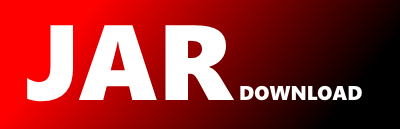
com.pulumi.azurenative.awsconnector.outputs.WebhookResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.FilterGroupResponse;
import com.pulumi.azurenative.awsconnector.outputs.WebhookBuildTypeEnumValueResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class WebhookResponse {
/**
* @return <p>A regular expression used to determine which repository branches are built when a webhook is triggered. If the name of a branch matches the regular expression, then it is built. If <code>branchFilter</code> is empty, then all branches are built.</p> <note> <p>It is recommended that you use <code>filterGroups</code> instead of <code>branchFilter</code>. </p> </note>
*
*/
private @Nullable String branchFilter;
/**
* @return <p>Specifies the type of build this webhook will trigger.</p>
*
*/
private @Nullable WebhookBuildTypeEnumValueResponse buildType;
/**
* @return <p>An array of arrays of <code>WebhookFilter</code> objects used to determine which webhooks are triggered. At least one <code>WebhookFilter</code> in the array must specify <code>EVENT</code> as its <code>type</code>. </p> <p>For a build to be triggered, at least one filter group in the <code>filterGroups</code> array must pass. For a filter group to pass, each of its filters must pass. </p>
*
*/
private @Nullable List filterGroups;
/**
* @return <p>A timestamp that indicates the last time a repository's secret token was modified. </p>
*
*/
private @Nullable String lastModifiedSecret;
/**
* @return <p>The CodeBuild endpoint where webhook events are sent.</p>
*
*/
private @Nullable String payloadUrl;
/**
* @return <p>The secret token of the associated repository. </p> <note> <p>A Bitbucket webhook does not support <code>secret</code>. </p> </note>
*
*/
private @Nullable String secret;
/**
* @return <p>The URL to the webhook.</p>
*
*/
private @Nullable String url;
private WebhookResponse() {}
/**
* @return <p>A regular expression used to determine which repository branches are built when a webhook is triggered. If the name of a branch matches the regular expression, then it is built. If <code>branchFilter</code> is empty, then all branches are built.</p> <note> <p>It is recommended that you use <code>filterGroups</code> instead of <code>branchFilter</code>. </p> </note>
*
*/
public Optional branchFilter() {
return Optional.ofNullable(this.branchFilter);
}
/**
* @return <p>Specifies the type of build this webhook will trigger.</p>
*
*/
public Optional buildType() {
return Optional.ofNullable(this.buildType);
}
/**
* @return <p>An array of arrays of <code>WebhookFilter</code> objects used to determine which webhooks are triggered. At least one <code>WebhookFilter</code> in the array must specify <code>EVENT</code> as its <code>type</code>. </p> <p>For a build to be triggered, at least one filter group in the <code>filterGroups</code> array must pass. For a filter group to pass, each of its filters must pass. </p>
*
*/
public List filterGroups() {
return this.filterGroups == null ? List.of() : this.filterGroups;
}
/**
* @return <p>A timestamp that indicates the last time a repository's secret token was modified. </p>
*
*/
public Optional lastModifiedSecret() {
return Optional.ofNullable(this.lastModifiedSecret);
}
/**
* @return <p>The CodeBuild endpoint where webhook events are sent.</p>
*
*/
public Optional payloadUrl() {
return Optional.ofNullable(this.payloadUrl);
}
/**
* @return <p>The secret token of the associated repository. </p> <note> <p>A Bitbucket webhook does not support <code>secret</code>. </p> </note>
*
*/
public Optional secret() {
return Optional.ofNullable(this.secret);
}
/**
* @return <p>The URL to the webhook.</p>
*
*/
public Optional url() {
return Optional.ofNullable(this.url);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(WebhookResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String branchFilter;
private @Nullable WebhookBuildTypeEnumValueResponse buildType;
private @Nullable List filterGroups;
private @Nullable String lastModifiedSecret;
private @Nullable String payloadUrl;
private @Nullable String secret;
private @Nullable String url;
public Builder() {}
public Builder(WebhookResponse defaults) {
Objects.requireNonNull(defaults);
this.branchFilter = defaults.branchFilter;
this.buildType = defaults.buildType;
this.filterGroups = defaults.filterGroups;
this.lastModifiedSecret = defaults.lastModifiedSecret;
this.payloadUrl = defaults.payloadUrl;
this.secret = defaults.secret;
this.url = defaults.url;
}
@CustomType.Setter
public Builder branchFilter(@Nullable String branchFilter) {
this.branchFilter = branchFilter;
return this;
}
@CustomType.Setter
public Builder buildType(@Nullable WebhookBuildTypeEnumValueResponse buildType) {
this.buildType = buildType;
return this;
}
@CustomType.Setter
public Builder filterGroups(@Nullable List filterGroups) {
this.filterGroups = filterGroups;
return this;
}
public Builder filterGroups(FilterGroupResponse... filterGroups) {
return filterGroups(List.of(filterGroups));
}
@CustomType.Setter
public Builder lastModifiedSecret(@Nullable String lastModifiedSecret) {
this.lastModifiedSecret = lastModifiedSecret;
return this;
}
@CustomType.Setter
public Builder payloadUrl(@Nullable String payloadUrl) {
this.payloadUrl = payloadUrl;
return this;
}
@CustomType.Setter
public Builder secret(@Nullable String secret) {
this.secret = secret;
return this;
}
@CustomType.Setter
public Builder url(@Nullable String url) {
this.url = url;
return this;
}
public WebhookResponse build() {
final var _resultValue = new WebhookResponse();
_resultValue.branchFilter = branchFilter;
_resultValue.buildType = buildType;
_resultValue.filterGroups = filterGroups;
_resultValue.lastModifiedSecret = lastModifiedSecret;
_resultValue.payloadUrl = payloadUrl;
_resultValue.secret = secret;
_resultValue.url = url;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy