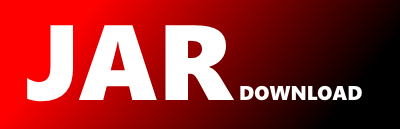
com.pulumi.azurenative.awsconnector.inputs.AwsElasticBeanstalkEnvironmentPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.inputs;
import com.pulumi.azurenative.awsconnector.enums.Tier;
import com.pulumi.azurenative.awsconnector.inputs.OptionSettingArgs;
import com.pulumi.azurenative.awsconnector.inputs.TagArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Definition of awsElasticBeanstalkEnvironment
*
*/
public final class AwsElasticBeanstalkEnvironmentPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final AwsElasticBeanstalkEnvironmentPropertiesArgs Empty = new AwsElasticBeanstalkEnvironmentPropertiesArgs();
/**
* The name of the application that is associated with this environment.
*
*/
@Import(name="applicationName")
private @Nullable Output applicationName;
/**
* @return The name of the application that is associated with this environment.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy