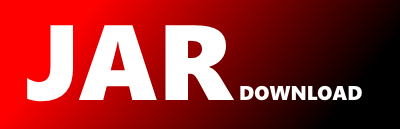
com.pulumi.azurenative.awsconnector.outputs.EbsInstanceBlockDeviceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.AttachmentStatusEnumValueResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EbsInstanceBlockDeviceResponse {
/**
* @return <p>The ARN of the Amazon ECS or Fargate task to which the volume is attached.</p>
*
*/
private @Nullable String associatedResource;
/**
* @return <p>The time stamp when the attachment initiated.</p>
*
*/
private @Nullable String attachTime;
/**
* @return <p>Indicates whether the volume is deleted on instance termination.</p>
*
*/
private @Nullable Boolean deleteOnTermination;
/**
* @return <p>The attachment state.</p>
*
*/
private @Nullable AttachmentStatusEnumValueResponse status;
/**
* @return <p>The ID of the EBS volume.</p>
*
*/
private @Nullable String volumeId;
/**
* @return <p>The ID of the Amazon Web Services account that owns the volume.</p> <p>This parameter is returned only for volumes that are attached to Fargate tasks.</p>
*
*/
private @Nullable String volumeOwnerId;
private EbsInstanceBlockDeviceResponse() {}
/**
* @return <p>The ARN of the Amazon ECS or Fargate task to which the volume is attached.</p>
*
*/
public Optional associatedResource() {
return Optional.ofNullable(this.associatedResource);
}
/**
* @return <p>The time stamp when the attachment initiated.</p>
*
*/
public Optional attachTime() {
return Optional.ofNullable(this.attachTime);
}
/**
* @return <p>Indicates whether the volume is deleted on instance termination.</p>
*
*/
public Optional deleteOnTermination() {
return Optional.ofNullable(this.deleteOnTermination);
}
/**
* @return <p>The attachment state.</p>
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return <p>The ID of the EBS volume.</p>
*
*/
public Optional volumeId() {
return Optional.ofNullable(this.volumeId);
}
/**
* @return <p>The ID of the Amazon Web Services account that owns the volume.</p> <p>This parameter is returned only for volumes that are attached to Fargate tasks.</p>
*
*/
public Optional volumeOwnerId() {
return Optional.ofNullable(this.volumeOwnerId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EbsInstanceBlockDeviceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String associatedResource;
private @Nullable String attachTime;
private @Nullable Boolean deleteOnTermination;
private @Nullable AttachmentStatusEnumValueResponse status;
private @Nullable String volumeId;
private @Nullable String volumeOwnerId;
public Builder() {}
public Builder(EbsInstanceBlockDeviceResponse defaults) {
Objects.requireNonNull(defaults);
this.associatedResource = defaults.associatedResource;
this.attachTime = defaults.attachTime;
this.deleteOnTermination = defaults.deleteOnTermination;
this.status = defaults.status;
this.volumeId = defaults.volumeId;
this.volumeOwnerId = defaults.volumeOwnerId;
}
@CustomType.Setter
public Builder associatedResource(@Nullable String associatedResource) {
this.associatedResource = associatedResource;
return this;
}
@CustomType.Setter
public Builder attachTime(@Nullable String attachTime) {
this.attachTime = attachTime;
return this;
}
@CustomType.Setter
public Builder deleteOnTermination(@Nullable Boolean deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
return this;
}
@CustomType.Setter
public Builder status(@Nullable AttachmentStatusEnumValueResponse status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder volumeId(@Nullable String volumeId) {
this.volumeId = volumeId;
return this;
}
@CustomType.Setter
public Builder volumeOwnerId(@Nullable String volumeOwnerId) {
this.volumeOwnerId = volumeOwnerId;
return this;
}
public EbsInstanceBlockDeviceResponse build() {
final var _resultValue = new EbsInstanceBlockDeviceResponse();
_resultValue.associatedResource = associatedResource;
_resultValue.attachTime = attachTime;
_resultValue.deleteOnTermination = deleteOnTermination;
_resultValue.status = status;
_resultValue.volumeId = volumeId;
_resultValue.volumeOwnerId = volumeOwnerId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy