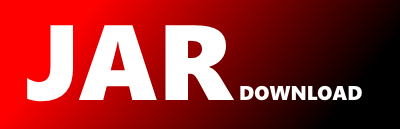
com.pulumi.azurenative.azurestackhci.inputs.DeploymentDataArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci.inputs;
import com.pulumi.azurenative.azurestackhci.inputs.ClusterArgs;
import com.pulumi.azurenative.azurestackhci.inputs.HostNetworkArgs;
import com.pulumi.azurenative.azurestackhci.inputs.InfrastructureNetworkArgs;
import com.pulumi.azurenative.azurestackhci.inputs.ObservabilityArgs;
import com.pulumi.azurenative.azurestackhci.inputs.OptionalServicesArgs;
import com.pulumi.azurenative.azurestackhci.inputs.PhysicalNodesArgs;
import com.pulumi.azurenative.azurestackhci.inputs.SecuritySettingsArgs;
import com.pulumi.azurenative.azurestackhci.inputs.StorageArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The Deployment data of AzureStackHCI Cluster.
*
*/
public final class DeploymentDataArgs extends com.pulumi.resources.ResourceArgs {
public static final DeploymentDataArgs Empty = new DeploymentDataArgs();
/**
* The path to the Active Directory Organizational Unit container object prepared for the deployment.
*
*/
@Import(name="adouPath")
private @Nullable Output adouPath;
/**
* @return The path to the Active Directory Organizational Unit container object prepared for the deployment.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy