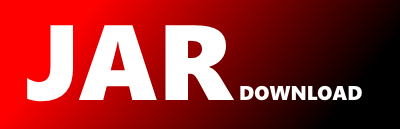
com.pulumi.azurenative.azuresphere.AzuresphereFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azuresphere;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.azuresphere.inputs.GetCatalogArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetCatalogPlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetDeploymentArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetDeploymentPlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetDeviceArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetDeviceGroupArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetDeviceGroupPlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetDevicePlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetImageArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetImagePlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetProductArgs;
import com.pulumi.azurenative.azuresphere.inputs.GetProductPlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDeploymentsArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDeploymentsPlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDeviceGroupsArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDeviceGroupsPlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDeviceInsightsArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDeviceInsightsPlainArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDevicesArgs;
import com.pulumi.azurenative.azuresphere.inputs.ListCatalogDevicesPlainArgs;
import com.pulumi.azurenative.azuresphere.outputs.GetCatalogResult;
import com.pulumi.azurenative.azuresphere.outputs.GetDeploymentResult;
import com.pulumi.azurenative.azuresphere.outputs.GetDeviceGroupResult;
import com.pulumi.azurenative.azuresphere.outputs.GetDeviceResult;
import com.pulumi.azurenative.azuresphere.outputs.GetImageResult;
import com.pulumi.azurenative.azuresphere.outputs.GetProductResult;
import com.pulumi.azurenative.azuresphere.outputs.ListCatalogDeploymentsResult;
import com.pulumi.azurenative.azuresphere.outputs.ListCatalogDeviceGroupsResult;
import com.pulumi.azurenative.azuresphere.outputs.ListCatalogDeviceInsightsResult;
import com.pulumi.azurenative.azuresphere.outputs.ListCatalogDevicesResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class AzuresphereFunctions {
/**
* Get a Catalog
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getCatalog(GetCatalogArgs args) {
return getCatalog(args, InvokeOptions.Empty);
}
/**
* Get a Catalog
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getCatalogPlain(GetCatalogPlainArgs args) {
return getCatalogPlain(args, InvokeOptions.Empty);
}
/**
* Get a Catalog
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getCatalog(GetCatalogArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:getCatalog", TypeShape.of(GetCatalogResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Catalog
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getCatalogPlain(GetCatalogPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:getCatalog", TypeShape.of(GetCatalogResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Deployment. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getDeployment(GetDeploymentArgs args) {
return getDeployment(args, InvokeOptions.Empty);
}
/**
* Get a Deployment. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getDeploymentPlain(GetDeploymentPlainArgs args) {
return getDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Get a Deployment. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getDeployment(GetDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:getDeployment", TypeShape.of(GetDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Deployment. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getDeploymentPlain(GetDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:getDeployment", TypeShape.of(GetDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Device. Use '.unassigned' or '.default' for the device group and product names when a device does not belong to a device group and product.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getDevice(GetDeviceArgs args) {
return getDevice(args, InvokeOptions.Empty);
}
/**
* Get a Device. Use '.unassigned' or '.default' for the device group and product names when a device does not belong to a device group and product.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getDevicePlain(GetDevicePlainArgs args) {
return getDevicePlain(args, InvokeOptions.Empty);
}
/**
* Get a Device. Use '.unassigned' or '.default' for the device group and product names when a device does not belong to a device group and product.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getDevice(GetDeviceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:getDevice", TypeShape.of(GetDeviceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Device. Use '.unassigned' or '.default' for the device group and product names when a device does not belong to a device group and product.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getDevicePlain(GetDevicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:getDevice", TypeShape.of(GetDeviceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DeviceGroup. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getDeviceGroup(GetDeviceGroupArgs args) {
return getDeviceGroup(args, InvokeOptions.Empty);
}
/**
* Get a DeviceGroup. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getDeviceGroupPlain(GetDeviceGroupPlainArgs args) {
return getDeviceGroupPlain(args, InvokeOptions.Empty);
}
/**
* Get a DeviceGroup. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getDeviceGroup(GetDeviceGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:getDeviceGroup", TypeShape.of(GetDeviceGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DeviceGroup. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getDeviceGroupPlain(GetDeviceGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:getDeviceGroup", TypeShape.of(GetDeviceGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Image
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getImage(GetImageArgs args) {
return getImage(args, InvokeOptions.Empty);
}
/**
* Get a Image
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getImagePlain(GetImagePlainArgs args) {
return getImagePlain(args, InvokeOptions.Empty);
}
/**
* Get a Image
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getImage(GetImageArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:getImage", TypeShape.of(GetImageResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Image
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getImagePlain(GetImagePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:getImage", TypeShape.of(GetImageResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Product. '.default' and '.unassigned' are system defined values and cannot be used for product name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getProduct(GetProductArgs args) {
return getProduct(args, InvokeOptions.Empty);
}
/**
* Get a Product. '.default' and '.unassigned' are system defined values and cannot be used for product name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getProductPlain(GetProductPlainArgs args) {
return getProductPlain(args, InvokeOptions.Empty);
}
/**
* Get a Product. '.default' and '.unassigned' are system defined values and cannot be used for product name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output getProduct(GetProductArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:getProduct", TypeShape.of(GetProductResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Product. '.default' and '.unassigned' are system defined values and cannot be used for product name.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture getProductPlain(GetProductPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:getProduct", TypeShape.of(GetProductResult.class), args, Utilities.withVersion(options));
}
/**
* Lists deployments for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDeployments(ListCatalogDeploymentsArgs args) {
return listCatalogDeployments(args, InvokeOptions.Empty);
}
/**
* Lists deployments for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDeploymentsPlain(ListCatalogDeploymentsPlainArgs args) {
return listCatalogDeploymentsPlain(args, InvokeOptions.Empty);
}
/**
* Lists deployments for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDeployments(ListCatalogDeploymentsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:listCatalogDeployments", TypeShape.of(ListCatalogDeploymentsResult.class), args, Utilities.withVersion(options));
}
/**
* Lists deployments for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDeploymentsPlain(ListCatalogDeploymentsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:listCatalogDeployments", TypeShape.of(ListCatalogDeploymentsResult.class), args, Utilities.withVersion(options));
}
/**
* List the device groups for the catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDeviceGroups(ListCatalogDeviceGroupsArgs args) {
return listCatalogDeviceGroups(args, InvokeOptions.Empty);
}
/**
* List the device groups for the catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDeviceGroupsPlain(ListCatalogDeviceGroupsPlainArgs args) {
return listCatalogDeviceGroupsPlain(args, InvokeOptions.Empty);
}
/**
* List the device groups for the catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDeviceGroups(ListCatalogDeviceGroupsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:listCatalogDeviceGroups", TypeShape.of(ListCatalogDeviceGroupsResult.class), args, Utilities.withVersion(options));
}
/**
* List the device groups for the catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDeviceGroupsPlain(ListCatalogDeviceGroupsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:listCatalogDeviceGroups", TypeShape.of(ListCatalogDeviceGroupsResult.class), args, Utilities.withVersion(options));
}
/**
* Lists device insights for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDeviceInsights(ListCatalogDeviceInsightsArgs args) {
return listCatalogDeviceInsights(args, InvokeOptions.Empty);
}
/**
* Lists device insights for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDeviceInsightsPlain(ListCatalogDeviceInsightsPlainArgs args) {
return listCatalogDeviceInsightsPlain(args, InvokeOptions.Empty);
}
/**
* Lists device insights for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDeviceInsights(ListCatalogDeviceInsightsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:listCatalogDeviceInsights", TypeShape.of(ListCatalogDeviceInsightsResult.class), args, Utilities.withVersion(options));
}
/**
* Lists device insights for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDeviceInsightsPlain(ListCatalogDeviceInsightsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:listCatalogDeviceInsights", TypeShape.of(ListCatalogDeviceInsightsResult.class), args, Utilities.withVersion(options));
}
/**
* Lists devices for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDevices(ListCatalogDevicesArgs args) {
return listCatalogDevices(args, InvokeOptions.Empty);
}
/**
* Lists devices for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDevicesPlain(ListCatalogDevicesPlainArgs args) {
return listCatalogDevicesPlain(args, InvokeOptions.Empty);
}
/**
* Lists devices for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static Output listCatalogDevices(ListCatalogDevicesArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:azuresphere:listCatalogDevices", TypeShape.of(ListCatalogDevicesResult.class), args, Utilities.withVersion(options));
}
/**
* Lists devices for catalog.
* Azure REST API version: 2022-09-01-preview.
*
* Other available API versions: 2024-04-01.
*
*/
public static CompletableFuture listCatalogDevicesPlain(ListCatalogDevicesPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:azuresphere:listCatalogDevices", TypeShape.of(ListCatalogDevicesResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy