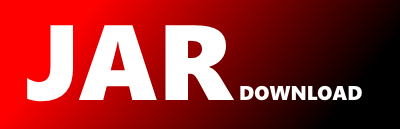
com.pulumi.azurenative.awsconnector.inputs.BlockDeviceMappingArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.inputs;
import com.pulumi.azurenative.awsconnector.inputs.EbsBlockDeviceArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Definition of BlockDeviceMapping
*
*/
public final class BlockDeviceMappingArgs extends com.pulumi.resources.ResourceArgs {
public static final BlockDeviceMappingArgs Empty = new BlockDeviceMappingArgs();
/**
* <p>The device name (for example, <code>/dev/sdh</code> or <code>xvdh</code>).</p>
*
*/
@Import(name="deviceName")
private @Nullable Output deviceName;
/**
* @return <p>The device name (for example, <code>/dev/sdh</code> or <code>xvdh</code>).</p>
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy