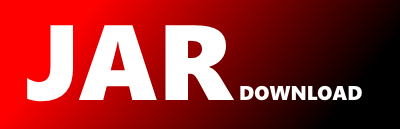
com.pulumi.azurenative.awsconnector.outputs.AwsRedshiftClusterPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.LoggingPropertiesResponse;
import com.pulumi.azurenative.awsconnector.outputs.RedshiftClusterEndpointResponse;
import com.pulumi.azurenative.awsconnector.outputs.TagResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AwsRedshiftClusterPropertiesResponse {
/**
* @return Major version upgrades can be applied during the maintenance window to the Amazon Redshift engine that is running on the cluster. Default value is True
*
*/
private @Nullable Boolean allowVersionUpgrade;
/**
* @return The value represents how the cluster is configured to use AQUA (Advanced Query Accelerator) after the cluster is restored. Possible values include the following.enabled - Use AQUA if it is available for the current Region and Amazon Redshift node type.disabled - Don't use AQUA.auto - Amazon Redshift determines whether to use AQUA.
*
*/
private @Nullable String aquaConfigurationStatus;
/**
* @return The number of days that automated snapshots are retained. If the value is 0, automated snapshots are disabled. Default value is 1
*
*/
private @Nullable Integer automatedSnapshotRetentionPeriod;
/**
* @return The EC2 Availability Zone (AZ) in which you want Amazon Redshift to provision the cluster. Default: A random, system-chosen Availability Zone in the region that is specified by the endpoint
*
*/
private @Nullable String availabilityZone;
/**
* @return The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the cluster modification is complete.
*
*/
private @Nullable Boolean availabilityZoneRelocation;
/**
* @return The availability zone relocation status of the cluster
*
*/
private @Nullable String availabilityZoneRelocationStatus;
/**
* @return A boolean value indicating whether the resize operation is using the classic resize process. If you don't provide this parameter or set the value to false , the resize type is elastic.
*
*/
private @Nullable Boolean classic;
/**
* @return A unique identifier for the cluster. You use this identifier to refer to the cluster for any subsequent cluster operations such as deleting or modifying. All alphabetical characters must be lower case, no hypens at the end, no two consecutive hyphens. Cluster name should be unique for all clusters within an AWS account
*
*/
private @Nullable String clusterIdentifier;
/**
* @return The Amazon Resource Name (ARN) of the cluster namespace.
*
*/
private @Nullable String clusterNamespaceArn;
/**
* @return The name of the parameter group to be associated with this cluster.
*
*/
private @Nullable String clusterParameterGroupName;
/**
* @return A list of security groups to be associated with this cluster.
*
*/
private @Nullable List clusterSecurityGroups;
/**
* @return The name of a cluster subnet group to be associated with this cluster.
*
*/
private @Nullable String clusterSubnetGroupName;
/**
* @return The type of the cluster. When cluster type is specified as single-node, the NumberOfNodes parameter is not required and if multi-node, the NumberOfNodes parameter is required
*
*/
private @Nullable String clusterType;
/**
* @return The version of the Amazon Redshift engine software that you want to deploy on the cluster.The version selected runs on all the nodes in the cluster.
*
*/
private @Nullable String clusterVersion;
/**
* @return The name of the first database to be created when the cluster is created. To create additional databases after the cluster is created, connect to the cluster with a SQL client and use SQL commands to create a database.
*
*/
private @Nullable String dbName;
/**
* @return A boolean indicating whether to enable the deferred maintenance window.
*
*/
private @Nullable Boolean deferMaintenance;
/**
* @return An integer indicating the duration of the maintenance window in days. If you specify a duration, you can't specify an end time. The duration must be 45 days or less.
*
*/
private @Nullable Integer deferMaintenanceDuration;
/**
* @return A timestamp indicating end time for the deferred maintenance window. If you specify an end time, you can't specify a duration.
*
*/
private @Nullable String deferMaintenanceEndTime;
/**
* @return A unique identifier for the deferred maintenance window.
*
*/
private @Nullable String deferMaintenanceIdentifier;
/**
* @return A timestamp indicating the start time for the deferred maintenance window.
*
*/
private @Nullable String deferMaintenanceStartTime;
/**
* @return The destination AWS Region that you want to copy snapshots to. Constraints: Must be the name of a valid AWS Region. For more information, see Regions and Endpoints in the Amazon Web Services [https://docs.aws.amazon.com/general/latest/gr/rande.html#redshift_region] General Reference
*
*/
private @Nullable String destinationRegion;
/**
* @return The Elastic IP (EIP) address for the cluster.
*
*/
private @Nullable String elasticIp;
/**
* @return If true, the data in the cluster is encrypted at rest.
*
*/
private @Nullable Boolean encrypted;
/**
* @return Property endpoint
*
*/
private @Nullable RedshiftClusterEndpointResponse endpoint;
/**
* @return An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a cluster that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC Routing in the Amazon Redshift Cluster Management Guide.If this option is true , enhanced VPC routing is enabled.Default: false
*
*/
private @Nullable Boolean enhancedVpcRouting;
/**
* @return Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data encryption keys stored in an HSM
*
*/
private @Nullable String hsmClientCertificateIdentifier;
/**
* @return Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster can use to retrieve and store keys in an HSM.
*
*/
private @Nullable String hsmConfigurationIdentifier;
/**
* @return A list of AWS Identity and Access Management (IAM) roles that can be used by the cluster to access other AWS services. You must supply the IAM roles in their Amazon Resource Name (ARN) format. You can supply up to 50 IAM roles in a single request
*
*/
private @Nullable List iamRoles;
/**
* @return The AWS Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in the cluster.
*
*/
private @Nullable String kmsKeyId;
/**
* @return Property loggingProperties
*
*/
private @Nullable LoggingPropertiesResponse loggingProperties;
/**
* @return The name for the maintenance track that you want to assign for the cluster. This name change is asynchronous. The new track name stays in the PendingModifiedValues for the cluster until the next maintenance window. When the maintenance track changes, the cluster is switched to the latest cluster release available for the maintenance track. At this point, the maintenance track name is applied.
*
*/
private @Nullable String maintenanceTrackName;
/**
* @return A boolean indicating if the redshift cluster's admin user credentials is managed by Redshift or not. You can't use MasterUserPassword if ManageMasterPassword is true. If ManageMasterPassword is false or not set, Amazon Redshift uses MasterUserPassword for the admin user account's password.
*
*/
private @Nullable Boolean manageMasterPassword;
/**
* @return The number of days to retain newly copied snapshots in the destination AWS Region after they are copied from the source AWS Region. If the value is -1, the manual snapshot is retained indefinitely.The value must be either -1 or an integer between 1 and 3,653.
*
*/
private @Nullable Integer manualSnapshotRetentionPeriod;
/**
* @return The Amazon Resource Name (ARN) for the cluster's admin user credentials secret.
*
*/
private @Nullable String masterPasswordSecretArn;
/**
* @return The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin user credentials secret.
*
*/
private @Nullable String masterPasswordSecretKmsKeyId;
/**
* @return The password associated with the master user account for the cluster that is being created. You can't use MasterUserPassword if ManageMasterPassword is true. Password must be between 8 and 64 characters in length, should have at least one uppercase letter.Must contain at least one lowercase letter.Must contain one number.Can be any printable ASCII character.
*
*/
private @Nullable String masterUserPassword;
/**
* @return The user name associated with the master user account for the cluster that is being created. The user name can't be PUBLIC and first character must be a letter.
*
*/
private @Nullable String masterUsername;
/**
* @return A boolean indicating if the redshift cluster is multi-az or not. If you don't provide this parameter or set the value to false, the redshift cluster will be single-az.
*
*/
private @Nullable Boolean multiAZ;
/**
* @return The namespace resource policy document that will be attached to a Redshift cluster.
*
*/
private @Nullable Object namespaceResourcePolicy;
/**
* @return The node type to be provisioned for the cluster.Valid Values: ds2.xlarge | ds2.8xlarge | dc1.large | dc1.8xlarge | dc2.large | dc2.8xlarge | ra3.4xlarge | ra3.16xlarge
*
*/
private @Nullable String nodeType;
/**
* @return The number of compute nodes in the cluster. This parameter is required when the ClusterType parameter is specified as multi-node.
*
*/
private @Nullable Integer numberOfNodes;
/**
* @return Property ownerAccount
*
*/
private @Nullable String ownerAccount;
/**
* @return The port number on which the cluster accepts incoming connections. The cluster is accessible only via the JDBC and ODBC connection strings
*
*/
private @Nullable Integer port;
/**
* @return The weekly time range (in UTC) during which automated cluster maintenance can occur.
*
*/
private @Nullable String preferredMaintenanceWindow;
/**
* @return If true, the cluster can be accessed from a public network.
*
*/
private @Nullable Boolean publiclyAccessible;
/**
* @return The Redshift operation to be performed. Resource Action supports pause-cluster, resume-cluster, failover-primary-compute APIs
*
*/
private @Nullable String resourceAction;
/**
* @return The identifier of the database revision. You can retrieve this value from the response to the DescribeClusterDbRevisions request.
*
*/
private @Nullable String revisionTarget;
/**
* @return A boolean indicating if we want to rotate Encryption Keys.
*
*/
private @Nullable Boolean rotateEncryptionKey;
/**
* @return The name of the cluster the source snapshot was created from. This parameter is required if your IAM user has a policy containing a snapshot resource element that specifies anything other than * for the cluster name.
*
*/
private @Nullable String snapshotClusterIdentifier;
/**
* @return The name of the snapshot copy grant to use when snapshots of an AWS KMS-encrypted cluster are copied to the destination region.
*
*/
private @Nullable String snapshotCopyGrantName;
/**
* @return Indicates whether to apply the snapshot retention period to newly copied manual snapshots instead of automated snapshots.
*
*/
private @Nullable Boolean snapshotCopyManual;
/**
* @return The number of days to retain automated snapshots in the destination region after they are copied from the source region. Default is 7. Constraints: Must be at least 1 and no more than 35.
*
*/
private @Nullable Integer snapshotCopyRetentionPeriod;
/**
* @return The name of the snapshot from which to create the new cluster. This parameter isn't case sensitive.
*
*/
private @Nullable String snapshotIdentifier;
/**
* @return The list of tags for the cluster parameter group.
*
*/
private @Nullable List tags;
/**
* @return A list of Virtual Private Cloud (VPC) security groups to be associated with the cluster.
*
*/
private @Nullable List vpcSecurityGroupIds;
private AwsRedshiftClusterPropertiesResponse() {}
/**
* @return Major version upgrades can be applied during the maintenance window to the Amazon Redshift engine that is running on the cluster. Default value is True
*
*/
public Optional allowVersionUpgrade() {
return Optional.ofNullable(this.allowVersionUpgrade);
}
/**
* @return The value represents how the cluster is configured to use AQUA (Advanced Query Accelerator) after the cluster is restored. Possible values include the following.enabled - Use AQUA if it is available for the current Region and Amazon Redshift node type.disabled - Don't use AQUA.auto - Amazon Redshift determines whether to use AQUA.
*
*/
public Optional aquaConfigurationStatus() {
return Optional.ofNullable(this.aquaConfigurationStatus);
}
/**
* @return The number of days that automated snapshots are retained. If the value is 0, automated snapshots are disabled. Default value is 1
*
*/
public Optional automatedSnapshotRetentionPeriod() {
return Optional.ofNullable(this.automatedSnapshotRetentionPeriod);
}
/**
* @return The EC2 Availability Zone (AZ) in which you want Amazon Redshift to provision the cluster. Default: A random, system-chosen Availability Zone in the region that is specified by the endpoint
*
*/
public Optional availabilityZone() {
return Optional.ofNullable(this.availabilityZone);
}
/**
* @return The option to enable relocation for an Amazon Redshift cluster between Availability Zones after the cluster modification is complete.
*
*/
public Optional availabilityZoneRelocation() {
return Optional.ofNullable(this.availabilityZoneRelocation);
}
/**
* @return The availability zone relocation status of the cluster
*
*/
public Optional availabilityZoneRelocationStatus() {
return Optional.ofNullable(this.availabilityZoneRelocationStatus);
}
/**
* @return A boolean value indicating whether the resize operation is using the classic resize process. If you don't provide this parameter or set the value to false , the resize type is elastic.
*
*/
public Optional classic() {
return Optional.ofNullable(this.classic);
}
/**
* @return A unique identifier for the cluster. You use this identifier to refer to the cluster for any subsequent cluster operations such as deleting or modifying. All alphabetical characters must be lower case, no hypens at the end, no two consecutive hyphens. Cluster name should be unique for all clusters within an AWS account
*
*/
public Optional clusterIdentifier() {
return Optional.ofNullable(this.clusterIdentifier);
}
/**
* @return The Amazon Resource Name (ARN) of the cluster namespace.
*
*/
public Optional clusterNamespaceArn() {
return Optional.ofNullable(this.clusterNamespaceArn);
}
/**
* @return The name of the parameter group to be associated with this cluster.
*
*/
public Optional clusterParameterGroupName() {
return Optional.ofNullable(this.clusterParameterGroupName);
}
/**
* @return A list of security groups to be associated with this cluster.
*
*/
public List clusterSecurityGroups() {
return this.clusterSecurityGroups == null ? List.of() : this.clusterSecurityGroups;
}
/**
* @return The name of a cluster subnet group to be associated with this cluster.
*
*/
public Optional clusterSubnetGroupName() {
return Optional.ofNullable(this.clusterSubnetGroupName);
}
/**
* @return The type of the cluster. When cluster type is specified as single-node, the NumberOfNodes parameter is not required and if multi-node, the NumberOfNodes parameter is required
*
*/
public Optional clusterType() {
return Optional.ofNullable(this.clusterType);
}
/**
* @return The version of the Amazon Redshift engine software that you want to deploy on the cluster.The version selected runs on all the nodes in the cluster.
*
*/
public Optional clusterVersion() {
return Optional.ofNullable(this.clusterVersion);
}
/**
* @return The name of the first database to be created when the cluster is created. To create additional databases after the cluster is created, connect to the cluster with a SQL client and use SQL commands to create a database.
*
*/
public Optional dbName() {
return Optional.ofNullable(this.dbName);
}
/**
* @return A boolean indicating whether to enable the deferred maintenance window.
*
*/
public Optional deferMaintenance() {
return Optional.ofNullable(this.deferMaintenance);
}
/**
* @return An integer indicating the duration of the maintenance window in days. If you specify a duration, you can't specify an end time. The duration must be 45 days or less.
*
*/
public Optional deferMaintenanceDuration() {
return Optional.ofNullable(this.deferMaintenanceDuration);
}
/**
* @return A timestamp indicating end time for the deferred maintenance window. If you specify an end time, you can't specify a duration.
*
*/
public Optional deferMaintenanceEndTime() {
return Optional.ofNullable(this.deferMaintenanceEndTime);
}
/**
* @return A unique identifier for the deferred maintenance window.
*
*/
public Optional deferMaintenanceIdentifier() {
return Optional.ofNullable(this.deferMaintenanceIdentifier);
}
/**
* @return A timestamp indicating the start time for the deferred maintenance window.
*
*/
public Optional deferMaintenanceStartTime() {
return Optional.ofNullable(this.deferMaintenanceStartTime);
}
/**
* @return The destination AWS Region that you want to copy snapshots to. Constraints: Must be the name of a valid AWS Region. For more information, see Regions and Endpoints in the Amazon Web Services [https://docs.aws.amazon.com/general/latest/gr/rande.html#redshift_region] General Reference
*
*/
public Optional destinationRegion() {
return Optional.ofNullable(this.destinationRegion);
}
/**
* @return The Elastic IP (EIP) address for the cluster.
*
*/
public Optional elasticIp() {
return Optional.ofNullable(this.elasticIp);
}
/**
* @return If true, the data in the cluster is encrypted at rest.
*
*/
public Optional encrypted() {
return Optional.ofNullable(this.encrypted);
}
/**
* @return Property endpoint
*
*/
public Optional endpoint() {
return Optional.ofNullable(this.endpoint);
}
/**
* @return An option that specifies whether to create the cluster with enhanced VPC routing enabled. To create a cluster that uses enhanced VPC routing, the cluster must be in a VPC. For more information, see Enhanced VPC Routing in the Amazon Redshift Cluster Management Guide.If this option is true , enhanced VPC routing is enabled.Default: false
*
*/
public Optional enhancedVpcRouting() {
return Optional.ofNullable(this.enhancedVpcRouting);
}
/**
* @return Specifies the name of the HSM client certificate the Amazon Redshift cluster uses to retrieve the data encryption keys stored in an HSM
*
*/
public Optional hsmClientCertificateIdentifier() {
return Optional.ofNullable(this.hsmClientCertificateIdentifier);
}
/**
* @return Specifies the name of the HSM configuration that contains the information the Amazon Redshift cluster can use to retrieve and store keys in an HSM.
*
*/
public Optional hsmConfigurationIdentifier() {
return Optional.ofNullable(this.hsmConfigurationIdentifier);
}
/**
* @return A list of AWS Identity and Access Management (IAM) roles that can be used by the cluster to access other AWS services. You must supply the IAM roles in their Amazon Resource Name (ARN) format. You can supply up to 50 IAM roles in a single request
*
*/
public List iamRoles() {
return this.iamRoles == null ? List.of() : this.iamRoles;
}
/**
* @return The AWS Key Management Service (KMS) key ID of the encryption key that you want to use to encrypt data in the cluster.
*
*/
public Optional kmsKeyId() {
return Optional.ofNullable(this.kmsKeyId);
}
/**
* @return Property loggingProperties
*
*/
public Optional loggingProperties() {
return Optional.ofNullable(this.loggingProperties);
}
/**
* @return The name for the maintenance track that you want to assign for the cluster. This name change is asynchronous. The new track name stays in the PendingModifiedValues for the cluster until the next maintenance window. When the maintenance track changes, the cluster is switched to the latest cluster release available for the maintenance track. At this point, the maintenance track name is applied.
*
*/
public Optional maintenanceTrackName() {
return Optional.ofNullable(this.maintenanceTrackName);
}
/**
* @return A boolean indicating if the redshift cluster's admin user credentials is managed by Redshift or not. You can't use MasterUserPassword if ManageMasterPassword is true. If ManageMasterPassword is false or not set, Amazon Redshift uses MasterUserPassword for the admin user account's password.
*
*/
public Optional manageMasterPassword() {
return Optional.ofNullable(this.manageMasterPassword);
}
/**
* @return The number of days to retain newly copied snapshots in the destination AWS Region after they are copied from the source AWS Region. If the value is -1, the manual snapshot is retained indefinitely.The value must be either -1 or an integer between 1 and 3,653.
*
*/
public Optional manualSnapshotRetentionPeriod() {
return Optional.ofNullable(this.manualSnapshotRetentionPeriod);
}
/**
* @return The Amazon Resource Name (ARN) for the cluster's admin user credentials secret.
*
*/
public Optional masterPasswordSecretArn() {
return Optional.ofNullable(this.masterPasswordSecretArn);
}
/**
* @return The ID of the Key Management Service (KMS) key used to encrypt and store the cluster's admin user credentials secret.
*
*/
public Optional masterPasswordSecretKmsKeyId() {
return Optional.ofNullable(this.masterPasswordSecretKmsKeyId);
}
/**
* @return The password associated with the master user account for the cluster that is being created. You can't use MasterUserPassword if ManageMasterPassword is true. Password must be between 8 and 64 characters in length, should have at least one uppercase letter.Must contain at least one lowercase letter.Must contain one number.Can be any printable ASCII character.
*
*/
public Optional masterUserPassword() {
return Optional.ofNullable(this.masterUserPassword);
}
/**
* @return The user name associated with the master user account for the cluster that is being created. The user name can't be PUBLIC and first character must be a letter.
*
*/
public Optional masterUsername() {
return Optional.ofNullable(this.masterUsername);
}
/**
* @return A boolean indicating if the redshift cluster is multi-az or not. If you don't provide this parameter or set the value to false, the redshift cluster will be single-az.
*
*/
public Optional multiAZ() {
return Optional.ofNullable(this.multiAZ);
}
/**
* @return The namespace resource policy document that will be attached to a Redshift cluster.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy