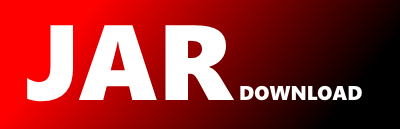
com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetNetworkConfigurationPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurefleet.outputs;
import com.pulumi.azurenative.azurefleet.outputs.SubResourceResponse;
import com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetIPConfigurationResponse;
import com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetNetworkConfigurationDnsSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualMachineScaleSetNetworkConfigurationPropertiesResponse {
/**
* @return Specifies whether the Auxiliary mode is enabled for the Network Interface
* resource.
*
*/
private @Nullable String auxiliaryMode;
/**
* @return Specifies whether the Auxiliary sku is enabled for the Network Interface
* resource.
*
*/
private @Nullable String auxiliarySku;
/**
* @return Specify what happens to the network interface when the VM is deleted
*
*/
private @Nullable String deleteOption;
/**
* @return Specifies whether the network interface is disabled for tcp state tracking.
*
*/
private @Nullable Boolean disableTcpStateTracking;
/**
* @return The dns settings to be applied on the network interfaces.
*
*/
private @Nullable VirtualMachineScaleSetNetworkConfigurationDnsSettingsResponse dnsSettings;
/**
* @return Specifies whether the network interface is accelerated networking-enabled.
*
*/
private @Nullable Boolean enableAcceleratedNetworking;
/**
* @return Specifies whether the network interface is FPGA networking-enabled.
*
*/
private @Nullable Boolean enableFpga;
/**
* @return Whether IP forwarding enabled on this NIC.
*
*/
private @Nullable Boolean enableIPForwarding;
/**
* @return Specifies the IP configurations of the network interface.
*
*/
private List ipConfigurations;
/**
* @return The network security group.
*
*/
private @Nullable SubResourceResponse networkSecurityGroup;
/**
* @return Specifies the primary network interface in case the virtual machine has more
* than 1 network interface.
*
*/
private @Nullable Boolean primary;
private VirtualMachineScaleSetNetworkConfigurationPropertiesResponse() {}
/**
* @return Specifies whether the Auxiliary mode is enabled for the Network Interface
* resource.
*
*/
public Optional auxiliaryMode() {
return Optional.ofNullable(this.auxiliaryMode);
}
/**
* @return Specifies whether the Auxiliary sku is enabled for the Network Interface
* resource.
*
*/
public Optional auxiliarySku() {
return Optional.ofNullable(this.auxiliarySku);
}
/**
* @return Specify what happens to the network interface when the VM is deleted
*
*/
public Optional deleteOption() {
return Optional.ofNullable(this.deleteOption);
}
/**
* @return Specifies whether the network interface is disabled for tcp state tracking.
*
*/
public Optional disableTcpStateTracking() {
return Optional.ofNullable(this.disableTcpStateTracking);
}
/**
* @return The dns settings to be applied on the network interfaces.
*
*/
public Optional dnsSettings() {
return Optional.ofNullable(this.dnsSettings);
}
/**
* @return Specifies whether the network interface is accelerated networking-enabled.
*
*/
public Optional enableAcceleratedNetworking() {
return Optional.ofNullable(this.enableAcceleratedNetworking);
}
/**
* @return Specifies whether the network interface is FPGA networking-enabled.
*
*/
public Optional enableFpga() {
return Optional.ofNullable(this.enableFpga);
}
/**
* @return Whether IP forwarding enabled on this NIC.
*
*/
public Optional enableIPForwarding() {
return Optional.ofNullable(this.enableIPForwarding);
}
/**
* @return Specifies the IP configurations of the network interface.
*
*/
public List ipConfigurations() {
return this.ipConfigurations;
}
/**
* @return The network security group.
*
*/
public Optional networkSecurityGroup() {
return Optional.ofNullable(this.networkSecurityGroup);
}
/**
* @return Specifies the primary network interface in case the virtual machine has more
* than 1 network interface.
*
*/
public Optional primary() {
return Optional.ofNullable(this.primary);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VirtualMachineScaleSetNetworkConfigurationPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String auxiliaryMode;
private @Nullable String auxiliarySku;
private @Nullable String deleteOption;
private @Nullable Boolean disableTcpStateTracking;
private @Nullable VirtualMachineScaleSetNetworkConfigurationDnsSettingsResponse dnsSettings;
private @Nullable Boolean enableAcceleratedNetworking;
private @Nullable Boolean enableFpga;
private @Nullable Boolean enableIPForwarding;
private List ipConfigurations;
private @Nullable SubResourceResponse networkSecurityGroup;
private @Nullable Boolean primary;
public Builder() {}
public Builder(VirtualMachineScaleSetNetworkConfigurationPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.auxiliaryMode = defaults.auxiliaryMode;
this.auxiliarySku = defaults.auxiliarySku;
this.deleteOption = defaults.deleteOption;
this.disableTcpStateTracking = defaults.disableTcpStateTracking;
this.dnsSettings = defaults.dnsSettings;
this.enableAcceleratedNetworking = defaults.enableAcceleratedNetworking;
this.enableFpga = defaults.enableFpga;
this.enableIPForwarding = defaults.enableIPForwarding;
this.ipConfigurations = defaults.ipConfigurations;
this.networkSecurityGroup = defaults.networkSecurityGroup;
this.primary = defaults.primary;
}
@CustomType.Setter
public Builder auxiliaryMode(@Nullable String auxiliaryMode) {
this.auxiliaryMode = auxiliaryMode;
return this;
}
@CustomType.Setter
public Builder auxiliarySku(@Nullable String auxiliarySku) {
this.auxiliarySku = auxiliarySku;
return this;
}
@CustomType.Setter
public Builder deleteOption(@Nullable String deleteOption) {
this.deleteOption = deleteOption;
return this;
}
@CustomType.Setter
public Builder disableTcpStateTracking(@Nullable Boolean disableTcpStateTracking) {
this.disableTcpStateTracking = disableTcpStateTracking;
return this;
}
@CustomType.Setter
public Builder dnsSettings(@Nullable VirtualMachineScaleSetNetworkConfigurationDnsSettingsResponse dnsSettings) {
this.dnsSettings = dnsSettings;
return this;
}
@CustomType.Setter
public Builder enableAcceleratedNetworking(@Nullable Boolean enableAcceleratedNetworking) {
this.enableAcceleratedNetworking = enableAcceleratedNetworking;
return this;
}
@CustomType.Setter
public Builder enableFpga(@Nullable Boolean enableFpga) {
this.enableFpga = enableFpga;
return this;
}
@CustomType.Setter
public Builder enableIPForwarding(@Nullable Boolean enableIPForwarding) {
this.enableIPForwarding = enableIPForwarding;
return this;
}
@CustomType.Setter
public Builder ipConfigurations(List ipConfigurations) {
if (ipConfigurations == null) {
throw new MissingRequiredPropertyException("VirtualMachineScaleSetNetworkConfigurationPropertiesResponse", "ipConfigurations");
}
this.ipConfigurations = ipConfigurations;
return this;
}
public Builder ipConfigurations(VirtualMachineScaleSetIPConfigurationResponse... ipConfigurations) {
return ipConfigurations(List.of(ipConfigurations));
}
@CustomType.Setter
public Builder networkSecurityGroup(@Nullable SubResourceResponse networkSecurityGroup) {
this.networkSecurityGroup = networkSecurityGroup;
return this;
}
@CustomType.Setter
public Builder primary(@Nullable Boolean primary) {
this.primary = primary;
return this;
}
public VirtualMachineScaleSetNetworkConfigurationPropertiesResponse build() {
final var _resultValue = new VirtualMachineScaleSetNetworkConfigurationPropertiesResponse();
_resultValue.auxiliaryMode = auxiliaryMode;
_resultValue.auxiliarySku = auxiliarySku;
_resultValue.deleteOption = deleteOption;
_resultValue.disableTcpStateTracking = disableTcpStateTracking;
_resultValue.dnsSettings = dnsSettings;
_resultValue.enableAcceleratedNetworking = enableAcceleratedNetworking;
_resultValue.enableFpga = enableFpga;
_resultValue.enableIPForwarding = enableIPForwarding;
_resultValue.ipConfigurations = ipConfigurations;
_resultValue.networkSecurityGroup = networkSecurityGroup;
_resultValue.primary = primary;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy