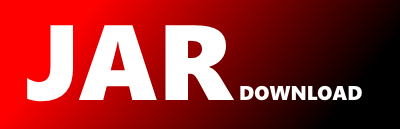
com.pulumi.azurenative.awsconnector.outputs.NodeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.DaxClusterEndpointResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class NodeResponse {
/**
* @return <p>The Availability Zone (AZ) in which the node has been deployed.</p>
*
*/
private @Nullable String availabilityZone;
/**
* @return <p>The endpoint for the node, consisting of a DNS name and a port number. Client applications can connect directly to a node endpoint, if desired (as an alternative to allowing DAX client software to intelligently route requests and responses to nodes in the DAX cluster.</p>
*
*/
private @Nullable DaxClusterEndpointResponse endpoint;
/**
* @return <p>The date and time (in UNIX epoch format) when the node was launched.</p>
*
*/
private @Nullable String nodeCreateTime;
/**
* @return <p>A system-generated identifier for the node.</p>
*
*/
private @Nullable String nodeId;
/**
* @return <p>The current status of the node. For example: <code>available</code>.</p>
*
*/
private @Nullable String nodeStatus;
/**
* @return <p>The status of the parameter group associated with this node. For example, <code>in-sync</code>.</p>
*
*/
private @Nullable String parameterGroupStatus;
private NodeResponse() {}
/**
* @return <p>The Availability Zone (AZ) in which the node has been deployed.</p>
*
*/
public Optional availabilityZone() {
return Optional.ofNullable(this.availabilityZone);
}
/**
* @return <p>The endpoint for the node, consisting of a DNS name and a port number. Client applications can connect directly to a node endpoint, if desired (as an alternative to allowing DAX client software to intelligently route requests and responses to nodes in the DAX cluster.</p>
*
*/
public Optional endpoint() {
return Optional.ofNullable(this.endpoint);
}
/**
* @return <p>The date and time (in UNIX epoch format) when the node was launched.</p>
*
*/
public Optional nodeCreateTime() {
return Optional.ofNullable(this.nodeCreateTime);
}
/**
* @return <p>A system-generated identifier for the node.</p>
*
*/
public Optional nodeId() {
return Optional.ofNullable(this.nodeId);
}
/**
* @return <p>The current status of the node. For example: <code>available</code>.</p>
*
*/
public Optional nodeStatus() {
return Optional.ofNullable(this.nodeStatus);
}
/**
* @return <p>The status of the parameter group associated with this node. For example, <code>in-sync</code>.</p>
*
*/
public Optional parameterGroupStatus() {
return Optional.ofNullable(this.parameterGroupStatus);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NodeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String availabilityZone;
private @Nullable DaxClusterEndpointResponse endpoint;
private @Nullable String nodeCreateTime;
private @Nullable String nodeId;
private @Nullable String nodeStatus;
private @Nullable String parameterGroupStatus;
public Builder() {}
public Builder(NodeResponse defaults) {
Objects.requireNonNull(defaults);
this.availabilityZone = defaults.availabilityZone;
this.endpoint = defaults.endpoint;
this.nodeCreateTime = defaults.nodeCreateTime;
this.nodeId = defaults.nodeId;
this.nodeStatus = defaults.nodeStatus;
this.parameterGroupStatus = defaults.parameterGroupStatus;
}
@CustomType.Setter
public Builder availabilityZone(@Nullable String availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
@CustomType.Setter
public Builder endpoint(@Nullable DaxClusterEndpointResponse endpoint) {
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder nodeCreateTime(@Nullable String nodeCreateTime) {
this.nodeCreateTime = nodeCreateTime;
return this;
}
@CustomType.Setter
public Builder nodeId(@Nullable String nodeId) {
this.nodeId = nodeId;
return this;
}
@CustomType.Setter
public Builder nodeStatus(@Nullable String nodeStatus) {
this.nodeStatus = nodeStatus;
return this;
}
@CustomType.Setter
public Builder parameterGroupStatus(@Nullable String parameterGroupStatus) {
this.parameterGroupStatus = parameterGroupStatus;
return this;
}
public NodeResponse build() {
final var _resultValue = new NodeResponse();
_resultValue.availabilityZone = availabilityZone;
_resultValue.endpoint = endpoint;
_resultValue.nodeCreateTime = nodeCreateTime;
_resultValue.nodeId = nodeId;
_resultValue.nodeStatus = nodeStatus;
_resultValue.parameterGroupStatus = parameterGroupStatus;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy