIOS name
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy