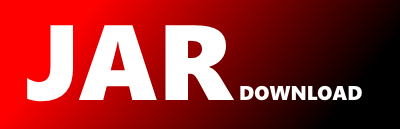
com.pulumi.azurenative.awsconnector.outputs.FirewallPolicyResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.CustomActionResponse;
import com.pulumi.azurenative.awsconnector.outputs.RuleVariablesModelResponse;
import com.pulumi.azurenative.awsconnector.outputs.StatefulEngineOptionsResponse;
import com.pulumi.azurenative.awsconnector.outputs.StatefulRuleGroupReferenceResponse;
import com.pulumi.azurenative.awsconnector.outputs.StatelessRuleGroupReferenceResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FirewallPolicyResponse {
/**
* @return Property policyVariables
*
*/
private @Nullable RuleVariablesModelResponse policyVariables;
/**
* @return Property statefulDefaultActions
*
*/
private @Nullable List statefulDefaultActions;
/**
* @return Property statefulEngineOptions
*
*/
private @Nullable StatefulEngineOptionsResponse statefulEngineOptions;
/**
* @return Property statefulRuleGroupReferences
*
*/
private @Nullable List statefulRuleGroupReferences;
/**
* @return Property statelessCustomActions
*
*/
private @Nullable List statelessCustomActions;
/**
* @return Property statelessDefaultActions
*
*/
private @Nullable List statelessDefaultActions;
/**
* @return Property statelessFragmentDefaultActions
*
*/
private @Nullable List statelessFragmentDefaultActions;
/**
* @return Property statelessRuleGroupReferences
*
*/
private @Nullable List statelessRuleGroupReferences;
/**
* @return A resource ARN.
*
*/
private @Nullable String tlsInspectionConfigurationArn;
private FirewallPolicyResponse() {}
/**
* @return Property policyVariables
*
*/
public Optional policyVariables() {
return Optional.ofNullable(this.policyVariables);
}
/**
* @return Property statefulDefaultActions
*
*/
public List statefulDefaultActions() {
return this.statefulDefaultActions == null ? List.of() : this.statefulDefaultActions;
}
/**
* @return Property statefulEngineOptions
*
*/
public Optional statefulEngineOptions() {
return Optional.ofNullable(this.statefulEngineOptions);
}
/**
* @return Property statefulRuleGroupReferences
*
*/
public List statefulRuleGroupReferences() {
return this.statefulRuleGroupReferences == null ? List.of() : this.statefulRuleGroupReferences;
}
/**
* @return Property statelessCustomActions
*
*/
public List statelessCustomActions() {
return this.statelessCustomActions == null ? List.of() : this.statelessCustomActions;
}
/**
* @return Property statelessDefaultActions
*
*/
public List statelessDefaultActions() {
return this.statelessDefaultActions == null ? List.of() : this.statelessDefaultActions;
}
/**
* @return Property statelessFragmentDefaultActions
*
*/
public List statelessFragmentDefaultActions() {
return this.statelessFragmentDefaultActions == null ? List.of() : this.statelessFragmentDefaultActions;
}
/**
* @return Property statelessRuleGroupReferences
*
*/
public List statelessRuleGroupReferences() {
return this.statelessRuleGroupReferences == null ? List.of() : this.statelessRuleGroupReferences;
}
/**
* @return A resource ARN.
*
*/
public Optional tlsInspectionConfigurationArn() {
return Optional.ofNullable(this.tlsInspectionConfigurationArn);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FirewallPolicyResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable RuleVariablesModelResponse policyVariables;
private @Nullable List statefulDefaultActions;
private @Nullable StatefulEngineOptionsResponse statefulEngineOptions;
private @Nullable List statefulRuleGroupReferences;
private @Nullable List statelessCustomActions;
private @Nullable List statelessDefaultActions;
private @Nullable List statelessFragmentDefaultActions;
private @Nullable List statelessRuleGroupReferences;
private @Nullable String tlsInspectionConfigurationArn;
public Builder() {}
public Builder(FirewallPolicyResponse defaults) {
Objects.requireNonNull(defaults);
this.policyVariables = defaults.policyVariables;
this.statefulDefaultActions = defaults.statefulDefaultActions;
this.statefulEngineOptions = defaults.statefulEngineOptions;
this.statefulRuleGroupReferences = defaults.statefulRuleGroupReferences;
this.statelessCustomActions = defaults.statelessCustomActions;
this.statelessDefaultActions = defaults.statelessDefaultActions;
this.statelessFragmentDefaultActions = defaults.statelessFragmentDefaultActions;
this.statelessRuleGroupReferences = defaults.statelessRuleGroupReferences;
this.tlsInspectionConfigurationArn = defaults.tlsInspectionConfigurationArn;
}
@CustomType.Setter
public Builder policyVariables(@Nullable RuleVariablesModelResponse policyVariables) {
this.policyVariables = policyVariables;
return this;
}
@CustomType.Setter
public Builder statefulDefaultActions(@Nullable List statefulDefaultActions) {
this.statefulDefaultActions = statefulDefaultActions;
return this;
}
public Builder statefulDefaultActions(String... statefulDefaultActions) {
return statefulDefaultActions(List.of(statefulDefaultActions));
}
@CustomType.Setter
public Builder statefulEngineOptions(@Nullable StatefulEngineOptionsResponse statefulEngineOptions) {
this.statefulEngineOptions = statefulEngineOptions;
return this;
}
@CustomType.Setter
public Builder statefulRuleGroupReferences(@Nullable List statefulRuleGroupReferences) {
this.statefulRuleGroupReferences = statefulRuleGroupReferences;
return this;
}
public Builder statefulRuleGroupReferences(StatefulRuleGroupReferenceResponse... statefulRuleGroupReferences) {
return statefulRuleGroupReferences(List.of(statefulRuleGroupReferences));
}
@CustomType.Setter
public Builder statelessCustomActions(@Nullable List statelessCustomActions) {
this.statelessCustomActions = statelessCustomActions;
return this;
}
public Builder statelessCustomActions(CustomActionResponse... statelessCustomActions) {
return statelessCustomActions(List.of(statelessCustomActions));
}
@CustomType.Setter
public Builder statelessDefaultActions(@Nullable List statelessDefaultActions) {
this.statelessDefaultActions = statelessDefaultActions;
return this;
}
public Builder statelessDefaultActions(String... statelessDefaultActions) {
return statelessDefaultActions(List.of(statelessDefaultActions));
}
@CustomType.Setter
public Builder statelessFragmentDefaultActions(@Nullable List statelessFragmentDefaultActions) {
this.statelessFragmentDefaultActions = statelessFragmentDefaultActions;
return this;
}
public Builder statelessFragmentDefaultActions(String... statelessFragmentDefaultActions) {
return statelessFragmentDefaultActions(List.of(statelessFragmentDefaultActions));
}
@CustomType.Setter
public Builder statelessRuleGroupReferences(@Nullable List statelessRuleGroupReferences) {
this.statelessRuleGroupReferences = statelessRuleGroupReferences;
return this;
}
public Builder statelessRuleGroupReferences(StatelessRuleGroupReferenceResponse... statelessRuleGroupReferences) {
return statelessRuleGroupReferences(List.of(statelessRuleGroupReferences));
}
@CustomType.Setter
public Builder tlsInspectionConfigurationArn(@Nullable String tlsInspectionConfigurationArn) {
this.tlsInspectionConfigurationArn = tlsInspectionConfigurationArn;
return this;
}
public FirewallPolicyResponse build() {
final var _resultValue = new FirewallPolicyResponse();
_resultValue.policyVariables = policyVariables;
_resultValue.statefulDefaultActions = statefulDefaultActions;
_resultValue.statefulEngineOptions = statefulEngineOptions;
_resultValue.statefulRuleGroupReferences = statefulRuleGroupReferences;
_resultValue.statelessCustomActions = statelessCustomActions;
_resultValue.statelessDefaultActions = statelessDefaultActions;
_resultValue.statelessFragmentDefaultActions = statelessFragmentDefaultActions;
_resultValue.statelessRuleGroupReferences = statelessRuleGroupReferences;
_resultValue.tlsInspectionConfigurationArn = tlsInspectionConfigurationArn;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy